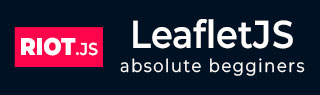
RIOT.JS - 快速指南
RIOT.JS - 概述
RIOT.js 是一个非常小巧/轻量级的基于 Web Components 的 UI 库,用于开发 Web 应用程序。它结合了 React.JS 和 Polymer 的优势,具有非常简洁的实现和简单的结构,易于学习和使用。它的压缩版本大小接近 10KB。
以下是 RIOT.js 的主要特性
表达式绑定
DOM 更新和重绘期间的有效载荷非常小。
更改从父标签向下传播到子标签/控件。
使用预编译的表达式并将其缓存以提高性能。
提供对生命周期事件的良好控制。
遵循标准
没有专有的事件系统
不依赖任何 polyfill 库。
没有向现有的 HTML 添加额外的属性。
与 jQuery 集成良好。
核心价值观
RIOT.js 的开发考虑了以下价值观。
简单且极简主义。
易于学习和实施。
提供响应式视图来构建用户界面。
提供事件库来构建具有独立模块的 API。
处理浏览器后退按钮的应用程序行为。
RIOT.JS - 环境设置
有两种方法可以使用 RIOT js。
本地安装 - 您可以在本地机器上下载 RIOT 库,并将其包含在您的 HTML 代码中。
基于 CDN 的版本 - 您可以直接从内容分发网络 (CDN) 将 RIOT 库包含到您的 HTML 代码中。
本地安装
访问 https://v3.riotjs.vercel.app/download/ 下载最新版本。
现在将下载的 riot.min.js 文件放入您网站的目录中,例如 /riotjs。
示例
现在您可以如下在 HTML 文件中包含 riotjs 库 -
<!DOCTYPE html> <html> <head> <script src = "/riotjs/riot.min.js"></script> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
这将产生以下结果 -
基于 CDN 的版本
您可以直接从内容分发网络 (CDN) 将 RIOT js 库包含到您的 HTML 代码中。Google 和 Microsoft 提供了最新版本的內容分发。
注意 - 在本教程中,我们始终使用 CDNJS 版本的库。
示例
现在让我们使用来自 CDNJS 的 jQuery 库重写上面的示例。
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 第一个应用程序
RIOT 通过构建自定义的可重用 html 标签来工作。这些标签类似于 Web Components,可以在页面和 Web 应用程序之间重用。
使用 RIOT 的步骤
在 html 页面中导入 riot.js。
<head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head>
开始一个脚本部分,并将标签内容定义为 html。脚本也可以包含在内,我们将在本教程后面的部分看到。
var tagHtml = "<h1>Hello World!</h1>";
使用 riot.tag() 方法定义一个标签。将标签的名称 messageTag 和包含标签内容的变量传递给它。
riot.tag("messageTag", tagHtml);
使用 riot.mount() 方法挂载标签。将标签的名称 messageTag 传递给它。挂载过程将 messageTag 挂载到 html 页面中所有出现的位置。MessageTag 标签应在挂载之前使用 riot.js 定义。
riot.mount("messageTag"); </script>
以下是完整的示例。
示例
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 标签
RIOT 通过构建自定义的可重用 html 标签来工作。这些标签类似于 Web Components,可以在页面和 Web 应用程序之间重用。当您在 HTML 页面中包含 RIOT 框架时,导入的 js 会创建一个指向 riot 对象的 riot 变量。此对象包含与 RIOT.js 交互所需的功能,例如创建和挂载标签。
我们可以通过两种方式创建和使用标签。
内联 HTML - 通过调用 riot.tag() 函数。此函数采用标签名称和标签定义来创建一个标签。标签定义可以包含 HTML、JavaScript 和 CSS 等。
单独的标签文件 - 通过将标签定义存储在标签文件中。此标签文件包含创建标签的标签定义。此文件需要导入到 riot.tag() 调用的位置。
<script src = "/riotjs/src/messageTag.tag" type = "riot/tag"></script<
以下是内联标签的示例。
示例
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
这将产生以下结果 -
以下是外部文件标签的示例。
示例
messageTag.tag
<messageTag> <h1>Hello World!</h1> </messageTag>
index.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script src = "messageTag.tag" type = "riot/tag"></script> <script> riot.mount("messageTag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 表达式
RIOT js 使用 {} 来定义表达式。RIOT js 允许以下类型的表达式。
简单表达式 - 定义一个变量并在标签中使用。
<customTag> <h1>{title}</h1> <script> this.title = "Welcome to TutorialsPoint.COM"; </script> </customTag>
评估表达式 - 在运算中使用时评估变量。
<customTag> <h2>{val * 5}</h2> <script> this.val = 4; </script> </customTag>
从 Options 对象获取值 - 获取通过属性传递给标签的值。
示例
以下是上述概念的完整示例。
customTag.tag
<customTag> <h1>{title}</h1> <h2>{val * 5}</h2> <h2>{opts.color}</h2> <script> this.title = "Welcome to TutorialsPoint.COM"; this.val = 4; </script> </customTag>
index.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <customTag color="red"></customTag> <script src = "customTag.tag" type = "riot/tag"></script> <script> riot.mount("customTag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 样式
RIOT js 标签可以有自己的样式,我们可以在标签内定义样式,这将仅影响标签内的内容。我们也可以在标签内的脚本中设置样式类。以下是实现 RIOT 标签样式的语法。
custom1Tag.tag
<custom1Tag> <h1>{title}</h1> <h2 class = "subTitleClass">{subTitle}</h2> <style> h1 { color: red; } .subHeader { color: green; } </style> <script> this.title = "Welcome to TutorialsPoint.COM"; this.subTitle = "Learning RIOT JS"; this.subTitleClass = "subHeader"; </script> </custom1Tag>
index.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <h1>Non RIOT Heading</h1> <custom1Tag></custom1Tag> <script src = "custom1Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom1Tag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 条件语句
