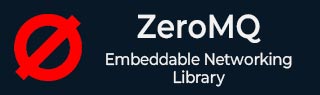
- ZeroMQ 教程
- ZeroMQ - 首页
- ZeroMQ - 概述
- ZeroMQ - 安装
- ZeroMQ - 特性
- ZeroMQ 消息传递
- ZeroMQ - 套接字类型
- ZeroMQ - 通信模式
- ZeroMQ - 传输协议
- ZeroMQ - 消息帧
- 扩展性和性能
- ZeroMQ - 负载均衡
- ZeroMQ - SMP
- ZeroMQ - 多线程
- ZeroMQ - 性能注意事项
- ZeroMQ 有用资源
- ZeroMQ - 有用资源
- ZeroMQ - 讨论
ZeroMQ - 安装设置
学习 ZeroMQ 的第一步是在您的系统上安装核心 ZeroMQ 库,并且根据您使用的编程语言,您需要安装相应的语言绑定以在代码中与 ZeroMQ 进行交互。
例如,如果您使用 Python 语言,则需要安装 **pyzmq**,它提供了 ZeroMQ 的 Python 绑定。
**ZeroMQ** 库的安装不依赖于操作系统。相反,它依赖于编程语言及其各自的绑定依赖项。因此,安装步骤在不同的操作系统上通常是类似的。
如何在 Java 中设置 ZeroMQ?
以下说明将解释如何在 Java 中安装和设置 ZeroMQ 库。要在 Java 中安装和使用 ZeroMQ (ØMQ),您需要使用 ZeroMQ 的 Java 绑定。最常用的绑定是 **JeroMQ**,它是 ZeroMQ 的纯 Java 实现。
以下是 Java 中安装 ZeroMQ 的分步指南:
步骤 1:在 Java 中创建一个 Maven 项目
要在 Java 中创建一个 Maven 项目,请打开您首选的 IDE(我们使用 Eclipse),点击左上角的 **文件** 菜单,并在显示的列表中选择 **Maven 项目**,如下面的图片所示:
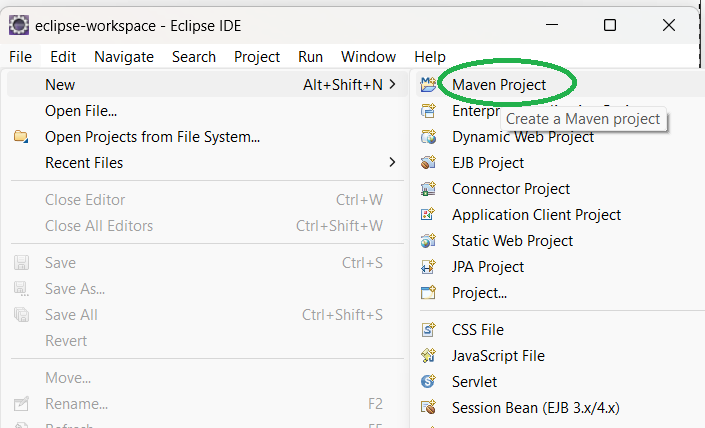
这将为您提供一个“新建 Maven 项目”窗口。在其中,点击 **下一步** 按钮。
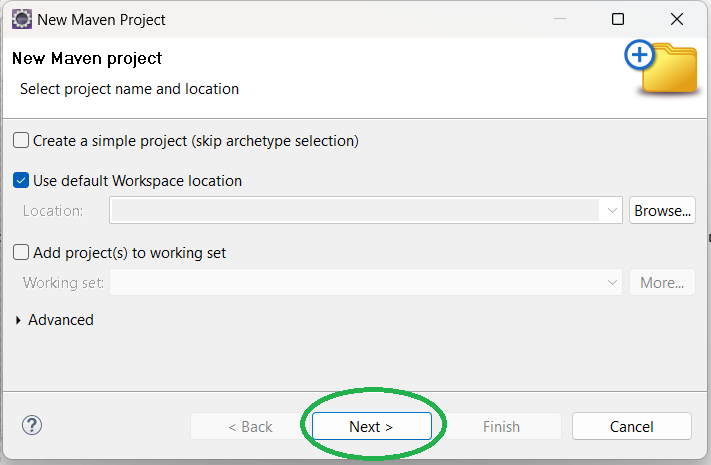
在下一个窗口中,在 **过滤器** 文本框中输入“Apache”,选择 **quickstart** 并点击 **下一步**。
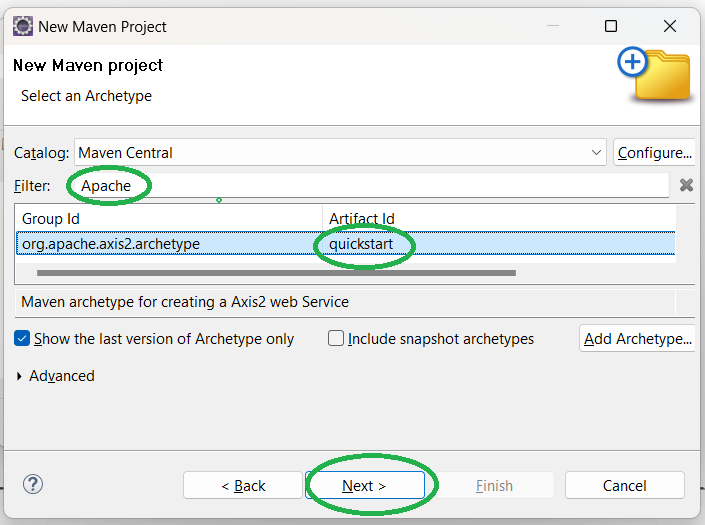
然后,在 **GroupId** 文本框中输入“com.zeromq”,在 **ArtifactId** 文本框中输入“zeromq”,然后点击 **完成**(您可以为“GroupId”和“ArtifactId”选择任何值)。
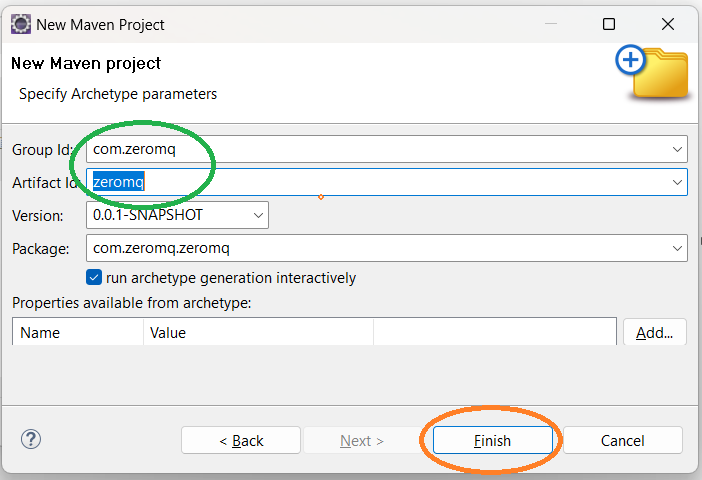
点击 **完成** 后,在您的终端中输入 'y' 并按 Enter。
步骤 2:添加 ZeroMQ 依赖项
在 Java 项目中安装 ZeroMQ 最简单的方法是使用 JeroMQ,它可以作为项目中的依赖项包含。
如果您使用 Maven 或 Gradle(两者都是 Java 开发工具)来管理您的项目,请将依赖项添加到您的 pom.xml 或 build.gradle 文件中。
