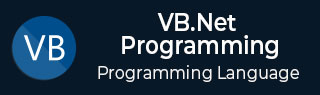
- VB.Net基础教程
- VB.Net - 首页
- VB.Net - 概述
- VB.Net - 环境设置
- VB.Net - 程序结构
- VB.Net - 基本语法
- VB.Net - 数据类型
- VB.Net - 变量
- VB.Net - 常量
- VB.Net - 修饰符
- VB.Net - 语句
- VB.Net - 指令
- VB.Net - 运算符
- VB.Net - 决策制定
- VB.Net - 循环
- VB.Net - 字符串
- VB.Net - 日期和时间
- VB.Net - 数组
- VB.Net - 集合
- VB.Net - 函数
- VB.Net - 子程序
- VB.Net - 类和对象
- VB.Net - 异常处理
- VB.Net - 文件处理
- VB.Net - 基本控件
- VB.Net - 对话框
- VB.Net - 高级窗体
- VB.Net - 事件处理
- VB.Net高级教程
- VB.Net - 正则表达式
- VB.Net - 数据库访问
- VB.Net - Excel表格
- VB.Net - 发送电子邮件
- VB.Net - XML处理
- VB.Net - Web编程
- VB.Net有用资源
- VB.Net - 快速指南
- VB.Net - 有用资源
- VB.Net - 讨论
从文本文件读取和写入文本文件
StreamReader 和 StreamWriter 类用于从文本文件读取数据和向文本文件写入数据。这些类继承自抽象基类 Stream,该基类支持将字节读写到文件流中。
StreamReader 类
StreamReader 类也继承自抽象基类 TextReader,该基类表示用于读取一系列字符的读取器。下表描述了 StreamReader 类的一些常用方法:
序号 | 方法名称及用途 |
---|---|
1 |
Public Overrides Sub Close 它关闭 StreamReader 对象和底层流,并释放与读取器关联的任何系统资源。 |
2 |
Public Overrides Function Peek As Integer 返回下一个可用字符,但不使用它。 |
3 |
Public Overrides Function Read As Integer 从输入流读取下一个字符,并将字符位置前进一个字符。 |
示例
以下示例演示了如何读取名为 Jamaica.txt 的文本文件。文件内容如下:
Down the way where the nights are gay And the sun shines daily on the mountain top I took a trip on a sailing ship And when I reached Jamaica I made a stop
Imports System.IO Module fileProg Sub Main() Try ' Create an instance of StreamReader to read from a file. ' The using statement also closes the StreamReader. Using sr As StreamReader = New StreamReader("e:/jamaica.txt") Dim line As String ' Read and display lines from the file until the end of ' the file is reached. line = sr.ReadLine() While (line <> Nothing) Console.WriteLine(line) line = sr.ReadLine() End While End Using Catch e As Exception ' Let the user know what went wrong. Console.WriteLine("The file could not be read:") Console.WriteLine(e.Message) End Try Console.ReadKey() End Sub End Module
猜猜编译并运行程序时会显示什么!
StreamWriter 类
StreamWriter 类继承自抽象类 TextWriter,该类表示一个写入器,可以写入一系列字符。
下表显示了此类的一些最常用的方法:
序号 | 方法名称及用途 |
---|---|
1 |
Public Overrides Sub Close 关闭当前 StreamWriter 对象和底层流。 |
2 |
Public Overrides Sub Flush 清除当前写入器的所有缓冲区,并导致任何缓冲数据写入底层流。 |
3 |
Public Overridable Sub Write (value As Boolean) 将布尔值的文本表示形式写入文本字符串或流。(继承自 TextWriter。) |
4 |
Public Overrides Sub Write (value As Char) 将字符写入流。 |
5 |
Public Overridable Sub Write (value As Decimal) 将十进制值的文本表示形式写入文本字符串或流。 |
6 |
Public Overridable Sub Write (value As Double) 将 8 字节浮点值的文本表示形式写入文本字符串或流。 |
7 |
Public Overridable Sub Write (value As Integer) 将 4 字节有符号整数的文本表示形式写入文本字符串或流。 |
8 |
Public Overrides Sub Write (value As String) 将字符串写入流。 |
9 |
Public Overridable Sub WriteLine 将行终止符写入文本字符串或流。 |
以上列表并不详尽。有关方法的完整列表,请访问 Microsoft 的文档
示例
以下示例演示了如何使用 StreamWriter 类将文本数据写入文件:
Imports System.IO Module fileProg Sub Main() Dim names As String() = New String() {"Zara Ali", _ "Nuha Ali", "Amir Sohel", "M Amlan"} Dim s As String Using sw As StreamWriter = New StreamWriter("names.txt") For Each s In names sw.WriteLine(s) Next s End Using ' Read and show each line from the file. Dim line As String Using sr As StreamReader = New StreamReader("names.txt") line = sr.ReadLine() While (line <> Nothing) Console.WriteLine(line) line = sr.ReadLine() End While End Using Console.ReadKey() End Sub End Module
编译并执行上述代码后,将产生以下结果:
Zara Ali Nuha Ali Amir Sohel M Amlan