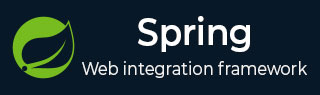
- Spring核心基础
- Spring - 首页
- Spring - 概述
- Spring - 架构
- Spring - 环境设置
- Spring - Hello World示例
- Spring - IoC容器
- Spring - Bean定义
- Spring - Bean作用域
- Spring - Bean生命周期
- Spring - Bean后处理器
- Spring - Bean定义继承
- Spring - 依赖注入
- Spring - 注入内部Bean
- Spring - 注入集合
- Spring - Bean自动装配
- 基于注解的配置
- Spring - 基于Java的配置
- Spring - Spring中的事件处理
- Spring - Spring中的自定义事件
- Spring - 使用Spring框架的AOP
- Spring - JDBC框架
- Spring - 事务管理
- Spring - Web MVC框架
- Spring - 使用Log4J进行日志记录
- Spring问答
- Spring - 问答
- Spring有用资源
- Spring - 快速指南
- Spring - 有用资源
- Spring - 讨论
程序化事务管理
程序化事务管理方法允许您在源代码中借助编程来管理事务。这为您提供了极大的灵活性,但难以维护。
在开始之前,重要的是至少有两个数据库表,我们可以借助事务在这些表上执行各种CRUD操作。让我们考虑一个**学生**表,它可以在MySQL TEST数据库中使用以下DDL创建:
CREATE TABLE Student( ID INT NOT NULL AUTO_INCREMENT, NAME VARCHAR(20) NOT NULL, AGE INT NOT NULL, PRIMARY KEY (ID) );
第二个表是**成绩**,我们将在其中根据年份维护学生的成绩。这里**SID**是学生表的外键。
CREATE TABLE Marks( SID INT NOT NULL, MARKS INT NOT NULL, YEAR INT NOT NULL );
让我们直接使用PlatformTransactionManager来实现程序化方法以实现事务。要启动新事务,您需要拥有一个具有适当事务属性的TransactionDefinition实例。对于此示例,我们将简单地创建一个ofDefaultTransactionDefinition实例以使用默认事务属性。
创建TransactionDefinition后,您可以通过调用getTransaction()方法启动事务,该方法返回TransactionStatus的实例。TransactionStatus对象有助于跟踪事务的当前状态,最后,如果一切正常,您可以使用PlatformTransactionManager的commit()方法提交事务,否则您可以使用rollback()回滚整个操作。
现在,让我们编写我们的Spring JDBC应用程序,它将在Student和Marks表上实现简单的操作。让我们准备一个可用的Eclipse IDE,并采取以下步骤创建一个Spring应用程序:
步骤 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个包com.tutorialspoint。 |
2 | 使用添加外部JAR选项添加所需的Spring库,如Spring Hello World示例章节中所述。 |
3 | 在项目中添加Spring JDBC特定的最新库mysql-connector-java.jar、org.springframework.jdbc.jar和org.springframework.transaction.jar。如果您还没有这些库,可以下载所需的库。 |
4 | 创建DAO接口StudentDAO并列出所有所需的方法。虽然这不是必需的,您可以直接编写StudentJDBCTemplate类,但作为一种良好的实践,让我们这样做。 |
5 | 在com.tutorialspoint包下创建其他所需的Java类StudentMarks、StudentMarksMapper、StudentJDBCTemplate和MainApp。如果需要,您可以创建其余的POJO类。 |
6 | 确保您已在TEST数据库中创建了Student和Marks表。还要确保您的MySQL服务器正常工作,并且您可以使用给定的用户名和密码访问数据库的读写权限。 |
7 | 在src文件夹下创建Beans配置文件Beans.xml。 |
8 | 最后一步是创建所有Java文件和Bean配置文件的内容,并按如下所述运行应用程序。 |
以下是数据访问对象接口文件StudentDAO.java的内容
package com.tutorialspoint; import java.util.List; import javax.sql.DataSource; public interface StudentDAO { /** * This is the method to be used to initialize * database resources ie. connection. */ public void setDataSource(DataSource ds); /** * This is the method to be used to create * a record in the Student and Marks tables. */ public void create(String name, Integer age, Integer marks, Integer year); /** * This is the method to be used to list down * all the records from the Student and Marks tables. */ public List<StudentMarks> listStudents(); }
以下是StudentMarks.java文件的内容
package com.tutorialspoint; public class StudentMarks { private Integer age; private String name; private Integer id; private Integer marks; private Integer year; private Integer sid; public void setAge(Integer age) { this.age = age; } public Integer getAge() { return age; } public void setName(String name) { this.name = name; } public String getName() { return name; } public void setId(Integer id) { this.id = id; } public Integer getId() { return id; } public void setMarks(Integer marks) { this.marks = marks; } public Integer getMarks() { return marks; } public void setYear(Integer year) { this.year = year; } public Integer getYear() { return year; } public void setSid(Integer sid) { this.sid = sid; } public Integer getSid() { return sid; } }
以下是StudentMarksMapper.java文件的内容
package com.tutorialspoint; import java.sql.ResultSet; import java.sql.SQLException; import org.springframework.jdbc.core.RowMapper; public class StudentMarksMapper implements RowMapper<StudentMarks> { public StudentMarks mapRow(ResultSet rs, int rowNum) throws SQLException { StudentMarks studentMarks = new StudentMarks(); studentMarks.setId(rs.getInt("id")); studentMarks.setName(rs.getString("name")); studentMarks.setAge(rs.getInt("age")); studentMarks.setSid(rs.getInt("sid")); studentMarks.setMarks(rs.getInt("marks")); studentMarks.setYear(rs.getInt("year")); return studentMarks; } }
以下是为已定义的DAO接口StudentDAO编写的实现类文件StudentJDBCTemplate.java
