在Swift中获取iOS警告框TextField的输入值
通常,我们使用UIAlertController来显示带有关闭操作按钮的警告框。但是UIAlertController 提供了更多灵活性来向警告框添加UITextFields。让我们看看一些不同用例的示例。
当您向UIAlertController添加文本字段时,您可以获取在文本字段中输入的值。也可以添加多个文本字段。
在这个例子中,我们将使用以下步骤来获取iOS文本字段的输入值。
基本设置
在此步骤中,您将向控制器的视图添加一个按钮对象,以便通过单击它来呈现警告控制器。这是基本设置代码:
示例
import UIKit class TestController: UIViewController { private let clickButton = UIButton() override func viewDidLoad() { super.viewDidLoad() initialSetup() } private func initialSetup() { // basic setup view.backgroundColor = .white navigationItem.title = "UIAlertController" // button customization clickButton.backgroundColor = .darkGray clickButton.setTitle("Show Alert", for: .normal) clickButton.setTitleColor(.white, for: .normal) clickButton.layer.cornerRadius = 8 clickButton.addTarget(self, action: #selector(handleClickButtonTapped), for: .touchUpInside) // adding constraints to the button view.addSubview(clickButton) clickButton.translatesAutoresizingMaskIntoConstraints = false clickButton.heightAnchor.constraint(equalToConstant: 50).isActive = true clickButton.widthAnchor.constraint(equalToConstant: 250).isActive = true clickButton.centerXAnchor.constraint(equalTo: view.centerXAnchor).isActive = true clickButton.centerYAnchor.constraint(equalTo: view.centerYAnchor).isActive = true } @objc private func handleClickButtonTapped() { } }
输出
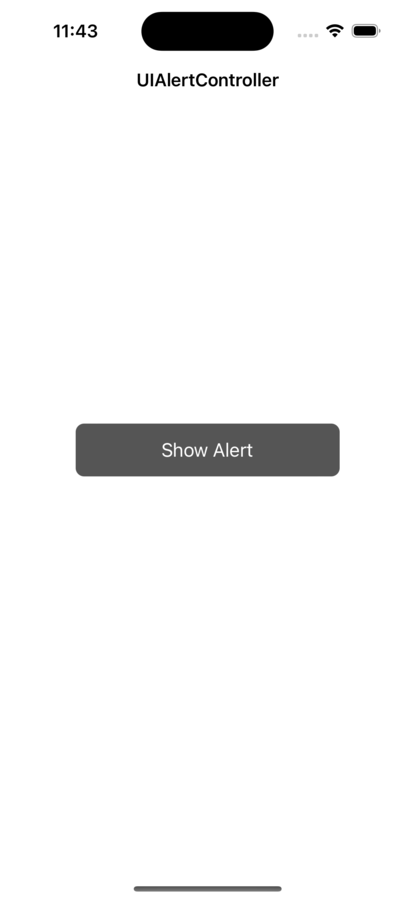
在上面的代码中,我们添加了一个带有目标动作方法的按钮。在下一步中,我们将实现handleClickButtonTapped方法来显示警告。
显示基本警告
在此步骤中,我们将显示一个带有标题和消息的简单警告。此外,我们还将添加一些操作来关闭警告。
示例
@objc private func handleClickButtonTapped() { // creating an alert controller object let alertController = UIAlertController(title: "Login", message: "Are you sure that you want to login?", preferredStyle: .alert) // adding actions to alert alertController.addAction(UIAlertAction(title: "Yes", style: .default)) alertController.addAction(UIAlertAction(title: "Not now", style: .default)) // present the alert on the screen self.present(alertController, animated: true) }
输出
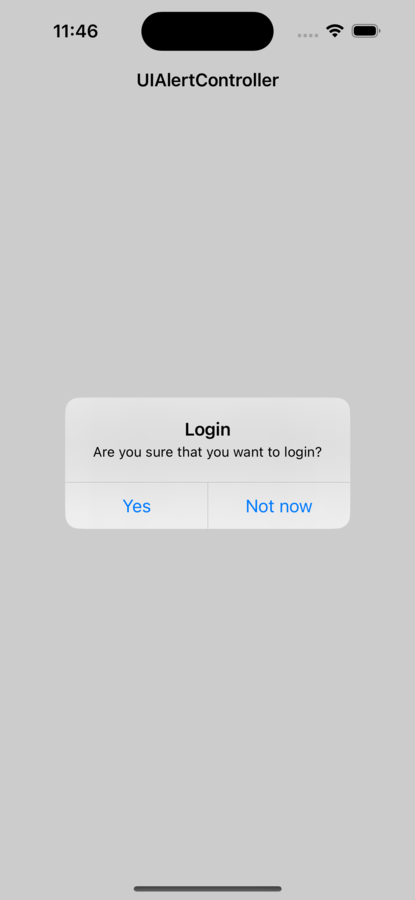
输入电子邮件地址
在此步骤中,我们将添加一个文本字段来输入电子邮件地址并获取其值。代码如下:
示例
@objc private func handleClickButtonTapped() { // creating an alert controller object let alertController = UIAlertController(title: "Enter Email", message: "Please enter a valid email address.", preferredStyle: .alert) // adding a textField for an email address alertController.addTextField { textField in // configure the text field textField.placeholder = "Email Address" textField.keyboardType = .emailAddress textField.autocorrectionType = .no } // creating OK action let okAction = UIAlertAction(title: "OK", style: .default) { action in guard let textField = alertController.textFields?.first, let text = textField.text else { print("No value has been entered in email address") return } print("Entered email address value: \(text)") } // creating a Cancel action let cancelAction = UIAlertAction(title: "Cancel", style: .cancel, handler: nil) // adding actions to alert alertController.addAction(okAction) alertController.addAction(cancelAction) // showing the alert present(alertController, animated: true, completion: nil) }
输出
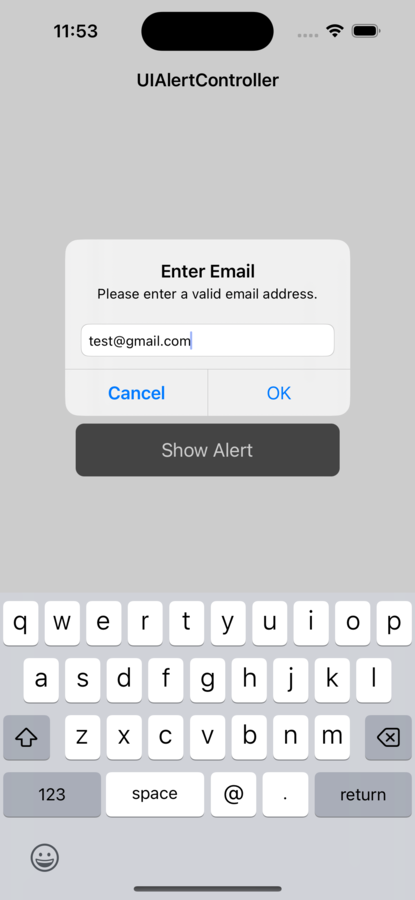
这是在控制台上打印的输出
Entered email address value: [email protected]
在这个例子中,我们创建了一个带有文本字段和两个操作(确定和取消)的警告控制器。当用户点击“确定”按钮时,将调用处理程序块,我们可以使用alertController.textFields?.first?.text访问文本字段的值。然后,我们使用文本的值来执行我们需要对输入执行的任何操作。
添加多个文本字段
在此步骤中,我们将向警告框添加两个文本字段。第一个文本字段用于姓名,第二个文本字段用于电子邮件地址。代码如下:
示例
@objc private func handleClickButtonTapped() { // creating an alert controller object let alertController = UIAlertController(title: "Enter Details", message: "Please enter your full name & a valid email address.", preferredStyle: .alert) // adding a textField for name alertController.addTextField { textField in textField.placeholder = "Full Name" textField.autocorrectionType = .no textField.autocapitalizationType = .words } // adding a textField for email address alertController.addTextField { textField in textField.placeholder = "Email Address" textField.keyboardType = .emailAddress textField.autocorrectionType = .no } // creating OK action let okAction = UIAlertAction(title: "OK", style: .default) { action in guard let nameTextField = alertController.textFields?[0], let emailTextField = alertController.textFields?[1], let name = nameTextField.text, let email = emailTextField.text else { return } print("Entered name: \(name) and email address: \(email)") } // creating Cancel action let cancelAction = UIAlertAction(title: "Cancel", style: .cancel, handler: nil) // adding actions to alert alertController.addAction(okAction) alertController.addAction(cancelAction) // showing the alert present(alertController, animated: true, completion: nil) }
输出
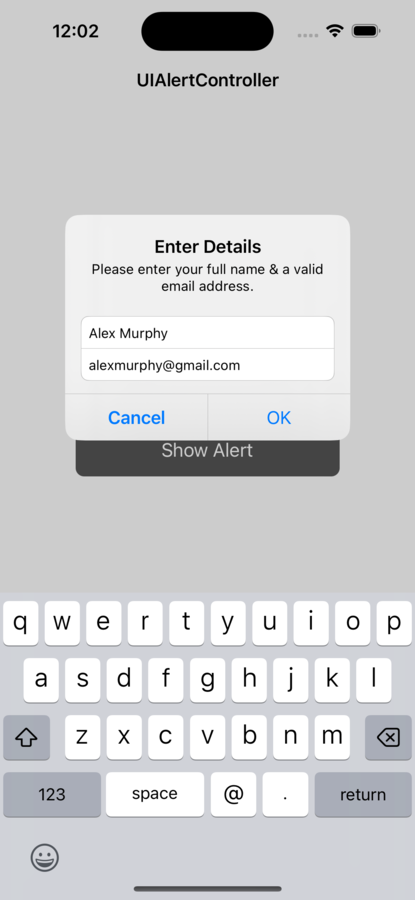
这是在控制台上打印的输出
Entered name: Alex Murphy and email address: [email protected]
在这个例子中,我们的警告框中有两个文本字段,我们使用它们在textFields数组中的相应索引来访问它们的值。
结论
总而言之,使用Swift在iOS警告框中从TextField获取输入数据非常简单。还有其他方法可以调整输入,例如添加更多文本字段、启用安全文本输入或将其限制为仅数字。您可以使用UIAlertController的处理程序参数检索文本字段的值。您可以使用这些方法为您的iOS应用程序设计适应性和吸引人的用户界面。