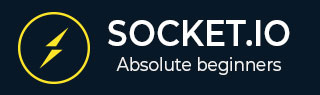
- Socket.IO 教程
- Socket.IO - 首页
- Socket.IO - 概述
- Socket.IO - 环境配置
- Socket.IO - Hello world
- Socket.IO - 事件处理
- Socket.IO - 广播
- Socket.IO - 命名空间
- Socket.IO - 房间
- Socket.IO - 错误处理
- Socket.IO - 日志记录与调试
- Socket.IO - 内部机制
- Socket.IO - 聊天应用
- Socket.IO 有用资源
- Socket.IO - 快速指南
- Socket.IO - 有用资源
- Socket.IO - 讨论区
Socket.IO - 事件处理
Sockets的工作机制基于事件。服务器端可以使用socket对象访问一些保留事件。
这些事件包括:
- 连接 (Connect)
- 消息 (Message)
- 断开连接 (Disconnect)
- 重新连接 (Reconnect)
- Ping
- 加入 (Join) 和
- 离开 (Leave)。
客户端的socket对象也提供了一些保留事件,包括:
- 连接 (Connect)
- 连接错误 (Connect_error)
- 连接超时 (Connect_timeout)
- 重新连接等等 (Reconnect, etc.)
现在,让我们来看一个使用SocketIO库处理事件的例子。
示例 1
In the Hello World example, we used the connection and disconnection events to log when a user connected and left. Now we will be using the message event to pass message from the server to the client. To do this, modify the io.on ('connection', function(socket)) as shown below –var app = require('express')(); var http = require('http').Server(app); var io = require('socket.io')(http); app.get('/', function(req, res){ res.sendFile('E:/test/index.html'); }); io.on('connection', function(socket){ console.log('A user connected'); // Send a message after a timeout of 4seconds setTimeout(function(){ socket.send('Sent a message 4seconds after connection!'); }, 4000); socket.on('disconnect', function () { console.log('A user disconnected'); }); }); http.listen(3000, function(){ console.log('listening on *:3000'); });
客户端连接四秒后,这段代码将发送一个名为message(内置)的事件到客户端。socket 对象上的send函数与'message'事件关联。
现在,我们需要在客户端处理这个事件。为此,请将index.html页面的内容替换为以下内容:
<!DOCTYPE html> <html> <head><title>Hello world</title></head> <script src="/socket.io/socket.io.js"></script> <script> var socket = io(); socket.on('message', function(data){document.write(data)}); </script> <body>Hello world</body> </html>
我们现在正在客户端处理'message'事件。当您现在在浏览器中访问该页面时,您将看到以下截图。
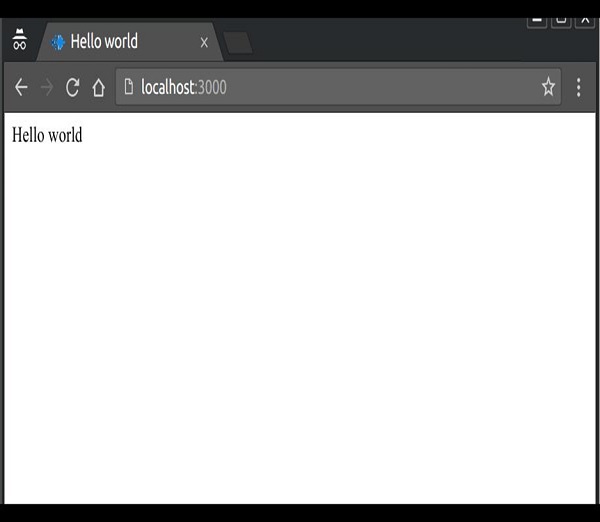
4秒后服务器发送message事件,客户端将处理它并产生以下输出:
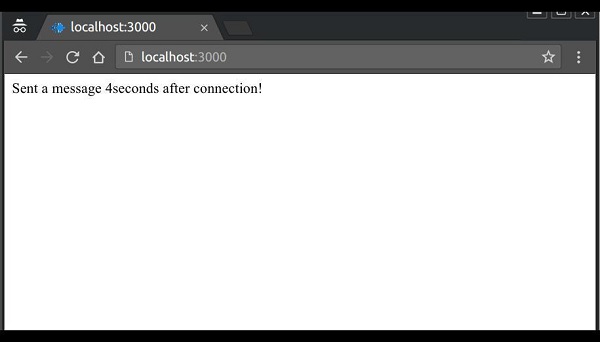
注意 - 我们这里发送的是文本字符串;我们也可以在任何事件中发送对象。
Message 是 API 提供的内置事件,但在实际应用中用处不大,因为我们需要能够区分不同的事件。
为了实现这一点,Socket.IO允许我们创建自定义事件。您可以使用socket.emit函数创建和触发自定义事件。以下代码发出一个名为testerEvent的事件:
var app = require('express')(); var http = require('http').Server(app); var io = require('socket.io')(http); app.get('/', function(req, res){ res.sendFile('E:/test/index.html'); }); io.on('connection', function(socket){ console.log('A user connected'); // Send a message when setTimeout(function(){ // Sending an object when emmiting an event socket.emit('testerEvent', { description: 'A custom event named testerEvent!'}); }, 4000); socket.on('disconnect', function () { console.log('A user disconnected'); }); }); http.listen(3000, function(){ console.log('listening on localhost:3000'); });
为了在客户端处理这个自定义事件,我们需要一个监听器来监听testerEvent事件。以下代码在客户端处理此事件:
<!DOCTYPE html> <html> <head><title>Hello world</title></head> <script src="/socket.io/socket.io.js"></script> <script> var socket = io(); socket.on('testerEvent', function(data){document.write(data.description)}); </script> <body>Hello world</body> </html>
这将与我们之前的示例一样工作,只是事件变成了testerEvent。当您打开浏览器并访问localhost:3000时,您将看到:
Hello world
四秒钟后,此事件将被触发,浏览器中的文本将更改为:
A custom event named testerEvent!
示例 2
我们也可以从客户端发出事件。要从客户端发出事件,请使用socket对象上的emit函数。
<!DOCTYPE html> <html> <head><title>Hello world</title></head> <script src="/socket.io/socket.io.js"></script> <script> var socket = io(); socket.emit('clientEvent', 'Sent an event from the client!'); </script> <body>Hello world</body> </html>
要处理这些事件,请在服务器上的socket对象上使用on函数。
var app = require('express')(); var http = require('http').Server(app); var io = require('socket.io')(http); app.get('/', function(req, res){ res.sendFile('E:/test/index.html'); }); io.on('connection', function(socket){ socket.on('clientEvent', function(data){ console.log(data); }); }); http.listen(3000, function(){ console.log('listening on localhost:3000'); });
因此,现在如果我们访问localhost:3000,我们将触发一个名为clientEvent的自定义事件。此事件将在服务器端通过记录以下内容进行处理:
Sent an event from the client!