package com.tutorialspoint; import java.util.List; import javax.sql.DataSource; import org.springframework.dao.DataAccessException; import org.springframework.jdbc.core.JdbcTemplate; import org.springframework.transaction.PlatformTransactionManager; import org.springframework.transaction.TransactionDefinition; import org.springframework.transaction.TransactionStatus; import org.springframework.transaction.support.DefaultTransactionDefinition; public class StudentJDBCTemplate implements StudentDAO { private DataSource dataSource; private JdbcTemplate jdbcTemplateObject; private PlatformTransactionManager transactionManager; public void setDataSource(DataSource dataSource) { this.dataSource = dataSource; this.jdbcTemplateObject = new JdbcTemplate(dataSource); } public void setTransactionManager(PlatformTransactionManager transactionManager) { this.transactionManager = transactionManager; } public void create(String name, Integer age, Integer marks, Integer year){ TransactionDefinition def = new DefaultTransactionDefinition(); TransactionStatus status = transactionManager.getTransaction(def); try { String SQL1 = "insert into Student (name, age) values (?, ?)"; jdbcTemplateObject.update( SQL1, name, age); // Get the latest student id to be used in Marks table String SQL2 = "select max(id) from Student"; int sid = jdbcTemplateObject.queryForInt( SQL2 ); String SQL3 = "insert into Marks(sid, marks, year) " + "values (?, ?, ?)"; jdbcTemplateObject.update( SQL3, sid, marks, year); System.out.println("Created Name = " + name + ", Age = " + age); transactionManager.commit(status); } catch (DataAccessException e) { System.out.println("Error in creating record, rolling back"); transactionManager.rollback(status); throw e; } return; } public List<StudentMarks> listStudents() { String SQL = "select * from Student, Marks where Student.id=Marks.sid"; List <StudentMarks> studentMarks = jdbcTemplateObject.query(SQL, new StudentMarksMapper()); return studentMarks; } }
现在让我们继续使用主应用程序文件MainApp.java,如下所示:
package com.tutorialspoint; import java.util.List; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.tutorialspoint.StudentJDBCTemplate; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); StudentJDBCTemplate studentJDBCTemplate = (StudentJDBCTemplate)context.getBean("studentJDBCTemplate"); System.out.println("------Records creation--------" ); studentJDBCTemplate.create("Zara", 11, 99, 2010); studentJDBCTemplate.create("Nuha", 20, 97, 2010); studentJDBCTemplate.create("Ayan", 25, 100, 2011); System.out.println("------Listing all the records--------" ); List<StudentMarks> studentMarks = studentJDBCTemplate.listStudents(); for (StudentMarks record : studentMarks) { System.out.print("ID : " + record.getId() ); System.out.print(", Name : " + record.getName() ); System.out.print(", Marks : " + record.getMarks()); System.out.print(", Year : " + record.getYear()); System.out.println(", Age : " + record.getAge()); } } }
以下是配置文件Beans.xml
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd "> <!-- Initialization for data source --> <bean id = "dataSource" class = "org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name = "driverClassName" value = "com.mysql.jdbc.Driver"/> <property name = "url" value = "jdbc:mysql://127.0.0.1:3306/TEST"/> <property name = "username" value = "root"/> <property name = "password" value = "password"/> </bean> <!-- Initialization for TransactionManager --> <bean id = "transactionManager" class = "org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name = "dataSource" ref = "dataSource" /> </bean> <!-- Definition for studentJDBCTemplate bean --> <bean id = "studentJDBCTemplate" class = "com.tutorialspoint.StudentJDBCTemplate"> <property name = "dataSource" ref = "dataSource" /> <property name = "transactionManager" ref = "transactionManager" /> </bean> </beans>
创建源代码和Bean配置文件后,让我们运行应用程序。如果您的应用程序一切正常,它将打印以下消息:
------Records creation-------- Created Name = Zara, Age = 11 Created Name = Nuha, Age = 20 Created Name = Ayan, Age = 25 ------Listing all the records-------- ID : 1, Name : Zara, Marks : 99, Year : 2010, Age : 11 ID : 2, Name : Nuha, Marks : 97, Year : 2010, Age : 20 ID : 3, Name : Ayan, Marks : 100, Year : 2011, Age : 25