如何在 Tkinter 画布中为子字符串着色?
Tkinter 是 Python 的标准 GUI 工具包,提供了一套多功能的工具来创建图形用户界面。GUI 应用程序的一个常见需求是在文本块中突出显示或着色特定的子字符串。本文探讨了如何在 Tkinter 的 Canvas 小部件中实现此功能,使开发人员能够创建更具视觉吸引力和交互性的用户界面。
了解 Tkinter 画布
Tkinter 提供了各种小部件来构建 GUI 应用程序,而 Canvas 小部件对于绘制形状、图像和文本特别有用。虽然 Text 小部件通常用于多行文本,但 Canvas 小部件在自定义绘制和图形元素方面提供了更大的灵活性。
子字符串着色的挑战
默认情况下,Tkinter 没有提供直接的方法来为文本块中的特定子字符串着色。但是,通过采用涉及标签和配置的策略方法,开发人员可以有效地完成此任务。挑战在于遍历文本、识别子字符串并在不影响整个文本内容的情况下应用颜色。
在 Tkinter 中使用标签
Tkinter 中的标签是一种强大的机制,用于将格式或样式应用于小部件中特定范围的文本。tag_configure 方法允许开发人员定义标签的属性,例如字体、颜色或其他视觉属性。另一方面,tag_add 方法用于将标签与特定范围的文本关联。
示例实现
让我们深入研究一个实际示例,以了解如何在 Tkinter Canvas 小部件中为特定子字符串着色。
import tkinter as tk # Function to color a substring within a Text widget def color_substring(widget, substring, color): # Start searching from the beginning of the text start_index = "1.0" # Loop until there are no more occurrences of the substring while True: # Search for the substring within the text start_index = widget.search(substring, start_index, stopindex=tk.END) # If no more occurrences are found, exit the loop if not start_index: break # Calculate the end index of the substring end_index = widget.index(f"{start_index}+{len(substring)}c") # Add a tag with the specified color to the identified substring widget.tag_add(color, start_index, end_index) # Update the start index for the next iteration start_index = end_index # Create the main Tkinter window root = tk.Tk() root.title("Color Substring Example") root.geometry("720x250") # Create a Text widget for displaying text text_widget = tk.Text(root, width=40, height=10) text_widget.pack() # Define a tag named "red" with a specific color # foreground color set to red text_widget.tag_configure("red", foreground="red") # Insert some text into the Text widget text_widget.insert(tk.END, "This is a sample text with a colored substring.") # Color the substring "colored" with the tag "red" color_substring(text_widget, "colored", "red") # Start the Tkinter event loop root.mainloop()
此示例使用名为 color_substring 的函数,该函数将 Tkinter Text 小部件、子字符串和颜色作为参数。然后,它遍历文本,识别指定子字符串的出现,并将带有给定颜色的标签应用于每次出现。
输出
运行代码后,您将获得以下输出窗口 -
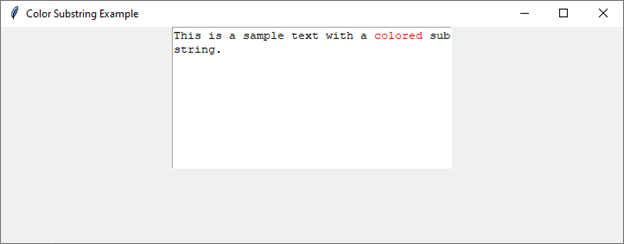
自定义实现
开发人员可以自定义此实现以满足其特定需求。例如,可以通过修改 tag_configure 方法来调整颜色、字体和其他样式属性。此外,可以根据应用程序的要求调整搜索逻辑以执行区分大小写或不区分大小写的搜索。
处理动态文本
在实际应用程序中,文本内容通常是动态的,并且可能需要动态地为子字符串着色。为了解决这个问题,开发人员可以将着色逻辑集成到事件处理程序或更新文本内容的函数中。这确保了每次文本更改时都会应用着色,从而提供无缝的用户体验。
增强功能和最佳实践
虽然提供的示例是一个基本说明,但开发人员可以根据最佳实践增强和优化实现。以下是一些注意事项 -
错误处理 - 整合错误处理机制以优雅地处理找不到子字符串或出现意外问题的情况。
性能优化 - 根据文本的大小,可能需要优化实现以获得更好的性能。考虑实施诸如缓存或根据文本可见部分限制搜索范围等技术。
用户交互 - 扩展功能以允许用户交互,例如在鼠标悬停时突出显示子字符串或提供颜色选择器以进行动态用户自定义。
与样式框架集成 - 对于更复杂的样式要求,请考虑将解决方案与提供高级文本格式选项的样式框架或库集成。
结论
在 Tkinter Canvas 小部件中为子字符串着色为 GUI 应用程序添加了一个宝贵的维度,增强了视觉吸引力和用户交互。