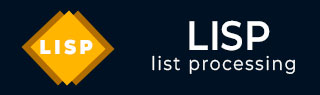
- LISP 教程
- LISP - 首页
- LISP - 概述
- LISP - 环境
- LISP - 程序结构
- LISP - 基本语法
- LISP - 数据类型
- LISP - 宏
- LISP - 变量
- LISP - 常量
- LISP - 运算符
- LISP - 决策
- LISP - 循环
- LISP - 函数
- LISP - 谓词
- LISP - 数字
- LISP - 字符
- LISP - 数组
- LISP - 字符串
- LISP - 序列
- LISP - 列表
- LISP - 符号
- LISP - 向量
- LISP - 集合
- LISP - 树
- LISP - 哈希表
- LISP - 输入 & 输出
- LISP - 文件 I/O
- LISP - 结构体
- LISP - 包
- LISP - 错误处理
- LISP - CLOS
- LISP 有用资源
- Lisp - 快速指南
- Lisp - 有用资源
- Lisp - 讨论
Lisp - 位运算符
位运算符作用于位,并执行逐位运算。位与、位或和位异或运算的真值表如下所示:
p | q | p 与 q | p 或 q | p 异或 q |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Assume if A = 60; and B = 13; now in binary format they will be as follows: A = 0011 1100 B = 0000 1101 ----------------- A and B = 0000 1100 A or B = 0011 1101 A xor B = 0011 0001 not A = 1100 0011
LISP 支持的位运算符列在下表中。假设变量 A 的值为 60,变量 B 的值为 13,则:
运算符 | 描述 | 示例 |
---|---|---|
logand | 返回其参数的按位逻辑与。如果未给出参数,则结果为 -1,这是此运算的恒等式。 | (logand a b) 将给出 12 |
logior | 返回其参数的按位逻辑包含或。如果未给出参数,则结果为零,这是此运算的恒等式。 | (logior a b) 将给出 61 |
logxor | 返回其参数的按位逻辑异或。如果未给出参数,则结果为零,这是此运算的恒等式。 | (logxor a b) 将给出 49 |
lognor | 返回其参数的按位非。如果未给出参数,则结果为 -1,这是此运算的恒等式。 | (lognor a b) 将给出 -62, |
logeqv | 返回其参数的按位逻辑等价(也称为异或非)。如果未给出参数,则结果为 -1,这是此运算的恒等式。 | (logeqv a b) 将给出 -50 |
示例 - logand、logior 运算符
创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。这里我们使用logand、logior运算符来检查各种场景。
main.lisp
; set a as 60 (setq a 60) ; set b as 13 (setq b 13) ; print logand of a and b (format t "~% BITWISE AND of a and b is ~a" (logand a b)) ; print logand of a or b (format t "~% BITWISE INCLUSIVE OR of a and b is ~a" (logior a b)) ; terminate printing to print in new line (terpri) ; assign variables new values (setq a 10) (setq b 0) (setq c 30) (setq d 40) ; print logand of all four variables (format t "~% Result of bitwise and operation on 10, 0, 30, 40 is ~a" (logand a b c d)) ; print logor of all four variables (format t "~% Result of bitwise or operation on 10, 0, 30, 40 is ~a" (logior a b c d))
单击“执行”按钮或键入 Ctrl+E 时,LISP 会立即执行它,并返回以下结果:
BITWISE AND of a and b is 12 BITWISE INCLUSIVE OR of a and b is 61 Result of bitwise and operation on 10, 0, 30, 40 is 0 Result of bitwise or operation on 10, 0, 30, 40 is 62
示例 - logxor、lognor 运算符
更新名为 main.lisp 的源代码文件,并在其中键入以下代码。这里我们使用logxor、lognor运算符来检查各种场景。
main.lisp
; set a as 60 (setq a 60) ; set b as 13 (setq b 13) ; print logxor of a and b (format t "~% BITWISE EXCLUSIVE OR of a and b is ~a" (logxor a b)) ; print lognor of a and b (format t "~% A NOT B is ~a" (lognor a b)) ; terminate printing to print in new line (terpri) ; assign variables new values (setq a 10) (setq b 0) (setq c 30) (setq d 40) ; print logxor of all four variables (format t "~% Result of bitwise xor operation on 10, 0, 30, 40 is ~a" (logxor a b c d)) ; print logeqv of all four variables (format t "~% Result of bitwise eqivalance operation on 10, 0, 30, 40 is ~a" (logeqv a b c d))
单击“执行”按钮或键入 Ctrl+E 时,LISP 会立即执行它,并返回以下结果:
BITWISE EXCLUSIVE OR of a and b is 49A NOT B is -62 Result of bitwise xor operation on 10, 0, 30, 40 is 60 Result of bitwise eqivalance operation on 10, 0, 30, 40 is -61
示例 - logeqv 运算符
更新名为 main.lisp 的源代码文件,并在其中键入以下代码。这里我们使用logeqv运算符来检查场景。
main.lisp
; set a as 60 (setq a 60) ; set b as 13 (setq b 13) ; print logeqv of a and b (format t "~% A EQUIVALANCE B is ~a" (logeqv a b)) ; terminate printing to print in new line (terpri) ; assign variables new values (setq a 10) (setq b 0) (setq c 30) (setq d 40) ; print logeqv of all four variables (format t "~% Result of bitwise eqivalance operation on 10, 0, 30, 40 is ~a" (logeqv a b c d))
单击“执行”按钮或键入 Ctrl+E 时,LISP 会立即执行它,并返回以下结果:
A EQUIVALANCE B is -50 Result of bitwise eqivalance operation on 10, 0, 30, 40 is -61
lisp_operators.htm
广告