使用Python Tkinter创建番茄工作法计时器
什么是番茄工作法?
番茄工作法是由弗朗切斯科·西里洛(Francesco Cirillo)在上世纪80年代末发明的。当时他还是一名大学生,在学习和完成作业时遇到了困难。感到不知所措的他,给自己设定了只专注学习10分钟的目标。受到这个挑战的启发,他发现了一个番茄形状的厨房计时器(意大利语为pomodoro),于是番茄工作法诞生了。在本教程中,我们将使用Python和Tkinter创建一个番茄工作法计时器。计时器将使用图形用户界面(GUI)创建,我们将使用Tkinter模块来创建GUI。
步骤和流程
步骤1 - 安装所需的库
在开始编码之前,我们需要确保已安装所有必需的库。在本教程中,我们将使用`time`和`tkinter`模块。这两个模块都预装在Python中,因此我们无需单独安装它们。
步骤2 - 创建GUI
现在我们有了所需的库,就可以开始创建GUI了。首先,我们需要创建一个新的Python文件并导入Tkinter模块。
import tkinter as tk import time from datetime import datetime, timedelta
接下来,我们需要创建一个Tkinter类的新的实例,并设置窗口标题和尺寸。
# Create a GUI window root = tk.Tk() root.title("Pomodoro Timer") # Set the window size and position window_width = 300 window_height = 150 screen_width = root.winfo_screenwidth() screen_height = root.winfo_screenheight() x_cordinate = int((screen_width / 2) - (window_width / 2)) y_cordinate = int((screen_height / 2) - (window_height / 2)) root.geometry("{}x{}+{}+{}".format(window_width, window_height, x_cordinate, y_cordinate))
这段代码创建一个新的窗口,标题为“番茄工作法计时器”,尺寸为300像素×150像素。
步骤3 - 添加标签和按钮
现在我们需要向我们创建的GUI窗口添加标签和按钮。我们将包括一个显示当前时间的标签,一个显示倒计时时间的标签,以及两个用于启动和停止计时器的按钮。
# Current Time Label current_time_label = tk.Label(text="", font=("Helvetica", 20)) current_time_label.pack() # Timer Label timer_label = tk.Label(text="", font=("Helvetica", 30)) timer_label.pack() # Start Button start_button = tk.Button(text="Start", font=("Helvetica", 10)) start_button.pack(side="left") # Stop Button stop_button = tk.Button(text="Stop", font=("Helvetica", 10)) stop_button.pack(side="right")
这段代码生成标签和按钮,并将它们放置在图形用户界面窗口中。目前,当前时间标签和计时器标签为空;稍后我们将用当前时间和计时器值填充它们。
步骤4 - 为按钮添加功能
现在我们已经有了标签和按钮,我们需要为启动和停止按钮添加功能。按下启动按钮时,计时器应该开始倒计时,并且当前时间标签应该反映当前时间。按下停止按钮时,计时器应该停止倒计时。
# Start Button Functionality def start_timer(): current_time_label.config(text=time.strftime("%H:%M:%S")) timer_label.config(text="25:00") start_button.config(state="disabled") stop_button.config(state="normal") # Stop Button Functionality def stop_timer(): timer_label.config(text="") start_button.config(state="normal") stop_button.config(state="disabled")
`start_timer`方法使用`time`模块的`strftime`函数更新当前时间标签的当前时间。它还将计时器标签更改为25分钟(番茄工作法的时间间隔长度),并禁用启动和停止按钮。`stop_timer`函数清除计时器标签,激活启动按钮,并禁用停止按钮。
步骤5 - 添加计时器功能
现在我们已经有了启动和停止按钮的功能,我们需要添加计时器功能。我们将使用`time`模块的`sleep`函数在每次倒计时之间暂停程序一秒钟。我们还将使用`datetime`模块的`timedelta`函数来计算剩余时间并更新计时器标签。
# Timer Functionality def countdown(minutes): seconds = minutes * 60 while seconds > 0: minutes, sec = divmod(seconds, 60) timer_label.config(text='{:02d}:{:02d}'.format(minutes, sec)) time.sleep(1) seconds -= 1 timer_label.config(text="Time's up!") start_button.config(state="normal") stop_button.config(state="disabled")
`countdown`函数将输入的分钟数转换为相应的秒数。如果还有剩余秒数,它将进入一个无限循环。在循环过程中,`divmod`函数用于确定剩余的分钟和秒数,`format`函数用于在计时器标签上显示该信息。每次执行倒计时时,程序都会休眠一秒钟。当倒计时接近零时,计时器标签将更改为“时间到!”,启动按钮被激活,停止按钮被禁用。
