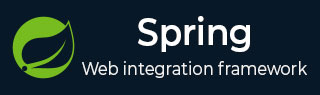
- Spring核心基础
- Spring - 首页
- Spring - 概述
- Spring - 架构
- Spring - 环境搭建
- Spring - Hello World 示例
- Spring - IoC 容器
- Spring - Bean 定义
- Spring - Bean 作用域
- Spring - Bean 生命周期
- Spring - Bean 后处理器
- Spring - Bean 定义继承
- Spring - 依赖注入
- Spring - 注入内部 Bean
- Spring - 注入集合
- Spring - Bean 自动装配
- 基于注解的配置
- Spring - 基于 Java 的配置
- Spring - Spring 中的事件处理
- Spring - Spring 中的自定义事件
- Spring - 使用 Spring 框架的 AOP
- Spring - JDBC 框架
- Spring - 事务管理
- Spring - Web MVC 框架
- Spring - 使用 Log4J 进行日志记录
- Spring 问题与解答
- Spring - 问题与解答
- Spring 有用资源
- Spring - 快速指南
- Spring - 有用资源
- Spring - 讨论
Spring 基于构造器的自动装配
此模式与byType非常相似,但它适用于构造函数参数。Spring容器查找在XML配置文件中autowire属性设置为constructor的Bean。然后尝试将其构造函数的参数与配置文件中的一个Bean名称进行匹配和连接。如果找到匹配项,它将注入这些Bean。否则,Bean将不会被连接。
例如,如果在配置文件中将Bean定义设置为通过constructor自动装配,并且它具有一个SpellChecker类型的参数的构造函数,则Spring会查找名为SpellChecker的Bean定义,并使用它来设置构造函数的参数。您仍然可以使用<constructor-arg>标签连接其余参数。以下示例将说明此概念。
让我们准备好一个可运行的Eclipse IDE,并按照以下步骤创建一个Spring应用程序:
步骤 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个com.tutorialspoint包。 |
2 | 如Spring Hello World 示例章节中所述,使用添加外部JAR选项添加所需的Spring库。 |
3 | 在com.tutorialspoint包下创建Java类TextEditor、SpellChecker和MainApp。 |
4 | 在src文件夹下创建Bean配置文件Beans.xml。 |
5 | 最后一步是创建所有Java文件和Bean配置文件的内容,然后运行应用程序,如下所述。 |
以下是TextEditor.java文件的内容:
package com.tutorialspoint; public class TextEditor { private SpellChecker spellChecker; private String name; public TextEditor( SpellChecker spellChecker, String name ) { this.spellChecker = spellChecker; this.name = name; } public SpellChecker getSpellChecker() { return spellChecker; } public String getName() { return name; } public void spellCheck() { spellChecker.checkSpelling(); } }
以下是另一个依赖类文件SpellChecker.java的内容:
package com.tutorialspoint; public class SpellChecker { public SpellChecker(){ System.out.println("Inside SpellChecker constructor." ); } public void checkSpelling(){ System.out.println("Inside checkSpelling." ); } }
以下是MainApp.java文件的内容:
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); TextEditor te = (TextEditor) context.getBean("textEditor"); te.spellCheck(); } }
以下是正常情况下Beans.xml配置文件的内容:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <!-- Definition for textEditor bean --> <bean id = "textEditor" class = "com.tutorialspoint.TextEditor"> <constructor-arg ref = "spellChecker" /> <constructor-arg value = "Generic Text Editor"/> </bean> <!-- Definition for spellChecker bean --> <bean id = "spellChecker" class = "com.tutorialspoint.SpellChecker"></bean> </beans>
但是,如果您要使用“按构造函数”自动装配,则XML配置文件将如下所示:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <!-- Definition for textEditor bean --> <bean id = "textEditor" class = "com.tutorialspoint.TextEditor" autowire = "constructor"> <constructor-arg value = "Generic Text Editor"/> </bean> <!-- Definition for spellChecker bean --> <bean id = "SpellChecker" class = "com.tutorialspoint.SpellChecker"></bean> </beans>
创建源代码和Bean配置文件后,让我们运行应用程序。如果您的应用程序一切正常,它将打印以下消息:
Inside SpellChecker constructor. Inside checkSpelling.
广告