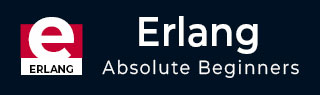
- Erlang 教程
- Erlang - 首页
- Erlang - 概述
- Erlang - 环境
- Erlang - 基本语法
- Erlang - Shell
- Erlang - 数据类型
- Erlang - 变量
- Erlang - 运算符
- Erlang - 循环
- Erlang - 决策
- Erlang - 函数
- Erlang - 模块
- Erlang - 递归
- Erlang - 数字
- Erlang - 字符串
- Erlang - 列表
- Erlang - 文件 I/O
- Erlang - 原子
- Erlang - 映射
- Erlang - 元组
- Erlang - 记录
- Erlang - 异常
- Erlang - 宏
- Erlang - 头文件
- Erlang - 预处理器
- Erlang - 模式匹配
- Erlang - 保卫
- Erlang - BIFS
- Erlang - 二进制
- Erlang - 函数
- Erlang - 进程
- Erlang - 邮件
- Erlang - 数据库
- Erlang - 端口
- Erlang - 分布式编程
- Erlang - OTP
- Erlang - 并发
- Erlang - 性能
- Erlang - 驱动
- Erlang - Web 编程
- Erlang 有用资源
- Erlang - 快速指南
- Erlang - 有用资源
- Erlang - 讨论
Erlang - 基本语法
为了理解 Erlang 的基本语法,让我们先看看一个简单的 **Hello World** 程序。
示例
% hello world program -module(helloworld). -export([start/0]). start() -> io:fwrite("Hello, world!\n").
关于以上程序需要注意以下几点:
% 符号用于在程序中添加注释。
module 语句就像在任何编程语言中添加命名空间一样。因此,在这里,我们提到此代码将是名为 **helloworld** 的模块的一部分。
export 函数用于定义程序中定义的任何函数都可以使用。我们正在定义一个名为 start 的函数,为了使用 start 函数,我们必须使用 export 语句。** /0 ** 表示我们的函数“start”接受 0 个参数。
我们最终定义了我们的 start 函数。在这里,我们使用了另一个名为 **io** 的模块,该模块包含 Erlang 中所有必需的输入输出函数。我们使用 **fwrite** 函数将“Hello World”输出到控制台。
以上程序的输出将是:
输出
Hello, world!
语句的一般形式
在 Erlang 中,您已经看到 Erlang 语言中使用了不同的符号。让我们回顾一下我们在简单的 Hello World 程序中学到的内容:
连字符符号 **(–)** 通常与模块、导入和导出语句一起使用。连字符符号用于根据需要赋予每个语句含义。因此,来自 Hello World 程序的示例如下所示:
-module(helloworld). -export([start/0]).
每个语句都用点 **(.)** 符号分隔。Erlang 中的每个语句都需要以这个分隔符结尾。来自 Hello World 程序的一个示例如下所示:
io:fwrite("Hello, world!\n").
斜杠 **(/)** 符号与函数一起使用,以定义函数接受的参数数量。
-export([start/0]).
模块
在 Erlang 中,所有代码都分为模块。模块由一系列属性和函数声明组成。它就像其他编程语言中的命名空间概念,用于逻辑上分离不同的代码单元。
定义模块
模块是用模块标识符定义的。一般语法和示例如下所示。
语法
-module(ModuleName)
**ModuleName** 必须与文件名(减去扩展名 **.erl**)相同。否则代码加载将无法按预期工作。
示例
-module(helloworld)
这些模块将在后续章节中详细介绍,这只是为了让您对如何定义模块有一个基本的了解。
Erlang 中的导入语句
在 Erlang 中,如果想要使用现有的 Erlang 模块的功能,可以使用 import 语句。导入语句的一般形式如下所示:
示例
-import (modulename, [functionname/parameter]).
其中,
**Modulename** - 这是需要导入的模块的名称。
**functionname/parameter** - 需要导入的模块中的函数。
让我们更改编写 Hello World 程序的方式以使用导入语句。示例如下所示:
示例
% hello world program -module(helloworld). -import(io,[fwrite/1]). -export([start/0]). start() -> fwrite("Hello, world!\n").
在上面的代码中,我们使用 import 关键字导入库“io”和特定的 **fwrite** 函数。因此,现在无论何时调用 fwrite 函数,我们都不必在任何地方都提及 **io** 模块名称。
Erlang 中的关键字
关键字是 Erlang 中的保留字,不应将其用于任何与预期用途不同的目的。以下是 Erlang 中的关键字列表。
after | and | andalso | band |
begin | bnot | bor | bsl |
bsr | bxor | case | catch |
cond | div | end | fun |
if | let | not | of |
or | orelse | receive | rem |
try | when | xor |
Erlang 中的注释
注释用于记录您的代码。单行注释通过在该行的任何位置使用 **%** 符号来识别。以下是一个示例:
示例
% hello world program -module(helloworld). % import function used to import the io module -import(io,[fwrite/1]). % export function used to ensure the start function can be accessed. -export([start/0]). start() -> fwrite("Hello, world!\n").