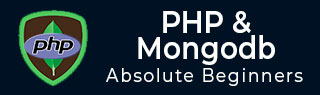
- PHP & MongoDB 教程
- PHP & MongoDB - 首页
- PHP & MongoDB - 概述
- PHP & MongoDB - 环境设置
- PHP & MongoDB 示例
- PHP & MongoDB - 连接数据库
- PHP & MongoDB - 显示数据库
- PHP & MongoDB - 删除数据库
- PHP & MongoDB - 创建集合
- PHP & MongoDB - 删除集合
- PHP & MongoDB - 显示集合
- PHP & MongoDB - 插入文档
- PHP & MongoDB - 选择文档
- PHP & MongoDB - 更新文档
- PHP & MongoDB - 删除文档
- PHP & MongoDB - 嵌入式文档
- PHP & MongoDB - 错误处理
- PHP & MongoDB - 限制记录
- PHP & MongoDB - 排序记录
- PHP & MongoDB 有用资源
- PHP & MongoDB - 快速指南
- PHP & MongoDB - 有用资源
- PHP & MongoDB - 讨论
PHP & MongoDB - 快速指南
PHP & MongoDB - 概述
PHP 开发团队提供了 MongoDB PHP 驱动程序,并提供了各种相关资源。
使用 PHP 连接 MongoDB 的第一步是在 php ext 目录中拥有 mongodb PHP 驱动程序 dll,并在 php.ini 中启用它,然后使用 mongodb API 连接到数据库。
连接到 MongoDB 数据库
假设 MongoDB 安装在本地并使用默认端口,则以下语法连接到 MongoDB 数据库。
$manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
MongoDB 驱动程序管理器是执行所有 mongodb 数据库操作的中心类。要连接到 mongodb 数据库,我们首先准备一个命令,然后执行它来连接到数据库。
$statistics = new MongoDB\Driver\Command(["dbstats" => 1]); $cursor = $manager->executeCommand("mydb", $statistics);
命令执行后,如果 mydb 数据库不存在,则会创建它,否则会连接到它。
PHP & MongoDB - 环境设置
安装 MongoDB 数据库
按照使用 MongoDB - 环境设置 的 MongoDB 安装步骤进行操作。
安装 PHP
按照使用 PHP 7 - 环境设置 的 PHP 安装步骤进行操作。
PHP MongoDB 驱动程序
要将 MongoDB 与 PHP 一起使用,您需要使用 MongoDB PHP 驱动程序。从网址 下载 PHP 驱动程序 下载驱动程序。确保下载其最新版本。现在解压缩存档并将 php_mongo.dll 放入您的 PHP 扩展目录(默认情况下为“ext”),并将以下行添加到您的 php.ini 文件中:
extension = php_mongo.dll
在 Windows 中,请确保 libsasl.dll 位于 windows 的 PATH 中。此 dll 可在 PHP 安装目录中找到。
PHP & MongoDB - 连接数据库
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并在数据库上执行一个命令,如果数据库不存在,则 MongoDB 会自动创建它。
// Create a Command Instance $statistics = new MongoDB\Driver\Command(["dbstats" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $statistics);
示例
尝试以下示例以连接到 MongoDB 服务器:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); echo "Connection to database successfully"; $statistics = new MongoDB\Driver\Command(["dbstats" => 1]); $cursor = $manager->executeCommand("myDb", $statistics); $statistics = current($cursor->toArray()); echo "<pre>"; print_r($statistics); echo "</pre>"; } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Connection to database successfully stdClass Object ( [db] => myDb [collections] => 0 [views] => 0 [objects] => 0 [avgObjSize] => 0 [dataSize] => 0 [storageSize] => 0 [totalSize] => 0 [indexes] => 0 [indexSize] => 0 [scaleFactor] => 1 [fileSize] => 0 [fsUsedSize] => 0 [fsTotalSize] => 0 [ok] => 1 )
PHP & MongoDB - 显示数据库
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并在数据库上执行一个命令来显示可用的数据库列表。
// Create a Command Instance $databaseList = new MongoDB\Driver\Command(["listDatabases" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("admin", $databaseList);
示例
尝试以下示例以列出 MongoDB 服务器中默认可用的数据库:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); // Create a Command Instance $databaseList = new MongoDB\Driver\Command(["listDatabases" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("admin", $databaseList); $databases = current($cursor->toArray()); foreach ($databases->databases as $database) { echo $database->name . "<br/>"; } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
admin config local
PHP & MongoDB - 删除数据库
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个命令来删除数据库。
// Create a Command Instance $dropDatabase = new MongoDB\Driver\Command(["dropDatabase" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $dropDatabase);
示例
尝试以下示例以删除 MongoDB 服务器中的数据库:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); // Create a Command Instance $dropDatabase = new MongoDB\Driver\Command(["dropDatabase" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $dropDatabase); echo "Database dropped." } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Database dropped.
