使用OpenCV在Python中进行模板匹配
模板匹配是一种技术,可以通过它从实际图像中匹配补丁或模板。这基本上是一种模式匹配机制。
在Python中,有OpenCV模块。使用OpenCV,我们可以轻松找到匹配项。因此,在这个问题中,使用了OpenCV模板匹配技术。
要使用OpenCV功能,我们需要使用pip下载它们。
sudo pip3 install opencv-python
对于模板匹配任务,存在一个精度因子,此因子称为阈值。例如,我们可以说可以使用这种模板匹配方案轻松创建面部识别方案。我们可以提供一些眼睛或面部其他部位的图像,然后使用这些图像作为模板,它可以轻松找到匹配项,但眼睛存在不同的变化。因此,如果我们将精度级别设置为50%,它将比精度级别100%检测得更好。通常,在不同情况下,精度级别为80%。
模板匹配步骤
获取实际图像并将其转换为灰度图像。
将模板作为灰度图像
模板在实际图像上滑动并找到精度级别匹配的位置。
当结果大于精度级别时,将该位置标记为已检测到。
对于第一种情况,输入图像和模板为:
主图像
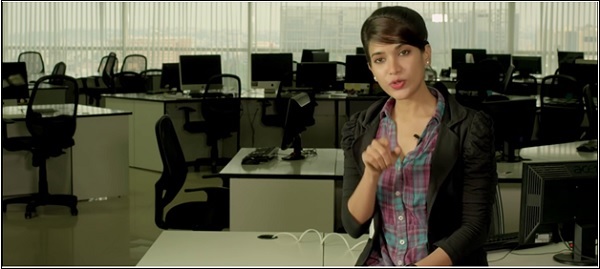
模板

示例代码
import cv2 import numpy as np #open the main image and convert it to gray scale image main_image = cv2.imread('main_image.png') gray_image = cv2.cvtColor(main_image, cv2.COLOR_BGR2GRAY) #open the template as gray scale image template = cv2.imread('template1.png', 0) width, height = template.shape[::-1] #get the width and height #match the template using cv2.matchTemplate match = cv2.matchTemplate(gray_image, template, cv2.TM_CCOEFF_NORMED) threshold = 0.8 position = np.where(match >= threshold) #get the location of template in the image for point in zip(*position[::-1]): #draw the rectangle around the matched template cv2.rectangle(main_image, point, (point[0] + width, point[1] + height), (0, 204, 153), 0) cv2.imshow('Template Found', main_image) cv2.waitKey(0)
输出
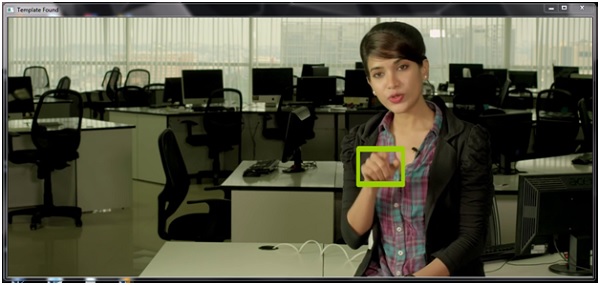
上述代码不支持多尺度。因此,如果模板的大小不同,它将无法检测到。因此,在下一部分中,我们将看到如何使用多尺度功能来检测模板。
在这种方法中,实际图像被转换为不同的尺寸,每次它都匹配模式,并找到最大的相关系数来定位匹配项。
这里的实际图像相同,模板在这里:
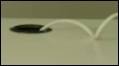
示例代码
import imutils import cv2 import numpy as np #Open template and get canny template = cv2.imread('template3.jpg') template = cv2.cvtColor(template, cv2.COLOR_BGR2GRAY) template = cv2.Canny(template, 10, 25) (height, width) = template.shape[:2] #open the main image and convert it to gray scale image main_image = cv2.imread('main_image.png') gray_image = cv2.cvtColor(main_image, cv2.COLOR_BGR2GRAY) temp_found = None for scale in np.linspace(0.2, 1.0, 20)[::-1]: #resize the image and store the ratio resized_img = imutils.resize(gray_image, width = int(gray_image.shape[1] * scale)) ratio = gray_image.shape[1] / float(resized_img.shape[1]) if resized_img.shape[0] < height or resized_img.shape[1] < width: break #Convert to edged image for checking e = cv2.Canny(resized_img, 10, 25) match = cv2.matchTemplate(e, template, cv2.TM_CCOEFF) (_, val_max, _, loc_max) = cv2.minMaxLoc(match) if temp_found is None or val_max>temp_found[0]: temp_found = (val_max, loc_max, ratio) #Get information from temp_found to compute x,y coordinate (_, loc_max, r) = temp_found (x_start, y_start) = (int(loc_max[0]), int(loc_max[1])) (x_end, y_end) = (int((loc_max[0] + width)), int((loc_max[1] + height))) #Draw rectangle around the template cv2.rectangle(main_image, (x_start, y_start), (x_end, y_end), (153, 22, 0), 5) cv2.imshow('Template Found', main_image) cv2.waitKey(0)
输出
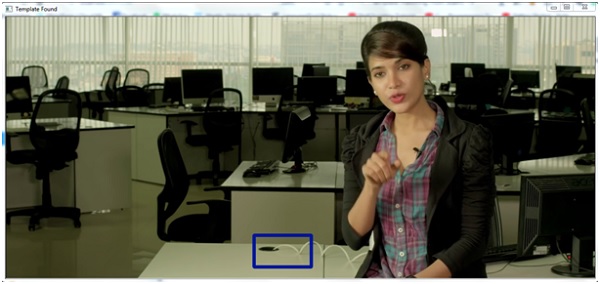
广告