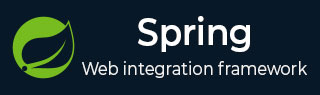
- Spring核心基础
- Spring - 首页
- Spring - 概述
- Spring - 架构
- Spring - 环境设置
- Spring - Hello World 示例
- Spring - IoC容器
- Spring - Bean定义
- Spring - Bean作用域
- Spring - Bean生命周期
- Spring - Bean后处理器
- Spring - Bean定义继承
- Spring - 依赖注入
- Spring - 注入内部Bean
- Spring - 注入集合
- Spring - Bean自动装配
- 基于注解的配置
- Spring - 基于Java的配置
- Spring - Spring中的事件处理
- Spring - Spring中的自定义事件
- Spring - Spring框架中的AOP
- Spring - JDBC框架
- Spring - 事务管理
- Spring - Web MVC框架
- Spring - 使用Log4J进行日志记录
- Spring问答
- Spring - 问答
- Spring有用资源
- Spring - 快速指南
- Spring - 有用资源
- Spring - 讨论
Spring @Autowired 注解
@Autowired 注解提供了更细粒度的控制,用于指定自动装配应该在哪里以及如何完成。@Autowired 注解可以用于自动装配setter方法上的Bean,就像@Required注解一样,也可以用于构造函数、属性或具有任意名称和/或多个参数的方法。
@Autowired 在Setter方法上
您可以使用@Autowired注解在setter方法上,以摆脱XML配置文件中的<property>元素。当Spring找到在setter方法上使用的@Autowired注解时,它会尝试对该方法执行按类型自动装配。
示例
让我们准备好一个Eclipse IDE,并按照以下步骤创建一个Spring应用程序:
步骤 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个包com.tutorialspoint。 |
2 | 如Spring Hello World 示例章节中所述,使用添加外部JAR选项添加所需的Spring库。 |
3 | 在com.tutorialspoint包下创建Java类TextEditor、SpellChecker和MainApp。 |
4 | 在src文件夹下创建Beans配置文件Beans.xml。 |
5 | 最后一步是创建所有Java文件和Bean配置文件的内容,并按如下所述运行应用程序。 |
以下是TextEditor.java文件的内容:
package com.tutorialspoint; import org.springframework.beans.factory.annotation.Autowired; public class TextEditor { private SpellChecker spellChecker; @Autowired public void setSpellChecker( SpellChecker spellChecker ){ this.spellChecker = spellChecker; } public SpellChecker getSpellChecker( ) { return spellChecker; } public void spellCheck() { spellChecker.checkSpelling(); } }
以下是另一个依赖类文件SpellChecker.java的内容:
package com.tutorialspoint; public class SpellChecker { public SpellChecker(){ System.out.println("Inside SpellChecker constructor." ); } public void checkSpelling(){ System.out.println("Inside checkSpelling." ); } }
以下是MainApp.java文件的内容:
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); TextEditor te = (TextEditor) context.getBean("textEditor"); te.spellCheck(); } }
以下是配置文件Beans.xml的内容:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:context = "http://www.springframework.org/schema/context" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <context:annotation-config/> <!-- Definition for textEditor bean without constructor-arg --> <bean id = "textEditor" class = "com.tutorialspoint.TextEditor"> </bean> <!-- Definition for spellChecker bean --> <bean id = "spellChecker" class = "com.tutorialspoint.SpellChecker"> </bean> </beans>
创建源文件和Bean配置文件后,让我们运行应用程序。如果应用程序一切正常,它将打印以下消息:
Inside SpellChecker constructor. Inside checkSpelling.
@Autowired 在属性上
您可以使用@Autowired注解在属性上,以摆脱setter方法。当您使用<property>传递自动装配属性的值时,Spring将自动使用传递的值或引用分配这些属性。因此,在属性上使用@Autowired后,您的TextEditor.java文件将如下所示:
package com.tutorialspoint; import org.springframework.beans.factory.annotation.Autowired; public class TextEditor { @Autowired private SpellChecker spellChecker; public TextEditor() { System.out.println("Inside TextEditor constructor." ); } public SpellChecker getSpellChecker( ){ return spellChecker; } public void spellCheck(){ spellChecker.checkSpelling(); } }
以下是配置文件Beans.xml的内容:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:context = "http://www.springframework.org/schema/context" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <context:annotation-config/> <!-- Definition for textEditor bean --> <bean id = "textEditor" class = "com.tutorialspoint.TextEditor"> </bean> <!-- Definition for spellChecker bean --> <bean id = "spellChecker" class = "com.tutorialspoint.SpellChecker"> </bean> </beans>
完成以上对源文件和Bean配置文件的两个更改后,让我们运行应用程序。如果应用程序一切正常,它将打印以下消息:
Inside TextEditor constructor. Inside SpellChecker constructor. Inside checkSpelling.
@Autowired 在构造函数上
您也可以将@Autowired应用于构造函数。构造函数@Autowired注解表示在创建Bean时应该自动装配构造函数,即使在XML文件中配置Bean时未使用<constructor-arg>元素。让我们检查下面的示例。
以下是TextEditor.java文件的内容:
package com.tutorialspoint; import org.springframework.beans.factory.annotation.Autowired; public class TextEditor { private SpellChecker spellChecker; @Autowired public TextEditor(SpellChecker spellChecker){ System.out.println("Inside TextEditor constructor." ); this.spellChecker = spellChecker; } public void spellCheck(){ spellChecker.checkSpelling(); } }
以下是配置文件Beans.xml的内容:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:context = "http://www.springframework.org/schema/context" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <context:annotation-config/> <!-- Definition for textEditor bean without constructor-arg --> <bean id = "textEditor" class = "com.tutorialspoint.TextEditor"> </bean> <!-- Definition for spellChecker bean --> <bean id = "spellChecker" class = "com.tutorialspoint.SpellChecker"> </bean> </beans>
完成以上对源文件和Bean配置文件的两个更改后,让我们运行应用程序。如果应用程序一切正常,它将打印以下消息:
Inside SpellChecker constructor. Inside TextEditor constructor. Inside checkSpelling.
@Autowired 使用(required = false)选项
默认情况下,@Autowired注解意味着依赖项是必需的,类似于@Required注解,但是,您可以使用(required=false)选项关闭@Autowired的默认行为。
即使您没有为age属性传递任何值,以下示例仍然可以工作,但它仍然需要name属性。您可以自己尝试这个示例,因为它类似于@Required注解示例,只是Student.java文件已更改。
package com.tutorialspoint; import org.springframework.beans.factory.annotation.Autowired; public class Student { private Integer age; private String name; @Autowired(required=false) public void setAge(Integer age) { this.age = age; } public Integer getAge() { return age; } @Autowired public void setName(String name) { this.name = name; } public String getName() { return name; } }