条件语句是用于显示/隐藏 RIOT 标签元素的结构。以下是 RIOT 支持的三种条件语句 -
if - 根据传递的值添加/删除元素。
<custom2Tag> <h2 if = {showMessage}>Using if!</h2> <script> this.showMessage = true; </script> </custom2Tag>
show - 如果传递 true,则使用 style = "display:' ' " 显示元素。
<custom2Tag> <h2 show = {showMessage}>Using show!</h2> <script> this.showMessage = true; </script> </custom2Tag>
hide - 如果传递 true,则使用 style = "display:'none' " 隐藏元素。
<custom2Tag> <h2 show = {showMessage}>Using show!</h2> <script> this.showMessage = true; </script> </custom2Tag>
示例
以下是完整的示例。
custom2Tag.tag
<custom2Tag> <h2 if = {showMessage}>Using if!</h2> <h2 if = {show}>Welcome!</h1> <h2 show = {showMessage}>Using show!</h2> <h2 hide = {show}>Using hide!</h2> <script> this.showMessage = true; this.show = false; </script> </custom2Tag>
custom2.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom2Tag></custom2Tag> <script src = "custom2Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom2Tag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - Yield
Yield 是一种将外部 html 内容放入 RIOT 标签的机制。有多种方法可以执行 yield。
简单 Yield - 如果我们想替换标签中的单个占位符。然后使用此机制。
<custom3Tag> Hello <yield/> </custom3Tag>
<custom3Tag><b>User</b></custom3Tag>
多个 Yield - 如果我们想替换标签中的多个占位符。然后使用此机制。
<custom4Tag> <br/><br/> Hello <yield from = "first"/> <br/><br/> Hello <yield from = "second"/> </custom4Tag>
<custom4Tag> <yield to = "first">User 1</yield> <yield to = "second">User 2</yield> </custom4Tag>
示例
以下是完整的示例。
custom3Tag.tag
<custom3Tag> Hello <yield/> </custom3Tag>
custom4Tag.tag
<custom4Tag> <br/><br/> Hello <yield from = "first"/> <br/><br/> Hello <yield from = "second"/> </custom4Tag>
custom3.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom3Tag><b>User</b></custom3Tag> <custom4Tag> <yield to = "first">User 1</yield> <yield to = "second">User 2</yield> </custom4Tag> <script src = "custom3Tag.tag" type = "riot/tag"></script> <script src = "custom4Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom3Tag"); riot.mount("custom4Tag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 事件处理
我们可以像使用 refs 对象访问 HTML 元素一样,将事件附加到 HTML 元素上。第一步是在 DOM 元素上添加 ref 属性,并在标签的脚本块中使用 this.ref 访问它。
附加 ref - 在 DOM 元素上添加 ref 属性。
<button ref = "clickButton">Click Me!</button>
使用 refs 对象 - 现在在 mount 事件中使用 refs 对象。当 RIOT 挂载自定义标签时,此事件会被触发,并且它会填充 refs 对象。
this.on("mount", function() { console.log("Mounting"); console.log(this.refs.username.value); })
示例
以下是完整的示例。
custom5Tag.tag
<custom5Tag> <form> <input ref = "username" type = "text" value = "Mahesh"/> <input type = "submit" value = "Click Me!" /> </form> <script> this.on("mount", function() { console.log("Mounting"); console.log(this.refs.username.value); }) </script> </custom5Tag>
custom5.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom5Tag></custom5Tag> <script src = "custom5Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom5Tag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 访问DOM
我们可以使用 refs 对象访问 HTML 元素。第一步是在 DOM 元素上添加 ref 属性,并在标签的脚本块中使用 this.ref 访问它。
附加 ref - 在 DOM 元素上添加 ref 属性。
<button ref = "clickButton">Click Me!</button>
使用 refs 对象 - 现在在 mount 事件中使用 refs 对象。当 RIOT 挂载自定义标签时,此事件会被触发,并且它会填充 refs 对象。
this.on("mount", function() { this.refs.clickButton.onclick = function(e) { console.log("clickButton clicked"); return false; }; })
示例
以下是完整的示例。
custom6Tag.tag
<custom6Tag> <form ref = "customForm"> <input ref = "username" type = "text" value = "Mahesh"/> <button ref = "clickButton">Click Me!</button> <input type = "submit" value = "Submit" /> </form> <script> this.on("mount", function() { this.refs.clickButton.onclick = function(e) { console.log("clickButton clicked"); return false; }; this.refs.customForm.onsubmit = function(e) { console.log("Form submitted"); return false; }; }) </script> </custom6Tag>
