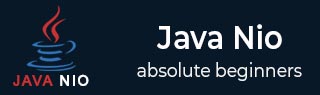
- Java NIO 教程
- Java NIO - 主页
- Java NIO - 概述
- Java NIO - 环境设置
- Java NIO 和 JAVA IO 对比
- Java NIO - 通道
- Java NIO - 文件通道
- Java NIO - 数据报通道
- Java NIO - 套接字通道
- Java NIO - 服务器套接字通道
- Java NIO - 分散
- Java NIO - 聚集
- Java NIO - 缓冲区
- Java NIO - 选择器
- Java NIO - 管道
- Java NIO - 路径
- Java NIO - 文件
- Java NIO - 异步文件通道
- Java NIO - 字符集
- Java NIO - 文件锁
- Java NIO 实用资源
- Java NIO - 快速指南
- Java NIO - 实用资源
- Java NIO - 讨论
Java NIO - 文件通道
描述
如前所述,Java NIO 通道中的 FileChannel 实现被引入以访问文件的元数据属性,包括创建、修改、大小等。除此之外,File 通道是多线程的,这又使得 Java NIO 比 Java IO 效率更高。
一般来说,我们可以说 FileChannel 是一个连接到文件的通道,你可以通过它从文件中读取数据和向文件中写入数据。FileChannel 的另一个重要特点是它不能被设置为非阻塞模式,并且始终以阻塞模式运行。
我们不能直接获取文件通道对象,文件通道对象可以通过以下方式获取 −
getChannel() − 在任何一个 FileInputStream、FileOutputStream 或 RandomAccessFile 上的方法。
open() − File 通道的方法,它默认打开通道。
File 通道的对象类型取决于从对象创建中调用的类类型,即如果对象是通过调用 FileInputStream 的 getchannel 方法创建的,则 File 通道将被打开用于读取,并且会抛出 NonWritableChannelException 以尝试写入它。
示例
以下示例展示了如何从 Java NIO FileChannel 中读取和写入数据。
以下示例从位于 C:/Test/temp.txt 的文本文件中读取,并将其内容打印到控制台。
temp.txt
Hello World!
FileChannelDemo.java
import java.io.IOException; import java.io.RandomAccessFile; import java.nio.ByteBuffer; import java.nio.channels.FileChannel; import java.nio.charset.Charset; import java.nio.file.Path; import java.nio.file.Paths; import java.nio.file.StandardOpenOption; import java.util.HashSet; import java.util.Set; public class FileChannelDemo { public static void main(String args[]) throws IOException { //append the content to existing file writeFileChannel(ByteBuffer.wrap("Welcome to TutorialsPoint".getBytes())); //read the file readFileChannel(); } public static void readFileChannel() throws IOException { RandomAccessFile randomAccessFile = new RandomAccessFile("C:/Test/temp.txt", "rw"); FileChannel fileChannel = randomAccessFile.getChannel(); ByteBuffer byteBuffer = ByteBuffer.allocate(512); Charset charset = Charset.forName("US-ASCII"); while (fileChannel.read(byteBuffer) > 0) { byteBuffer.rewind(); System.out.print(charset.decode(byteBuffer)); byteBuffer.flip(); } fileChannel.close(); randomAccessFile.close(); } public static void writeFileChannel(ByteBuffer byteBuffer)throws IOException { Set<StandardOpenOption> options = new HashSet<>(); options.add(StandardOpenOption.CREATE); options.add(StandardOpenOption.APPEND); Path path = Paths.get("C:/Test/temp.txt"); FileChannel fileChannel = FileChannel.open(path, options); fileChannel.write(byteBuffer); fileChannel.close(); } }
输出
Hello World! Welcome to TutorialsPoint
广告