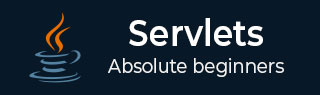
- Servlet 教程
- Servlet - 首页
- Servlet - 概述
- Servlet - 环境设置
- Servlet - 生命周期
- Servlet - 示例
- Servlet - 表单数据
- Servlet - 客户端请求
- Servlet - 服务器响应
- Servlet - HTTP 状态码
- Servlet - 编写过滤器
- Servlet - 异常处理
- Servlet - Cookie 处理
- Servlet - Session跟踪
- Servlet - 数据库访问
- Servlet - 文件上传
- Servlet - 日期处理
- Servlet - 页面重定向
- Servlet - 点击计数器
- Servlet - 自动刷新
- Servlet - 发送邮件
- Servlet - 打包
- Servlet - 调试
- Servlet - 国际化
- Servlet - 注解
- Servlet 有用资源
- Servlet - 常见问题解答
- Servlet - 快速指南
- Servlet - 有用资源
- Servlet - 讨论
Servlet - 国际化
在我们继续之前,让我解释三个重要的术语:
国际化 (i18n) - 这意味着使网站能够提供不同版本的翻译内容,以适应访问者的语言或国籍。
本地化 (l10n) - 这意味着向网站添加资源,以适应特定的地理区域或文化区域。
区域设置 (locale) - 这是一个特定的文化或地理区域。它通常指语言代码后跟国家/地区代码,两者之间用下划线分隔。例如,“en_US”表示美国的英语区域设置。
在构建全球性网站时,需要考虑许多因素。本教程不会提供这方面的完整细节,但它将提供一个很好的示例,说明如何通过区分访问者的位置(即区域设置)为互联网社区提供不同语言的网页。
Servlet可以根据请求者的区域设置选择合适的网站版本,并根据当地语言、文化和需求提供相应的网站版本。以下是request对象的返回Locale对象的方法。
java.util.Locale request.getLocale()
检测区域设置
以下是您可以用来检测请求者位置、语言和区域设置的重要区域设置方法。以下所有方法都显示在请求者浏览器中设置的国家/地区名称和语言名称。
序号 | 方法及描述 |
---|---|
1 | String getCountry() 此方法返回此区域设置的国家/地区代码(大写),采用ISO 3166 2字母格式。 |
2 | String getDisplayCountry() 此方法返回适合显示给用户的区域设置国家/地区的名称。 |
3 | String getLanguage() 此方法返回此区域设置的语言代码(小写),采用ISO 639格式。 |
4 | String getDisplayLanguage() 此方法返回适合显示给用户的区域设置语言的名称。 |
5 | String getISO3Country() 此方法返回此区域设置的国家/地区的三个字母缩写。 |
6 | String getISO3Language() 此方法返回此区域设置的语言的三个字母缩写。 |
示例
此示例演示如何显示请求的语言和关联国家/地区:
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import java.util.Locale; public class GetLocale extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //Get the client's Locale Locale locale = request.getLocale(); String language = locale.getLanguage(); String country = locale.getCountry(); // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); String title = "Detecting Locale"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; out.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#f0f0f0\">\n" + "<h1 align = \"center\">" + language + "</h1>\n" + "<h2 align = \"center\">" + country + "</h2>\n" + "</body> </html>" ); } }
语言设置
Servlet可以输出用西欧语言(如英语、西班牙语、德语、法语、意大利语、荷兰语等)编写的页面。这里重要的是设置ContentLanguage标头以正确显示所有字符。
第二点是使用HTML实体显示所有特殊字符,例如,“ñ”表示“ñ”,“¡”表示“¡”,如下所示
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import java.util.Locale; public class DisplaySpanish extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); // Set spanish language code. response.setHeader("Content-Language", "es"); String title = "En Español"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; out.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#f0f0f0\">\n" + "<h1>" + "En Español:" + "</h1>\n" + "<h1>" + "¡Hola Mundo!" + "</h1>\n" + "</body> </html>" ); } }
特定区域设置的日期
您可以使用java.text.DateFormat类及其静态getDateTimeInstance()方法来格式化特定于区域设置的日期和时间。以下示例演示如何格式化特定于给定区域设置的日期:
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import java.util.Locale; import java.text.DateFormat; import java.util.Date; public class DateLocale extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); //Get the client's Locale Locale locale = request.getLocale( ); String date = DateFormat.getDateTimeInstance(DateFormat.FULL, DateFormat.SHORT, locale).format(new Date( )); String title = "Locale Specific Dates"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; out.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#f0f0f0\">\n" + "<h1 align = \"center\">" + date + "</h1>\n" + "</body> </html>" ); } }
特定区域设置的货币
您可以使用java.txt.NumberFormat类及其静态getCurrencyInstance()方法以特定于区域设置的货币格式化数字(例如long或double类型)。以下示例演示如何格式化特定于给定区域设置的货币:
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import java.util.Locale; import java.text.NumberFormat; import java.util.Date; public class CurrencyLocale extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); //Get the client's Locale Locale locale = request.getLocale( ); NumberFormat nft = NumberFormat.getCurrencyInstance(locale); String formattedCurr = nft.format(1000000); String title = "Locale Specific Currency"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; out.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#f0f0f0\">\n" + "<h1 align = \"center\">" + formattedCurr + "</h1>\n" + "</body> </html>" ); } }
特定区域设置的百分比
您可以使用java.txt.NumberFormat类及其静态getPercentInstance()方法获取特定于区域设置的百分比。以下示例演示如何格式化特定于给定区域设置的百分比:
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import java.util.Locale; import java.text.NumberFormat; import java.util.Date; public class PercentageLocale extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); //Get the client's Locale Locale locale = request.getLocale( ); NumberFormat nft = NumberFormat.getPercentInstance(locale); String formattedPerc = nft.format(0.51); String title = "Locale Specific Percentage"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; out.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#f0f0f0\">\n" + "<h1 align = \"center\">" + formattedPerc + "</h1>\n" + "</body> </html>" ); } }