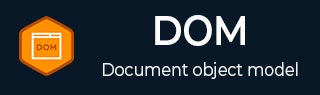
- XML DOM 基础
- XML DOM - 首页
- XML DOM - 概述
- XML DOM - 模型
- XML DOM - 节点
- XML DOM - 节点树
- XML DOM - 方法
- XML DOM - 加载
- XML DOM - 遍历
- XML DOM - 导航
- XML DOM - 访问
- XML DOM 操作
- XML DOM - 获取节点
- XML DOM - 设置节点
- XML DOM - 创建节点
- XML DOM - 添加节点
- XML DOM - 替换节点
- XML DOM - 删除节点
- XML DOM - 克隆节点
- XML DOM 对象
- DOM - 节点对象
- DOM - NodeList 对象
- DOM - NamedNodeMap 对象
- DOM - DOMImplementation
- DOM - DocumentType 对象
- DOM - ProcessingInstruction
- DOM - 实体对象
- DOM - 实体引用对象
- DOM - 符号对象
- DOM - 元素对象
- DOM - 属性对象
- DOM - CDATASection 对象
- DOM - 注释对象
- DOM - XMLHttpRequest 对象
- DOM - DOMException 对象
- XML DOM 有用资源
- XML DOM - 快速指南
- XML DOM - 有用资源
- XML DOM - 讨论
XML DOM - 导航
到目前为止,我们学习了 DOM 结构,如何加载和解析 XML DOM 对象以及遍历 DOM 对象。在这里,我们将了解如何在 DOM 对象中节点之间进行导航。XML DOM 包含节点的各种属性,这些属性可以帮助我们遍历节点,例如:
- parentNode
- childNodes
- firstChild
- lastChild
- nextSibling
- previousSibling
以下是节点树的示意图,显示了它与其他节点的关系。
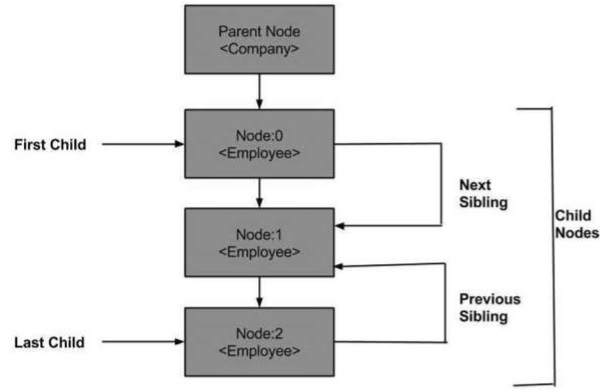
DOM - 父节点
此属性将父节点指定为节点对象。
示例
以下示例 (navigate_example.htm) 将 XML 文档 (node.xml) 解析为 XML DOM 对象。然后,通过子节点导航到父节点:
<!DOCTYPE html> <html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; var y = xmlDoc.getElementsByTagName("Employee")[0]; document.write(y.parentNode.nodeName); </script> </body> </html>
如上例所示,子节点 Employee 导航到其父节点。
执行
将此文件另存为服务器路径上的 navigate_example.html(此文件和 node.xml 应位于服务器上的同一路径)。在输出中,我们得到 Employee 的父节点,即 Company。
第一个子节点
此属性的类型为 Node,表示 NodeList 中存在的第一个子节点名称。
示例
以下示例 (first_node_example.htm) 将 XML 文档 (node.xml) 解析为 XML DOM 对象,然后导航到 DOM 对象中存在的第一个子节点。
<!DOCTYPE html> <html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_firstChild(p) { a = p.firstChild; while (a.nodeType != 1) { a = a.nextSibling; } return a; } var firstchild = get_firstChild(xmlDoc.getElementsByTagName("Employee")[0]); document.write(firstchild.nodeName); </script> </body> </html>
函数 get_firstChild(p) 用于避免空节点。它有助于从节点列表中获取 firstChild 元素。
x = get_firstChild(xmlDoc.getElementsByTagName("Employee")[0]) 获取标签名称为 Employee 的第一个子节点。
执行
将此文件另存为服务器路径上的 first_node_example.htm(此文件和 node.xml 应位于服务器上的同一路径)。在输出中,我们得到 Employee 的第一个子节点,即 FirstName。
最后一个子节点
此属性的类型为 Node,表示 NodeList 中存在的最后一个子节点名称。
示例
以下示例 (last_node_example.htm) 将 XML 文档 (node.xml) 解析为 XML DOM 对象,然后导航到 xml DOM 对象中存在的最后一个子节点。
<!DOCTYPE html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_lastChild(p) { a = p.lastChild; while (a.nodeType != 1){ a = a.previousSibling; } return a; } var lastchild = get_lastChild(xmlDoc.getElementsByTagName("Employee")[0]); document.write(lastchild.nodeName); </script> </body> </html>
执行
将此文件另存为服务器路径上的 last_node_example.htm(此文件和 node.xml 应位于服务器上的同一路径)。在输出中,我们得到 Employee 的最后一个子节点,即 Email。
下一个兄弟节点
此属性的类型为 Node,表示下一个子节点,即 NodeList 中存在的指定子元素的下一个兄弟节点。
示例
以下示例 (nextSibling_example.htm) 将 XML 文档 (node.xml) 解析为 XML DOM 对象,该对象立即导航到 xml 文档中存在的下一个节点。
<!DOCTYPE html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_nextSibling(p) { a = p.nextSibling; while (a.nodeType != 1) { a = a.nextSibling; } return a; } var nextsibling = get_nextSibling(xmlDoc.getElementsByTagName("FirstName")[0]); document.write(nextsibling.nodeName); </script> </body> </html>
执行
将此文件另存为服务器路径上的 nextSibling_example.htm(此文件和 node.xml 应位于服务器上的同一路径)。在输出中,我们得到 FirstName 的下一个兄弟节点,即 LastName。
上一个兄弟节点
此属性的类型为 Node,表示上一个子节点,即 NodeList 中存在的指定子元素的上一个兄弟节点。
示例
以下示例 (previoussibling_example.htm) 将 XML 文档 (node.xml) 解析为 XML DOM 对象,然后导航到 xml 文档中最后一个子节点之前的节点。
<!DOCTYPE html> <body> <script> if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","/dom/node.xml",false); xmlhttp.send(); xmlDoc = xmlhttp.responseXML; function get_previousSibling(p) { a = p.previousSibling; while (a.nodeType != 1) { a = a.previousSibling; } return a; } prevsibling = get_previousSibling(xmlDoc.getElementsByTagName("Email")[0]); document.write(prevsibling.nodeName); </script> </body> </html>
执行
将此文件另存为服务器路径上的 previoussibling_example.htm(此文件和 node.xml 应位于服务器上的同一路径)。在输出中,我们得到 Email 的上一个兄弟节点,即 ContactNo。