<dependency> <groupId>org.zeromq</groupId> <artifactId>jeromq</artifactId> <version>0.5.3</version> </dependency> //for the latest SNAPSHOT --> <dependency> <groupId>org.zeromq</groupId> <artifactId>jeromq</artifactId> <version>0.6.0-SNAPSHOT</version> </dependency> //If you can't find the latest snapshot <repositories> <repository> <id>sonatype-nexus-snapshots</id> <url>https://oss.sonatype.org/content/repositories/snapshots</url> <releases> <enabled>false</enabled> </releases> <snapshots> <enabled>true</enabled> </snapshots> </repository> </repositories>
步骤 3:编写您的第一个 Java ZeroMQ 程序
将 JeroMQ 包含到您的项目中后,您就可以开始使用它了。以下是 Java 中基本的 ZeroMQ 发布者和订阅者的示例:
发布者
以下是“发布者”的基本示例:
import org.zeromq.ZMQ; import org.zeromq.ZMQ.Context; import org.zeromq.ZMQ.Socket; public class Publisher { public static void main(String[] args) { Context context = ZMQ.context(1); Socket socket = context.socket(ZMQ.PUB); socket.bind("tcp://*:5555"); while (true) { socket.send("Hello, subscribers!".getBytes(), 0); } } }
输出
//it will simply send the message to the receiver
订阅者
以下是“订阅者”示例:
import org.zeromq.ZMQ; import org.zeromq.ZMQ.Context; import org.zeromq.ZMQ.Socket; public class Subscriber { public static void main(String[] args) { Context context = ZMQ.context(1); Socket socket = context.socket(ZMQ.SUB); socket.connect("tcp://127.0.0.1:5555"); socket.subscribe("".getBytes()); // Subscribe to all messages while (true) { byte[] message = socket.recv(0); System.out.println("Received message: " + new String(message)); } } }
输出
上述程序显示了由“发布者”发送的所有接收到的消息:
Received message: Hello, subscribers! Received message: Hello, subscribers! Received message: Hello, subscribers! Received message: Hello, subscribers! Received message: Hello, subscribers! Received message: Hello, subscribers!
