在Android模拟器上运行Google Maps v2
介绍
如今许多Android应用程序都使用Google Maps来向用户显示地图。例如,在许多外卖应用程序中,我们可以看到订单状态以及送货员在Google Maps上的位置。在本文中,我们将了解如何在Android模拟器上运行Google Maps v2。
实现
我们将创建一个简单的应用程序,在这个应用程序中,我们将显示一个简单的Google地图,并在该地图上添加一个标记到德里位置。我们将遵循逐步指南来构建此应用程序。现在让我们转向Android Studio创建一个新项目。
步骤1:在Android Studio中创建一个新项目
导航到Android Studio,如下面的屏幕所示。在下面的屏幕中,单击“新建项目”以创建一个新的Android Studio项目。
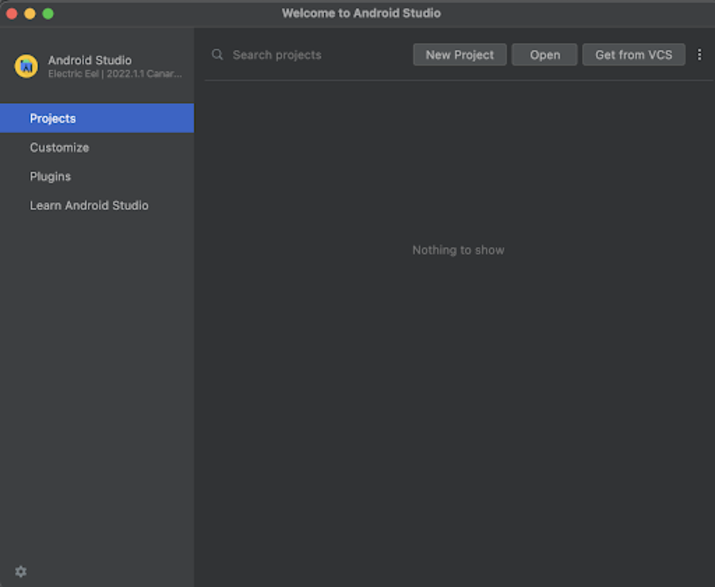
单击“新建项目”后,您将看到下面的屏幕。
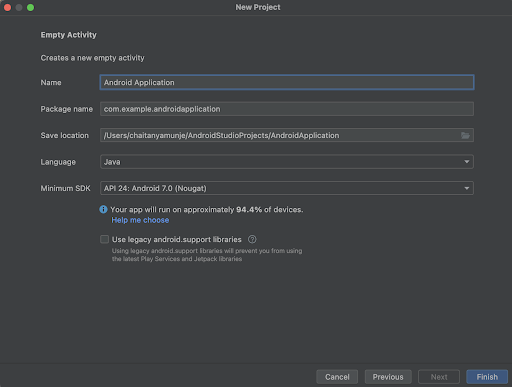
在这个屏幕中,我们只需选择Google Maps Activity并单击“下一步”。单击“下一步”后,您将看到下面的屏幕。
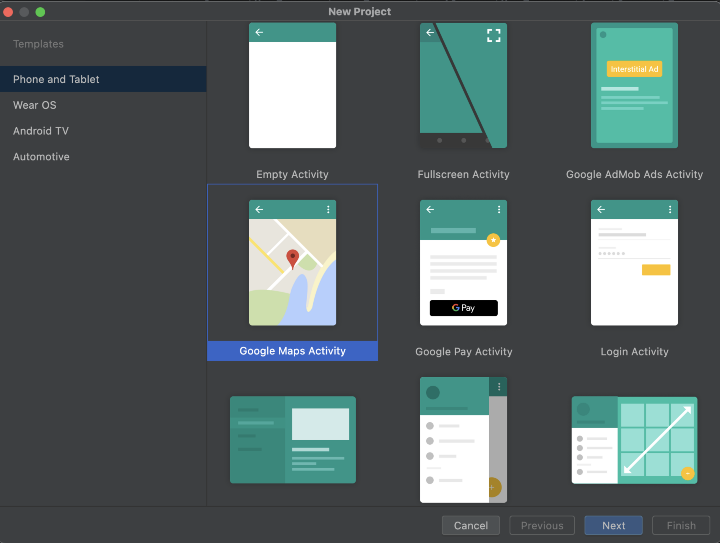
在这个屏幕中,我们只需指定项目名称。然后包名将自动生成。
注意 − 确保选择Java作为语言。
指定所有详细信息后,单击“完成”以创建一个新的Android Studio项目。
项目创建完成后,我们将看到两个打开的文件:activity_maps.xml和MapsActivity.java文件。
步骤2:创建API密钥以显示Google Maps
访问以下URL,您将找到关于如何创建Google Maps API密钥的详细文档,我们必须将其添加到我们的应用程序中才能显示Google Maps。
步骤3:使用AndroidManifest.xml文件
现在,我们已经生成了Google Maps API密钥,我们必须在项目的AndroidManifest.xml文件中使用此密钥。导航到app>AndroidManifest.xml文件,并将以下代码添加到其中。代码中添加了注释以便详细了解。
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"> <application android:allowBackup="true" android:dataExtractionRules="@xml/data_extraction_rules" android:fullBackupContent="@xml/backup_rules" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.GoogleMapsProject" tools:targetApi="31"> <!-- TODO: Before you run your application, you need a Google Maps API key. To get one, follow the directions here: https://developers.google.com/maps/documentation/android-sdk/get-api-key Once you have your API key (it starts with "AIza"), define a new property in your project's local.properties file (e.g. MAPS_API_KEY=Aiza...), and replace the "YOUR_API_KEY" string in this file with "${MAPS_API_KEY}". --> <meta-data android:name="com.google.android.geo.API_KEY" android:value="Enter your API key here" /> <activity android:name=".MapsActivity" android:exported="true" android:label="@string/title_activity_maps"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
注意 − 确保将您生成的API密钥添加到“输入您的API密钥”标签的位置。
步骤4:使用activity_main.xml
导航到activity_maps.xml。如果此文件不可见,则要打开此文件。在左侧窗格中导航到app>res>layout>activity_maps.xml以打开此文件。打开此文件后,将以下代码添加到其中。代码中添加了注释以便详细了解。
<?xml version="1.0" encoding="utf-8"?> <!-- on below line creating a fragment for displaying maps--> <fragment xmlns:android="http://schemas.android.com/apk/res/android" xmlns:map="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/map" android:name="com.google.android.gms.maps.SupportMapFragment" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MapsActivity" />
