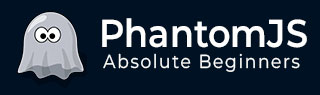
- 文件系统模块
- PhantomJS - 属性
- PhantomJS - 方法
- 系统模块
- PhantomJS - 属性
- Web 服务器模块
- PhantomJS - 属性
- PhantomJS - 方法
- 其他
- 命令行界面
- PhantomJS - 屏幕截图
- PhantomJS - 页面自动化
- PhantomJS - 网络监控
- PhantomJS - 测试
- PhantomJS - REPL
- PhantomJS - 示例
- PhantomJS 有用资源
- PhantomJS - 快速指南
- PhantomJS - 有用资源
- PhantomJS - 讨论
PhantomJS - 方法
PhantomJS 是一个无需浏览器即可执行 JavaScript 的平台。为此,它使用了以下方法,这些方法有助于添加 Cookie、删除、清除、退出脚本、注入 JS 等。
本章将详细讨论这些 PhantomJS 方法及其语法。类似的方法,即 **addcookie、injectjs** 存在于 webpage 模块中,将在后续章节中讨论。
PhantomJS 提供以下方法,可以帮助我们在没有浏览器的情况下执行 JavaScript:
- addCookie
- clearCookie
- deleteCookie
- exit
- injectJs
现在让我们用示例详细了解这些方法。
addCookie
addcookie 方法用于添加 Cookie 并将其存储在数据中。这与浏览器存储 Cookie 的方式类似。它接受一个参数,该参数是一个包含所有 Cookie 属性的对象,其语法如下所示:
语法
其语法如下:
phantom.addCookie ({ "name" : "cookie_name", "value" : "cookie_value", "domain" : "localhost" });
name、value 和 domain 是必须添加到 addcookie 函数的属性。如果 Cookie 对象中缺少任何这些属性,则此方法将失败。
**name** - 指定 Cookie 的名称。
**value** - 指定要使用的 Cookie 的值。
**domain** - 将应用 Cookie 的域名。
示例
这是一个 **addcookie** 方法的示例。
var page = require('webpage').create(),url = 'https://127.0.0.1/tasks/a.html'; page.open(url, function(status) { if (status === 'success') { phantom.addCookie({ //add name cookie1 with value = 1 name: 'cookie1', value: '1', domain: 'localhost' }); phantom.addCookie({ // add cookie2 with value 2 name: 'cookie2', value: '2', domain: 'localhost' }); phantom.addCookie({ // add cookie3 with value 3 name: 'cookie3', value: '3', domain: 'localhost' }); console.log('Added 3 cookies'); console.log('Total cookies :'+phantom.cookies.length); // will output the total cookies added to the url. } else { console.error('Cannot open file'); phantom.exit(1); } });
示例
a.html
<html> <head> <title>Welcome to phantomjs test page</title> </head> <body> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> </body> </html>
上述程序生成以下**输出**。
Added 3 cookies Total cookies :3
代码注释不言自明。
clearCookies
此方法允许删除所有 Cookie。
语法
其语法如下:
phantom.clearCookies();
此概念类似于通过选择浏览器菜单中的选项来删除浏览器 Cookie。
示例
这是一个 **clearCookies** 方法的示例。
var page = require('webpage').create(),url = 'https://127.0.0.1/tasks/a.html'; page.open(url, function(status) { if (status === 'success') { phantom.addCookie({ //add name cookie1 with value = 1 name: 'cookie1', value: '1', domain: 'localhost' }); phantom.addCookie({ // add cookie2 with value 2 name: 'cookie2', value: '2', domain: 'localhost' }); phantom.addCookie({ // add cookie3 with value 3 name: 'cookie3', value: '3', domain: 'localhost' }); console.log('Added 3 cookies'); console.log('Total cookies :'+phantom.cookies.length); phantom.clearCookies(); console.log( 'After clearcookies method total cookies :' +phantom.cookies.length); phantom.exit(); } else { console.error('Cannot open file'); phantom.exit(1); } });
a.html
<html> <head> <title>Welcome to phantomjs test page</title> </head> <body> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> </body> </html>
上述程序生成以下**输出**。
Added 3 cookies Total cookies :3 After clearcookies method total cookies :0
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
deleteCookie
删除 **CookieJar** 中任何具有与 cookieName 匹配的 'name' 属性的 Cookie。如果成功删除,则返回 **true**;否则返回 **false**。
语法
其语法如下:
phantom.deleteCookie(cookiename);
让我们通过一个示例来了解 **addcookie、clearcookies** 和 **deletecookie**。
示例
这是一个演示 deleteCookie 方法用法的示例:
文件:cookie.js
var page = require('webpage').create(),url = 'https://127.0.0.1/tasks/a.html'; page.open(url, function(status) { if (status === 'success') { phantom.addCookie({ //add name cookie1 with value = 1 name: 'cookie1', value: '1', domain: 'localhost' }); phantom.addCookie({ // add cookie2 with value 2 name: 'cookie2', value: '2', domain: 'localhost' }); phantom.addCookie({ // add cookie3 with value 3 name: 'cookie3', value: '3', domain: 'localhost' }); console.log('Added 3 cookies'); console.log('Total cookies :'+phantom.cookies.length); //will output the total cookies added to the url. console.log("Deleting cookie2"); phantom.deleteCookie('cookie2'); console.log('Total cookies :'+phantom.cookies.length); phantom.clearCookies(); console.log( 'After clearcookies method total cookies :' +phantom.cookies.length); phantom.exit(); } else { console.error('Cannot open file'); phantom.exit(1); } });
上述程序生成以下**输出**。
phantomjs cookie.js Added 3 cookies Total cookies :3 Deleting cookie2 Total cookies :2 After clearcookies method total cookies :0
exit
phantom.exit 方法将退出它启动的脚本。它使用提到的返回值退出程序。如果没有传递值,则返回 **‘0’**。
语法
其语法如下:
phantom.exit(value);
如果您不添加 **phantom.exit**,则命令行会假设执行仍在进行中,并且不会完成。
示例
让我们来看一个示例,以了解 **exit** 方法的用法。
console.log('Welcome to phantomJs'); // outputs Welcome to phantomJS var a = 1; if (a === 1) { console.log('Exit 1'); //outputs Exit 1 phantom.exit(); // Code exits. } else { console.log('Exit 2'); phantom.exit(1); }
上述程序生成以下**输出**。
phantomjs exit.js
Welcome to phantomJs Exit 1
phantom.exit 之后的所有代码段都不会执行,因为 phantom.exit 是结束脚本的方法。
injectJs
injectJs 用于在 phantom 中添加 **addtionaljs** 文件。如果当前 **directory librarypath** 中找不到该文件,则 phantom 属性 (phantom.libraryPath) 将用作跟踪路径的其他位置。如果文件添加成功,则返回 **true**;否则返回 **false**,表示失败,因为它无法找到该文件。
语法
其语法如下:
phantom.injectJs(filename);
示例
让我们来看下面的示例,以了解 **injectJs** 的用法。
文件名:inject.js
console.log(“Added file”);
文件名:addfile.js
var addfile = injectJs(inject.js); console.log(addfile); phantom.exit();
输出
**命令** - C:\phantomjs\bin>phantomjs addfile.js
Added file // coming from inject.js true
在上面的示例中,**addfile.js** 使用 injectJs 调用文件 **inject.js**。当您执行 addfile.js 时,inject.js 中的 console.log 将显示在输出中。它还为 addfile 变量显示 true,因为 inject.js 文件已成功添加。