如何在 Python 中设置 ZeroMQ?
要下载并在 Python 中安装 ZeroMQ,我们需要下载与 ZeroMQ 绑定的 Python 库,即 **PyZMQ 库**,它是一个快速且轻量级的消息传递库。
PyZMQ 可与任何合理的 Python 版本(应大于或等于 3.7)以及 PyPy 一起使用。PyZMQ 用于在没有消息代理的情况下进行消息排队。
步骤 1:安装 PyZMQ
要安装 PyZMQ,我们可以使用 **pip**,这是安装 Python 包最常见的方法。以下是步骤:
打开终端和命令提示符
- **在 Windows 上**,按 **Windows + R**,键入 **cmd**,然后按 Enter。
- **在 Mac 或 Linux 上**,打开一个终端。
运行 pip install 命令
要安装 PyZMQ,请在您的终端或命令提示符中运行以下命令:
pip install pyzmq
上述命令将下载并安装 PyZMQ 和 ZeroMQ 库。
Downloading pyzmq-26.2.0-cp312-cp312-win_amd64.whl.metadata (6.2 kB) Downloading pyzmq-26.2.0-cp312-cp312-win_amd64.whl (637 kB) --------------------- 637.8/637.8 kB 5.8 MB/s eta 0:00:00 Installing collected packages: pyzmq Successfully installed pyzmq-26.2.0 [notice] A new release of pip is available: 24.0 -> 24.2 [notice] To update, run: python.exe -m pip install --upgrade pip
步骤 2:验证
要确认 PyZMQ 是否已正确安装,请打开 Python shell 并运行以下命令。如果它在没有任何错误的情况下打印出已安装的 ZeroMQ 和 PyZMQ 版本,则安装成功。
>>> import zmq >>> print(zmq.zmq_version()) 4.3.5 >>> print(zmq.pyzmq_version()) 26.2.0 >>>
步骤 3:编写您的第一个 Python ZeroMQ 程序
由于我们已经安装了 Python 消息传递库“PyZMQ”,因此我们可以使用 ZeroMQ 编写 Python 程序。
发布者
以下是“发布者”的基本示例:
import zmq import time # Create a ZeroMQ context context = zmq.Context() # Create a PUB socket socket = context.socket(zmq.PUB) socket.bind("tcp://*:5555") while True: # Send a message every second message = "Hello, ZeroMQ!" socket.send_string(message) print(f"Sent: {message}") time.sleep(1)
输出
Sent: Hello, ZeroMQ! Sent: Hello, ZeroMQ! Sent: Hello, ZeroMQ! Sent: Hello, ZeroMQ! Sent: Hello, ZeroMQ!
订阅者
以下是“订阅者”示例:
import zmq # Create a ZeroMQ context context = zmq.Context() # Create a SUB socket socket = context.socket(zmq.SUB) socket.connect("tcp://127.0.0.1:5555") # Subscribe to all messages (empty string as subscription) socket.setsockopt_string(zmq.SUBSCRIBE, "") while True: message = socket.recv_string() print(f"Received: {message}")
输出
Received: Hello, ZeroMQ! Received: Hello, ZeroMQ! Received: Hello, ZeroMQ! Received: Hello, ZeroMQ! Received: Hello, ZeroMQ!