PHP & MongoDB - 创建集合
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个命令来创建集合。
// Create a Command Instance $createCollection = new MongoDB\Driver\Command(["create" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection);
示例
尝试以下示例以在 MongoDB 服务器中创建集合:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); // Create a Command Instance $createCollection = new MongoDB\Driver\Command(["create" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection); echo "Collection created."; } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Collection created.
PHP & MongoDB - 删除集合
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个命令来删除集合。
// Create a Command Instance $createCollection = new MongoDB\Driver\Command(["drop" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection);
示例
尝试以下示例以删除 MongoDB 服务器中的集合:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); // Create a Command Instance $dropCollection = new MongoDB\Driver\Command(["drop" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $dropCollection); echo "Collection dropped." } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Collection dropped.
PHP & MongoDB - 显示集合
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并在数据库上执行一个命令来显示可用的集合列表。
// Create a Command Instance $collectionList = new MongoDB\Driver\Command(["listCollections" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $collectionList);
示例
尝试以下示例以列出 MongoDB 服务器中数据库的集合:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); // Create a Command Instance $collectionList = new MongoDB\Driver\Command(["listCollections" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $collectionList); $collections = $cursor->toArray(); foreach ($collections as $collection) { echo $collection->name . "<br/>"; } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
sampleCollection
PHP & MongoDB - 插入文档
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个 bulkWrite 对象以在集合中插入记录。
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['First_Name' => "Mahesh", 'Last_Name' => 'Parashar', 'Date_Of_Birth' => '1990-08-21', 'e_mail' => 'mahesh_parashar.123@gmail.com', 'phone' => '9034343345']); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
示例
尝试以下示例以在 MongoDB 服务器中的集合中插入文档:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['First_Name' => "Mahesh", 'Last_Name' => 'Parashar', 'Date_Of_Birth' => '1990-08-21', 'e_mail' => 'mahesh_parashar.123@gmail.com', 'phone' => '9034343345']); $bulk->insert(['First_Name' => "Radhika", 'Last_Name' => 'Sharma', 'Date_Of_Birth' => '1995-09-26', 'e_mail' => 'radhika_sharma.123@gmail.com', 'phone' => '9000012345']); $bulk->insert(['First_Name' => "Rachel", 'Last_Name' => 'Christopher', 'Date_Of_Birth' => '1990-02-16', 'e_mail' => 'rachel_christopher.123@gmail.com', 'phone' => '9000054321']); $bulk->insert(['First_Name' => "Fathima", 'Last_Name' => 'Sheik', 'Date_Of_Birth' => '1990-02-16', 'e_mail' => 'fathima_sheik.123@gmail.com', 'phone' => '9000012345']); // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); printf("Inserted %d document(s).\n", $result->getInsertedCount()); } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Inserted 4 document(s).
PHP & MongoDB - 选择文档
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个 Query 对象以在集合中选择记录。
// Create a Query Object $query = new MongoDB\Driver\Query(['First_Name' => "Radhika"]); // Execute the query $rows = $manager->executeQuery("testdb.sampleCollection", $query);
示例
尝试以下示例以在 MongoDB 服务器中选择文档:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); $filter = []; // Create a Query Object $query = new MongoDB\Driver\Query($filter); // Execute the query $rows = $manager->executeQuery("myDb.sampleCollection", $query); foreach ($rows as $row) { printf("First Name: %s, Last Name: %s.<br/>", $row->First_Name, $row->Last_Name); } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
First Name: Radhika, Last Name: Sharma. First Name: Rachel, Last Name: Christopher. First Name: Fathima, Last Name: Sheik.
