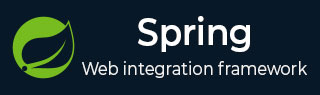
- Spring 核心基础
- Spring - 首页
- Spring - 概述
- Spring - 架构
- Spring - 环境搭建
- Spring - Hello World 示例
- Spring - IoC 容器
- Spring - Bean 定义
- Spring - Bean 作用域
- Spring - Bean 生命周期
- Spring - Bean 后处理器
- Spring - Bean 定义继承
- Spring - 依赖注入
- Spring - 注入内部 Bean
- Spring - 注入集合
- Spring - Bean 自动装配
- 基于注解的配置
- Spring - 基于 Java 的配置
- Spring - Spring 中的事件处理
- Spring - Spring 中的自定义事件
- Spring - 使用 Spring 框架进行 AOP
- Spring - JDBC 框架
- Spring - 事务管理
- Spring - Web MVC 框架
- Spring - 使用 Log4J 进行日志记录
- Spring 问题与解答
- Spring - 问题与解答
- Spring 有用资源
- Spring - 快速指南
- Spring - 有用资源
- Spring - 讨论
基于 AspectJ 的 Spring AOP
@AspectJ 指的是一种将方面声明为使用 Java 5 注解进行注解的常规 Java 类的方法。通过在基于 XML Schema 的配置文件中包含以下元素,可以启用 @AspectJ 支持。
<aop:aspectj-autoproxy/>
你还需要在应用程序的类路径中包含以下 AspectJ 库。这些库可在 AspectJ 安装的“lib”目录中找到,或者你可以从互联网上下载它们。
- aspectjrt.jar
- aspectjweaver.jar
- aspectj.jar
- aopalliance.jar
声明一个方面
方面类就像任何其他普通 Bean 一样,可以像任何其他类一样拥有方法和字段,只不过它们将使用 @Aspect 注解,如下所示:
package org.xyz; import org.aspectj.lang.annotation.Aspect; @Aspect public class AspectModule { }
它们将像任何其他 Bean 一样在 XML 中进行配置,如下所示:
<bean id = "myAspect" class = "org.xyz.AspectModule"> <!-- configure properties of aspect here as normal --> </bean>
声明切点
一个切点有助于确定要与不同通知一起执行的感兴趣的连接点(即方法)。在使用基于 @AspectJ 的配置时,切点声明包含两个部分:
一个切点表达式,用于精确确定我们感兴趣的方法执行。
一个切点签名,包含一个名称和任意数量的参数。方法的实际主体无关紧要,实际上应该为空。
以下示例定义了一个名为“businessService”的切点,该切点将匹配 com.xyz.myapp.service 包下类中每个方法的执行:
import org.aspectj.lang.annotation.Pointcut; @Pointcut("execution(* com.xyz.myapp.service.*.*(..))") // expression private void businessService() {} // signature
以下示例定义了一个名为“getname”的切点,该切点将匹配 com.tutorialspoint 包下 Student 类中 getName() 方法的执行:
import org.aspectj.lang.annotation.Pointcut; @Pointcut("execution(* com.tutorialspoint.Student.getName(..))") private void getname() {}
声明通知
你可以使用 @{ADVICE-NAME} 注解声明任何五种通知,如代码片段所示。这假设你已经定义了一个切点签名方法 businessService():
@Before("businessService()") public void doBeforeTask(){ ... } @After("businessService()") public void doAfterTask(){ ... } @AfterReturning(pointcut = "businessService()", returning = "retVal") public void doAfterReturnningTask(Object retVal) { // you can intercept retVal here. ... } @AfterThrowing(pointcut = "businessService()", throwing = "ex") public void doAfterThrowingTask(Exception ex) { // you can intercept thrown exception here. ... } @Around("businessService()") public void doAroundTask(){ ... }
你可以为任何通知内联定义切点。以下是如何为 before 通知定义内联切点的示例:
@Before("execution(* com.xyz.myapp.service.*.*(..))") public doBeforeTask(){ ... }
基于 @AspectJ 的 AOP 示例
为了理解上述与基于 @AspectJ 的 AOP 相关的概念,让我们编写一个示例,该示例将实现一些通知。为了使用一些通知编写我们的示例,让我们准备一个可用的 Eclipse IDE,并采取以下步骤创建一个 Spring 应用程序:
步骤 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个com.tutorialspoint包。 |
2 | 如Spring Hello World 示例章节中所述,使用添加外部 JAR选项添加所需的 Spring 库。 |
3 | 在项目中添加 Spring AOP 特定的库aspectjrt.jar、aspectjweaver.jar和aspectj.jar。 |
4 | 在com.tutorialspoint包下创建 Java 类Logging、Student和MainApp。 |
5 | 在src文件夹下创建 Bean 配置文件Beans.xml。 |
6 | 最后一步是创建所有 Java 文件和 Bean 配置文件的内容,并按如下所述运行应用程序。 |
以下是Logging.java文件的内容。这实际上是一个方面模块的示例,它定义了要在各个点调用的方法。
package com.tutorialspoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; import org.aspectj.lang.annotation.Before; import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.AfterThrowing; import org.aspectj.lang.annotation.AfterReturning; import org.aspectj.lang.annotation.Around; @Aspect public class Logging { /** Following is the definition for a pointcut to select * all the methods available. So advice will be called * for all the methods. */ @Pointcut("execution(* com.tutorialspoint.*.*(..))") private void selectAll(){} /** * This is the method which I would like to execute * before a selected method execution. */ @Before("selectAll()") public void beforeAdvice(){ System.out.println("Going to setup student profile."); } /** * This is the method which I would like to execute * after a selected method execution. */ @After("selectAll()") public void afterAdvice(){ System.out.println("Student profile has been setup."); } /** * This is the method which I would like to execute * when any method returns. */ @AfterReturning(pointcut = "selectAll()", returning = "retVal") public void afterReturningAdvice(Object retVal){ System.out.println("Returning:" + retVal.toString() ); } /** * This is the method which I would like to execute * if there is an exception raised by any method. */ @AfterThrowing(pointcut = "selectAll()", throwing = "ex") public void AfterThrowingAdvice(IllegalArgumentException ex){ System.out.println("There has been an exception: " + ex.toString()); } }
以下是Student.java文件的内容
package com.tutorialspoint; public class Student { private Integer age; private String name; public void setAge(Integer age) { this.age = age; } public Integer getAge() { System.out.println("Age : " + age ); return age; } public void setName(String name) { this.name = name; } public String getName() { System.out.println("Name : " + name ); return name; } public void printThrowException(){ System.out.println("Exception raised"); throw new IllegalArgumentException(); } }
以下是MainApp.java文件的内容
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); Student student = (Student) context.getBean("student"); student.getName(); student.getAge(); student.printThrowException(); } }
以下是配置文件Beans.xml
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:aop = "http://www.springframework.org/schema/aop" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd "> <aop:aspectj-autoproxy/> <!-- Definition for student bean --> <bean id = "student" class = "com.tutorialspoint.Student"> <property name = "name" value = "Zara" /> <property name = "age" value = "11"/> </bean> <!-- Definition for logging aspect --> <bean id = "logging" class = "com.tutorialspoint.Logging"/> </beans>
创建源文件和 Bean 配置文件后,让我们运行应用程序。如果你的应用程序一切正常,它将打印以下消息:
Going to setup student profile. Name : Zara Student profile has been setup. Returning:Zara Going to setup student profile. Age : 11 Student profile has been setup. Returning:11 Going to setup student profile. Exception raised Student profile has been setup. There has been an exception: java.lang.IllegalArgumentException ..... other exception content