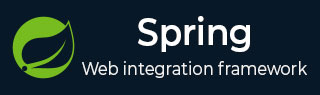
- Spring核心基础
- Spring - 首页
- Spring - 概述
- Spring - 架构
- Spring - 环境设置
- Spring - HelloWorld示例
- Spring - IoC容器
- Spring - Bean定义
- Spring - Bean作用域
- Spring - Bean生命周期
- Spring - Bean后处理器
- Spring - Bean定义继承
- Spring - 依赖注入
- Spring - 注入内部Bean
- Spring - 注入集合
- Spring - Bean自动装配
- 基于注解的配置
- Spring - 基于Java的配置
- Spring - Spring中的事件处理
- Spring - Spring中的自定义事件
- Spring - 使用Spring框架的AOP
- Spring - JDBC框架
- Spring - 事务管理
- Spring - Web MVC框架
- Spring - 使用Log4J进行日志记录
- Spring问答
- Spring - 问答
- Spring有用资源
- Spring - 快速指南
- Spring - 有用资源
- Spring - 讨论
Spring中的事件处理
在所有章节中,您都看到了Spring的核心是ApplicationContext,它管理Bean的完整生命周期。ApplicationContext在加载Bean时发布某些类型的事件。例如,当上下文启动时发布ContextStartedEvent,当上下文停止时发布ContextStoppedEvent。
ApplicationContext中的事件处理是通过ApplicationEvent类和ApplicationListener接口提供的。因此,如果一个Bean实现了ApplicationListener,那么每次将ApplicationEvent发布到ApplicationContext时,都会通知该Bean。
Spring提供以下标准事件:
序号 | Spring内置事件及描述 |
---|---|
1 |
ContextRefreshedEvent 当ApplicationContext被初始化或刷新时发布此事件。这也可以使用ConfigurableApplicationContext接口上的refresh()方法触发。 |
2 |
ContextStartedEvent 使用ConfigurableApplicationContext接口上的start()方法启动ApplicationContext时发布此事件。收到此事件后,您可以轮询数据库或重新启动任何已停止的应用程序。 |
3 |
ContextStoppedEvent 使用ConfigurableApplicationContext接口上的stop()方法停止ApplicationContext时发布此事件。收到此事件后,您可以执行必要的清理工作。 |
4 |
ContextClosedEvent 使用ConfigurableApplicationContext接口上的close()方法关闭ApplicationContext时发布此事件。关闭的上下文将达到其生命周期结束;它无法刷新或重新启动。 |
5 |
RequestHandledEvent 这是一个特定于Web的事件,它告诉所有Bean已处理HTTP请求。 |
Spring的事件处理是单线程的,因此如果发布了一个事件,除非所有接收者都收到消息,否则进程将被阻塞,并且流程将不会继续。因此,如果要使用事件处理,则在设计应用程序时应注意。
监听上下文事件
要监听上下文事件,Bean应该实现ApplicationListener接口,该接口只有一个方法onApplicationEvent()。让我们编写一个示例来查看事件如何传播以及如何根据某些事件编写代码来执行所需的任务。
让我们准备好一个可用的Eclipse IDE,并按照以下步骤创建一个Spring应用程序:
步骤 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个com.tutorialspoint包。 |
2 | 如Spring HelloWorld示例章节中所述,使用添加外部JAR选项添加所需的Spring库。 |
3 | 在com.tutorialspoint包下创建Java类HelloWorld、CStartEventHandler、CStopEventHandler和MainApp。 |
4 | 在src文件夹下创建Bean配置文件Beans.xml。 |
5 | 最后一步是创建所有Java文件和Bean配置文件的内容,并按如下所述运行应用程序。 |
以下是HelloWorld.java文件的内容
package com.tutorialspoint; public class HelloWorld { private String message; public void setMessage(String message){ this.message = message; } public void getMessage(){ System.out.println("Your Message : " + message); } }
以下是CStartEventHandler.java文件的内容
package com.tutorialspoint; import org.springframework.context.ApplicationListener; import org.springframework.context.event.ContextStartedEvent; public class CStartEventHandler implements ApplicationListener<ContextStartedEvent>{ public void onApplicationEvent(ContextStartedEvent event) { System.out.println("ContextStartedEvent Received"); } }
以下是CStopEventHandler.java文件的内容
package com.tutorialspoint; import org.springframework.context.ApplicationListener; import org.springframework.context.event.ContextStoppedEvent; public class CStopEventHandler implements ApplicationListener<ContextStoppedEvent>{ public void onApplicationEvent(ContextStoppedEvent event) { System.out.println("ContextStoppedEvent Received"); } }
以下是MainApp.java文件的内容
package com.tutorialspoint; import org.springframework.context.ConfigurableApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ConfigurableApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); // Let us raise a start event. context.start(); HelloWorld obj = (HelloWorld) context.getBean("helloWorld"); obj.getMessage(); // Let us raise a stop event. context.stop(); } }
以下是配置文件Beans.xml
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id = "helloWorld" class = "com.tutorialspoint.HelloWorld"> <property name = "message" value = "Hello World!"/> </bean> <bean id = "cStartEventHandler" class = "com.tutorialspoint.CStartEventHandler"/> <bean id = "cStopEventHandler" class = "com.tutorialspoint.CStopEventHandler"/> </beans>
创建源文件和Bean配置文件后,让我们运行应用程序。如果您的应用程序一切正常,它将打印以下消息:
ContextStartedEvent Received Your Message : Hello World! ContextStoppedEvent Received
如果您愿意,您可以发布您自己的自定义事件,稍后您可以捕获相同的事件以对这些自定义事件采取任何操作。如果您有兴趣编写您自己的自定义事件,您可以查看Spring中的自定义事件。