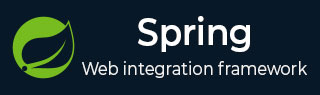
- Spring核心基础
- Spring - 首页
- Spring - 概述
- Spring - 架构
- Spring - 环境设置
- Spring - HelloWorld示例
- Spring - IoC容器
- Spring - Bean定义
- Spring - Bean 作用域
- Spring - Bean生命周期
- Spring - Bean后处理器
- Spring - Bean定义继承
- Spring - 依赖注入
- Spring - 注入内部Bean
- Spring - 注入集合
- Spring - Bean自动装配
- 基于注解的配置
- Spring - 基于Java的配置
- Spring - Spring中的事件处理
- Spring - Spring中的自定义事件
- Spring - Spring框架中的AOP
- Spring - JDBC框架
- Spring - 事务管理
- Spring - Web MVC框架
- Spring - 使用Log4J进行日志记录
- Spring问答
- Spring - 问答
- Spring实用资源
- Spring - 快速指南
- Spring - 实用资源
- Spring - 讨论
Spring - Bean 作用域
在定义<bean>时,您可以选择声明该bean的作用域。例如,要强制Spring每次需要时都生成一个新的bean实例,应将bean的作用域属性声明为prototype。同样,如果您希望Spring每次需要时都返回相同的bean实例,则应将bean的作用域属性声明为singleton。
Spring框架支持以下五个作用域,其中三个作用域仅在使用支持Web的ApplicationContext时才可用。
序号 | 作用域及描述 |
---|---|
1 |
singleton 此作用域将bean定义的作用域限定为每个Spring IoC容器一个实例(默认值)。 |
2 |
prototype 此作用域将单个bean定义的作用域限定为任意数量的对象实例。 |
3 |
request 此作用域将bean定义的作用域限定为HTTP请求。仅在支持Web的Spring ApplicationContext上下文中有效。 |
4 |
session 此作用域将bean定义的作用域限定为HTTP会话。仅在支持Web的Spring ApplicationContext上下文中有效。 |
5 |
global-session 此作用域将bean定义的作用域限定为全局HTTP会话。仅在支持Web的Spring ApplicationContext上下文中有效。 |
在本章中,我们将讨论前两个作用域,其余三个作用域将在讨论支持Web的Spring ApplicationContext时进行讨论。
singleton作用域
如果作用域设置为singleton,则Spring IoC容器将创建由该bean定义确定的对象的单个实例。此单个实例存储在这样的singleton bean的缓存中,并且对该命名bean的所有后续请求和引用都将返回缓存的对象。
默认作用域始终为singleton。但是,当您只需要一个bean的一个实例时,可以在bean配置文件中将scope属性设置为singleton,如下面的代码片段所示:
<!-- A bean definition with singleton scope --> <bean id = "..." class = "..." scope = "singleton"> <!-- collaborators and configuration for this bean go here --> </bean>
示例
让我们准备好一个可用的Eclipse IDE,并按照以下步骤创建一个Spring应用程序:
步骤 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个名为com.tutorialspoint的包。 |
2 | 如Spring HelloWorld示例章节中所述,使用添加外部JAR选项添加所需的Spring库。 |
3 | 在com.tutorialspoint包下创建Java类HelloWorld和MainApp。 |
4 | 在src文件夹下创建Bean配置文件Beans.xml。 |
5 | 最后一步是创建所有Java文件和Bean配置文件的内容,然后运行应用程序,如下所述。 |
以下是HelloWorld.java文件的内容:
package com.tutorialspoint; public class HelloWorld { private String message; public void setMessage(String message){ this.message = message; } public void getMessage(){ System.out.println("Your Message : " + message); } }
以下是MainApp.java文件的内容:
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); HelloWorld objA = (HelloWorld) context.getBean("helloWorld"); objA.setMessage("I'm object A"); objA.getMessage(); HelloWorld objB = (HelloWorld) context.getBean("helloWorld"); objB.getMessage(); } }
以下是singleton作用域所需的配置文件Beans.xml:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id = "helloWorld" class = "com.tutorialspoint.HelloWorld" scope = "singleton"> </bean> </beans>
创建源文件和bean配置文件后,让我们运行应用程序。如果应用程序一切正常,它将打印以下消息:
Your Message : I'm object A Your Message : I'm object A
prototype作用域
如果作用域设置为prototype,则Spring IoC容器每次对该特定bean发出请求时都会创建一个新的bean对象实例。通常,对于所有有状态bean使用prototype作用域,对于无状态bean使用singleton作用域。
要定义prototype作用域,可以在bean配置文件中将scope属性设置为prototype,如下面的代码片段所示:
<!-- A bean definition with prototype scope --> <bean id = "..." class = "..." scope = "prototype"> <!-- collaborators and configuration for this bean go here --> </bean>
示例
让我们准备好一个可用的Eclipse IDE,并按照以下步骤创建一个Spring应用程序:
步骤 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个名为com.tutorialspoint的包。 |
2 | 如Spring HelloWorld示例章节中所述,使用添加外部JAR选项添加所需的Spring库。 |
3 | 在com.tutorialspoint包下创建Java类HelloWorld和MainApp。 |
4 | 在src文件夹下创建Bean配置文件Beans.xml。 |
5 | 最后一步是创建所有Java文件和Bean配置文件的内容,然后运行应用程序,如下所述。 |
以下是HelloWorld.java文件的内容:
package com.tutorialspoint; public class HelloWorld { private String message; public void setMessage(String message){ this.message = message; } public void getMessage(){ System.out.println("Your Message : " + message); } }
以下是MainApp.java文件的内容:
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); HelloWorld objA = (HelloWorld) context.getBean("helloWorld"); objA.setMessage("I'm object A"); objA.getMessage(); HelloWorld objB = (HelloWorld) context.getBean("helloWorld"); objB.getMessage(); } }
以下是prototype作用域所需的配置文件Beans.xml:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id = "helloWorld" class = "com.tutorialspoint.HelloWorld" scope = "prototype"> </bean> </beans>
创建源文件和bean配置文件后,让我们运行应用程序。如果应用程序一切正常,它将打印以下消息:
Your Message : I'm object A Your Message : null