PDFBox - 添加文本
在上一章中,我们讨论了如何向 PDF 文档添加页面。在本章中,我们将讨论如何向现有的 PDF 文档添加文本。
向现有 PDF 文档添加文本
您可以使用 PDFBox 库向文档添加内容,它提供了一个名为 PDPageContentStream 的类,其中包含插入文本、图像和其他类型内容到 PDF 文档页面所需的必要方法。
以下是创建空文档并在其中向页面添加内容的步骤。
步骤 1:加载现有文档
您可以使用 PDDocument 类的 load() 方法加载现有文档。因此,实例化此类并加载所需的文档,如下所示。
File file = new File("Path of the document"); PDDocument doc = document.load(file);
步骤 2:获取所需的页面
您可以使用 getPage() 方法获取文档中的所需页面。通过将页面的索引传递给此方法,检索所需页面的对象,如下所示。
PDPage page = doc.getPage(1);
步骤 3:准备内容流
您可以使用 PDPageContentStream 类的对象插入各种类型的数据元素。您需要将文档对象和页面对象传递给此类的构造函数,因此,通过传递在前面步骤中创建的这两个对象来实例化此类,如下所示。
PDPageContentStream contentStream = new PDPageContentStream(doc, page);
步骤 4:开始文本
在 PDF 文档中插入文本时,您可以使用 PDPageContentStream 类的 beginText() 和 endText() 方法指定文本的起始和结束点,如下所示。
contentStream.beginText(); ……………………….. code to add text content ……………………….. contentStream.endText();
因此,使用 beginText() 方法开始文本,如下所示。
contentStream.beginText();
步骤 5:设置文本的位置
使用 newLineAtOffset() 方法,您可以在页面上的内容流中设置位置。
//Setting the position for the line contentStream.newLineAtOffset(25, 700);
步骤 6:设置字体
您可以使用 PDPageContentStream 类的 setFont() 方法将文本的字体设置为所需的样式,如下所示。您需要将字体类型和大小传递给此方法。
contentStream.setFont( font_type, font_size );
步骤 7:插入文本
您可以使用 PDPageContentStream 类的 ShowText() 方法将文本插入页面,如下所示。此方法接受以字符串形式表示的所需文本。
contentStream.showText(text);
步骤 8:结束文本
插入文本后,您需要使用 PDPageContentStream 类的 endText() 方法结束文本,如下所示。
contentStream.endText();
步骤 9:关闭 PDPageContentStream
使用 close() 方法关闭 PDPageContentStream 对象,如下所示。
contentstream.close();
步骤 10:保存文档
添加所需内容后,使用 PDDocument 类的 save() 方法保存 PDF 文档,如下面的代码块所示。
doc.save("Path");
步骤 11:关闭文档
最后,使用 PDDocument 类的 close() 方法关闭文档,如下所示。
doc.close();
示例
此示例演示了如何在文档的页面中添加内容。在这里,我们将创建一个 Java 程序来加载名为 my_doc.pdf 的 PDF 文档(保存在 C:/PdfBox_Examples/ 路径下),并向其中添加一些文本。将此代码保存在名为 AddingContent.java 的文件中。
import java.io.File; import java.io.IOException; import org.apache.pdfbox.pdmodel.PDDocument; import org.apache.pdfbox.pdmodel.PDPage; import org.apache.pdfbox.pdmodel.PDPageContentStream; import org.apache.pdfbox.pdmodel.font.PDType1Font; public class AddingContent { public static void main (String args[]) throws IOException { //Loading an existing document File file = new File("C:/PdfBox_Examples/my_doc.pdf"); PDDocument document = PDDocument.load(file); //Retrieving the pages of the document PDPage page = document.getPage(1); PDPageContentStream contentStream = new PDPageContentStream(document, page); //Begin the Content stream contentStream.beginText(); //Setting the font to the Content stream contentStream.setFont(PDType1Font.TIMES_ROMAN, 12); //Setting the position for the line contentStream.newLineAtOffset(25, 500); String text = "This is the sample document and we are adding content to it."; //Adding text in the form of string contentStream.showText(text); //Ending the content stream contentStream.endText(); System.out.println("Content added"); //Closing the content stream contentStream.close(); //Saving the document document.save(new File("C:/PdfBox_Examples/new.pdf")); //Closing the document document.close(); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac AddingContent.java java AddingContent
执行后,上述程序将给定的文本添加到文档中,并显示以下消息。
Content added
如果在指定的路径中验证 PDF 文档 new.pdf,您可以观察到给定的内容已添加到文档中,如下所示。
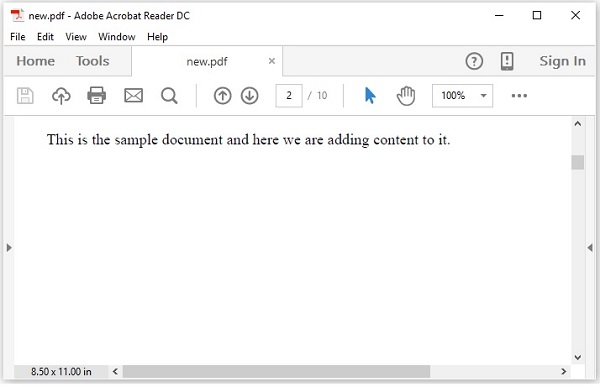