步骤6 - 将按钮连接到函数
最后一步是将启动和停止按钮连接到它们各自的函数。我们可以使用按钮的`command`参数来实现。
start_button.config(command=lambda: (start_timer(), countdown(25))) stop_button.config(command=stop_timer)
示例
现在我们可以运行程序并测试番茄工作法计时器。要运行程序,请将代码保存在一个以`.py`为扩展名的文件中,并使用Python解释器运行它。
# importing the essential libraries for our work import tkinter as tk import time from datetime import datetime, timedelta # Create a GUI window root = tk.Tk() root.title("Pomodoro Timer") # Set the window size and position window_width = 300 window_height = 150 screen_width = root.winfo_screenwidth() screen_height = root.winfo_screenheight() x_cordinate = int((screen_width / 2) - (window_width / 2)) y_cordinate = int((screen_height / 2) - (window_height / 2)) root.geometry("{}x{}+{}+{}".format(window_width, window_height, x_cordinate, y_cordinate)) # Current Time Label current_time_label = tk.Label(text="", font=("Helvetica", 20)) current_time_label.pack() # Timer Label timer_label = tk.Label(text="", font=("Helvetica", 30)) timer_label.pack() # Start Button start_button = tk.Button(text="Start", font=("Helvetica", 10)) start_button.pack(side="left") # Stop Button stop_button = tk.Button(text="Stop", font=("Helvetica", 10)) stop_button.pack(side="right") # This code snippet is used to understand the start Button Functionality def start_timer(): current_time_label.config(text=time.strftime("%H:%M:%S")) timer_label.config(text="25:00") start_button.config(state="disabled") stop_button.config(state="normal") # This code snippet is used to understand the stop Button Functionality def stop_timer(): timer_label.config(text="") start_button.config(state="normal") stop_button.config(state="disabled") # This code snippet is used to understand the timer Functionality def countdown(minutes): seconds = minutes * 60 while seconds > 0: minutes, sec = divmod(seconds, 60) timer_label.config(text='{:02d}:{:02d}'.format(minutes, sec)) time.sleep(1) seconds -= 1 timer_label.config(text="Time's up!") start_button.config(state="normal") stop_button.config(state="disabled") # this piece of code is used to connect the start and stop button to make it easier start_button.config(command=lambda: (start_timer(), countdown(25))) stop_button.config(command=stop_timer)
输出
在上框中,我们可以看到带有GUI的番茄工作法应用程序的输出,这可以用来创建一个。
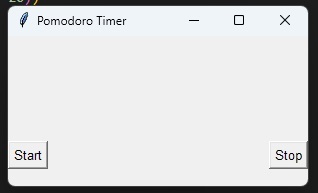
结论
按照本说明,你将能够使用Python和Tkinter创建你自己的番茄工作法计时器。我们使用Tkinter模块创建了一个图形用户界面(GUI)窗口,并用文本和控件元素填充它。为了开发一个在计时器标签上显示剩余时间的倒计时计时器,我们使用了`time`和`datetime`模块,并配置了启动和停止按钮的功能。最后,我们将按钮连接到它们预期的操作并运行了代码。如果你想在更短的时间内完成更多工作,那么这个番茄工作法计时器适合你。