PHP & MongoDB - 更新文档
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个 bulkWrite 对象以更新集合中的记录。
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->update(['First_Name' => "Mahesh"],['$set' => ['e_mail' => 'maheshparashar@gmail.com']]); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
示例
尝试以下示例以更新 MongoDB 服务器中的文档:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->update(['First_Name' => "Mahesh"],['$set' => ['e_mail' => 'maheshparashar@gmail.com']]); // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); printf("Updated %d document(s).\n", $result->getModifiedCount()); } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Updated 1 document(s).
PHP & MongoDB - 删除文档
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个 bulkWrite 对象以删除集合中的记录。
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->delete(['First_Name' => "Mahesh"]); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
示例
尝试以下示例以删除 MongoDB 服务器中集合的文档:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->delete(['First_Name' => "Mahesh"]); // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); echo "Document deleted." } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Document deleted.
PHP & MongoDB - 插入嵌入式文档
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个 bulkWrite 对象以在集合中插入包含嵌入式文档的记录。
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['title' => "MongoDB Overview", 'description' => 'MongoDB is no SQL database', 'by' => 'tutorials point', 'url' => 'https://tutorialspoint.com', 'comments' => [ ['user' => "user1", 'message' => "My First Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ['user' => "user2", 'message' => "My Second Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ]]); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
示例
尝试以下示例以在 MongoDB 服务器中的集合中插入包含嵌入式文档的文档:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['title' => "MongoDB Overview", 'description' => 'MongoDB is no SQL database', 'by' => 'tutorials point', 'url' => 'https://tutorialspoint.com', 'comments' => [ ['user' => "user1", 'message' => "My First Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ['user' => "user2", 'message' => "My Second Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ]]); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); printf("Inserted %d document(s).\n", $result->getInsertedCount()); } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Inserted 1 document(s).
PHP & MongoDB - 错误处理
如果任何数据库操作发生异常,MongoDB 驱动程序操作会抛出异常,并且可以使用以下语法轻松捕获。
try { } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "<br/>"; echo "File:", $e->getFile(), "<br/>"; echo "Line:", $e->getLine(), "<br/>"; }
示例
尝试以下示例以检查 MongoDB 服务器中的数据库操作错误:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); // Create a Command Instance $createCollection = new MongoDB\Driver\Command(["create" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection); echo "Collection created."; } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "<br/>"; echo "File:", $e->getFile(), "<br/>"; echo "Line:", $e->getLine(), "<br/>"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
Exception:Collection already exists. NS: myDb.sampleCollection File: \htdocs\php_mongodb\mongodb_example.php Line:10
PHP & MongoDB - 限制记录
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个 Query 对象以在集合中选择记录,并传递过滤器和选项以限制搜索结果。
$filter = []; $options = ['limit' => 1]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("testdb.sampleCollection", $query);
示例
尝试以下示例以限制 MongoDB 服务器中的搜索结果:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); $filter = []; $options = ['limit' => 1]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("myDb.sampleCollection", $query); foreach ($rows as $row) { printf("First Name: %s, Last Name: %s.<br/>", $row->First_Name, $row->Last_Name); } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
First Name: Radhika, Last Name: Sharma.
PHP & MongoDB - 排序记录
执行任何操作的第一步是创建一个 Manager 实例。
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017");
第二步是准备并执行一个 Query 对象以在集合中选择记录,并传递过滤器和选项以对记录进行排序。
$filter = []; // Sort in Descending Order, For ascending order pass 1 $options = ['sort' => ['First_Name' => -1]]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("testdb.sampleCollection", $query);
示例
尝试以下示例以限制 MongoDB 服务器中的搜索结果:
复制并粘贴以下示例作为 mongodb_example.php:
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://127.0.0.1:27017"); $filter = []; $options = ['limit' => 3, 'sort' => ['First_Name' => -1]]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("myDb.sampleCollection", $query); foreach ($rows as $row) { printf("First Name: %s, Last Name: %s.<br/>", $row->First_Name, $row->Last_Name); } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
输出
访问部署在 apache web 服务器上的 mongodb_example.php 并验证输出。
First Name: Radhika, Last Name: Sharma. First Name: Rachel, Last Name: Christopher. First Name: Fathima, Last Name: Sheik.