说明 − 在上面的代码中,我们正在创建一个片段,我们将在其中显示应用程序内的Google地图。我们为这个片段指定了一个名为“支持地图片段”的名称。
步骤4:使用MapsActivity.java文件
导航到MainActivity.java。如果此文件不可见,则要打开此文件。在左侧窗格中导航到app>res>layout>MainActivity.java以打开此文件。打开此文件后,将以下代码添加到其中。代码中添加了注释以便详细了解。
package com.example.googlemapsproject; import androidx.fragment.app.FragmentActivity; import android.os.Bundle; import com.google.android.gms.maps.CameraUpdateFactory; import com.google.android.gms.maps.GoogleMap; import com.google.android.gms.maps.OnMapReadyCallback; import com.google.android.gms.maps.SupportMapFragment; import com.google.android.gms.maps.model.LatLng; import com.google.android.gms.maps.model.MarkerOptions; import com.example.googlemapsproject.databinding.ActivityMapsBinding; public class MapsActivity extends FragmentActivity implements OnMapReadyCallback { private GoogleMap mMap; private ActivityMapsBinding binding; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); binding = ActivityMapsBinding.inflate(getLayoutInflater()); setContentView(binding.getRoot()); // Obtain the SupportMapFragment and get notified when the map is ready to be used. SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager() .findFragmentById(R.id.map); mapFragment.getMapAsync(this); } /** * Manipulates the map once available. * This callback is triggered when the map is ready to be used. * This is where we can add markers or lines, add listeners or move the camera. In this case, * we just add a marker near Sydney, Australia. * If Google Play services is not installed on the device, the user will be prompted to install * it inside the SupportMapFragment. This method will only be triggered once the user has * installed Google Play services and returned to the app. */ @Override public void onMapReady(GoogleMap googleMap) { mMap = googleMap; // Add a marker in Delhi and move the camera LatLng sydney = new LatLng(28.7041, 77.1025); mMap.addMarker(new MarkerOptions().position(sydney).title("Marker in Delhi")); mMap.moveCamera(CameraUpdateFactory.newLatLng(sydney)); } }
说明 −在上面的代码中,首先我们为Google Maps和活动绑定创建变量。现在我们将看到onCreate方法。这是每个Android应用程序的默认方法。创建应用程序视图时将调用此方法。在此方法中,我们设置内容视图,即名为activity_maps.xml的布局文件,以设置该文件中的UI。在onCreate方法中,我们初始化绑定变量,然后为支持片段创建一个变量,并使用我们在activity_maps.xml文件中提供的片段ID初始化该变量。然后调用getMapAsync来同步我们的地图。之后,我们覆盖onMapReady方法。在此方法中,我们为将在德里位置显示的标记创建纬度和经度。然后我们在地图上添加一个标记。最后,将我们的相机移动到我们显示标记的位置。
添加上述代码后,我们只需单击顶部栏中的绿色图标即可在移动设备上运行我们的应用程序。
注意 − 确保已连接到您的真实设备或模拟器。
输出
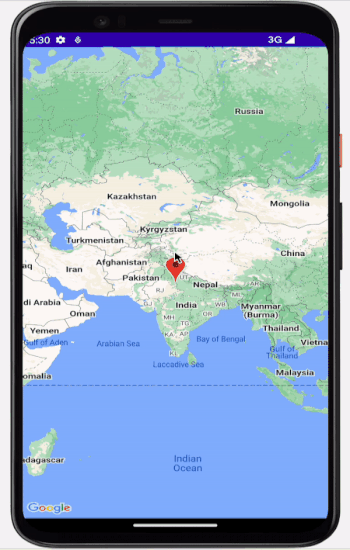
结论
在本文中,我们了解了如何在Android模拟器上的Android应用程序中运行Google Maps v2。