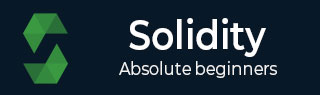
- Solidity 教程
- Solidity - 首页
- Solidity - 概述
- Solidity - 环境搭建
- Solidity - 基本语法
- Solidity - 第一个应用
- Solidity - 注释
- Solidity - 数据类型
- Solidity - 变量
- Solidity - 变量作用域
- Solidity - 运算符
- Solidity - 循环
- Solidity - 条件语句
- Solidity - 字符串
- Solidity - 数组
- Solidity - 枚举
- Solidity - 结构体
- Solidity - 映射
- Solidity - 类型转换
- Solidity - 以太币单位
- Solidity - 特殊变量
- Solidity - 样式指南
- Solidity 函数
- Solidity - 函数
- Solidity - 函数修饰符
- Solidity - View 函数
- Solidity - Pure 函数
- Solidity - 回退函数
- 函数重载
- 数学函数
- 加密函数
- Solidity 常用模式
- Solidity - 提款模式
- Solidity - 受限访问
- Solidity 高级特性
- Solidity - 合约
- Solidity - 继承
- Solidity - 构造函数
- Solidity - 抽象合约
- Solidity - 接口
- Solidity - 库
- Solidity - 汇编
- Solidity - 事件
- Solidity - 错误处理
- Solidity 有用资源
- Solidity - 快速指南
- Solidity - 有用资源
- Solidity - 讨论
Solidity - 提款模式
提款模式确保不会进行直接转账调用,这会带来安全风险。以下合约展示了不安全的转账调用方式来发送以太币。
pragma solidity ^0.5.0; contract Test { address payable public richest; uint public mostSent; constructor() public payable { richest = msg.sender; mostSent = msg.value; } function becomeRichest() public payable returns (bool) { if (msg.value > mostSent) { // Insecure practice richest.transfer(msg.value); richest = msg.sender; mostSent = msg.value; return true; } else { return false; } } }
上述合约可以通过使最富有者成为回退函数失败的合约来使其处于不可用状态。当回退函数失败时,becomeRichest() 函数也会失败,合约将永远卡住。为了减轻这个问题,我们可以使用提款模式。
在提款模式中,我们将在每次转账前重置待处理金额。这将确保只有调用者合约失败。
pragma solidity ^0.5.0; contract Test { address public richest; uint public mostSent; mapping (address => uint) pendingWithdrawals; constructor() public payable { richest = msg.sender; mostSent = msg.value; } function becomeRichest() public payable returns (bool) { if (msg.value > mostSent) { pendingWithdrawals[richest] += msg.value; richest = msg.sender; mostSent = msg.value; return true; } else { return false; } } function withdraw() public { uint amount = pendingWithdrawals[msg.sender]; pendingWithdrawals[msg.sender] = 0; msg.sender.transfer(amount); } }
广告