custom6.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom6Tag></custom6Tag> <script src = "custom6Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom6Tag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 循环
我们可以遍历 RIOT 的基本类型或对象的数组,并在运行时创建/更新 html 元素。使用“each”结构可以实现它。
创建数组 - 创建一个对象数组。
this.cities = [ { city : "Shanghai" , country:"China" , done: true }, { city : "Seoul" , country:"South Korea" }, { city : "Moscow" , country:"Russia" } ];
添加 each 属性 - 现在使用“each”属性。
<ul> <li each = { cities } ></li> </ul>
迭代对象数组 - 使用对象属性迭代数组。
<input type = "checkbox" checked = { done }> { city } - { country }
示例
以下是完整的示例。
custom7Tag.tag
<custom7Tag> <style> ul { list-style-type: none; } </style> <ul> <li each = { cities } > <input type = "checkbox" checked = { done }> { city } - { country } </li> </ul> <script> this.cities = [ { city : "Shanghai" , country:"China" , done: true }, { city : "Seoul" , country:"South Korea" }, { city : "Moscow" , country:"Russia" } ]; </script> </custom7Tag>
custom7.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom7Tag></custom6Tag> <script src = "custom7Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom7Tag"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - Mixin
通过 Mixin,我们可以在标签之间共享通用功能。Mixin 可以是函数、类或对象。考虑一个每个标签都应该使用的身份验证服务的案例。
定义 Mixin - 在调用 mount() 之前,使用 riot.mixin() 方法定义 mixin。
riot.mixin('authService', { init: function() { console.log('AuthService Created!') }, login: function(user, password) { if(user == "admin" && password == "admin"){ return 'User is authentic!' }else{ return 'Authentication failed!' } } });
初始化 mixin - 在每个标签中初始化 mixin。
this.mixin('authService')
使用 mixin - 初始化后,可以在标签内使用 mixin。
this.message = this.login("admin","admin");
示例
以下是完整的示例。
custom8Tag.tag
<custom8Tag> <h1>{ message }</h1> <script> this.mixin('authService') this.message = this.login("admin","admin") </script> </custom8Tag>
custom9Tag.tag
<custom9Tag> <h1>{ message }</h1> <script> this.mixin('authService') this.message = this.login("admin1","admin") </script> </custom9Tag>
custom8.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom8Tag></custom8Tag> <custom9Tag></custom9Tag> <script src = "custom8Tag.tag" type = "riot/tag"></script> <script src = "custom9Tag.tag" type = "riot/tag"></script> <script> riot.mixin('authService', { init: function() { console.log('AuthService Created!') }, login: function(user, password) { if(user == "admin" && password == "admin"){ return 'User is authentic!' }else{ return 'Authentication failed!' } } }); riot.mount("*"); </script> </body> </html>
这将产生以下结果 -
RIOT.JS - 可观察对象
可观察对象机制允许 RIOT 从一个标签向另一个标签发送事件。以下 API 对于理解 RIOT 可观察对象非常重要。
riot.observable(element) - 为给定的对象元素添加观察者支持,或者如果参数为空,则创建一个新的可观察对象实例并返回。之后,对象能够触发和监听事件。
var EventBus = function(){ riot.observable(this); }
element.trigger(events) - 执行所有监听给定事件的回调函数。
sendMessage() { riot.eventBus.trigger('message', 'Custom 10 Button Clicked!'); }
element.on(events, callback) - 监听给定事件,并在每次触发事件时执行回调函数。
riot.eventBus.on('message', function(input) { console.log(input); });
示例
以下是完整的示例。
custom10Tag.tag
<custom10Tag> <button onclick = {sendMessage}>Custom 10</button> <script> sendMessage() { riot.eventBus.trigger('message', 'Custom 10 Button Clicked!'); } </script> </custom10Tag>
custom11Tag.tag
<custom11Tag> <script> riot.eventBus.on('message', function(input) { console.log(input); }); </script> </custom11Tag>
custom9.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom10Tag></custom10Tag> <custom11Tag></custom11Tag> <script src = "custom10Tag.tag" type = "riot/tag"></script> <script src = "custom11Tag.tag" type = "riot/tag"></script> <script> var EventBus = function(){ riot.observable(this); } riot.eventBus = new EventBus(); riot.mount("*"); </script> </body> </html>
这将产生以下结果 -