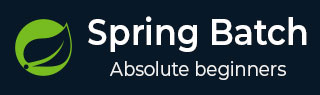
- Spring Batch 教程
- Spring Batch - 首页
- Spring Batch - 概述
- Spring Batch - 环境
- Spring Batch - 架构
- Spring Batch - 应用
- Spring Batch - 配置
- 读取器、写入器和处理器
- Spring Batch - 基础应用
- Spring Batch - XML 到 MySQL
- Spring Batch - CSV 到 XML
- Spring Batch - MySQL 到 XML
- Spring Batch - MySQL 到平面文件
- Spring Batch 有用资源
- Spring Batch - 快速指南
- Spring Batch - 有用资源
- Spring Batch - 讨论
Spring Batch - 基础应用
本章向您展示了 Spring Batch 的基本应用程序。它将简单地执行一个 **tasklet** 来显示一条消息。
我们的 Spring Batch 应用程序包含以下文件:
**配置文件** - 这是一个 XML 文件,我们在其中定义作业和作业的步骤。(如果应用程序还涉及读取器和写入器,则**读取器**和**写入器**的配置也包含在此文件中。)
**Context.xml** - 在此文件中,我们将定义像作业存储库、作业启动器和事务管理器这样的 bean。
**Tasklet 类** - 在此类中,我们将编写作业的处理代码(在本例中,它显示一条简单的消息)
**启动器类** - 在此类中,我们将通过运行作业启动器来启动批处理应用程序。
创建项目
创建一个新的 Maven 项目,如 Spring Batch - 环境 章节中所述。
pom.xml
以下是此 Maven 项目中使用的 pom.xml 文件的内容。
<project xmlns = "http://maven.apache.org/POM/4.0.0" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>SpringBatchSample</artifactId> <packaging>jar</packaging> <version>1.0-SNAPSHOT</version> <name>SpringBatchExample</name> <url>http://maven.apache.org</url> <properties> <jdk.version>21</jdk.version> <spring.version>5.3.14</spring.version> <spring.batch.version>4.3.4</spring.batch.version> <mysql.driver.version>5.1.25</mysql.driver.version> <junit.version>4.11</junit.version> </properties> <dependencies> <!-- Spring Core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <!-- Spring jdbc, for database --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring.version}</version> </dependency> <!-- Spring XML to/back object --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-oxm</artifactId> <version>${spring.version}</version> </dependency> <!-- MySQL database driver --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>${mysql.driver.version}</version> </dependency> <!-- Spring Batch dependencies --> <dependency> <groupId>org.springframework.batch</groupId> <artifactId>spring-batch-core</artifactId> <version>${spring.batch.version}</version> </dependency> <dependency> <groupId>org.springframework.batch</groupId> <artifactId>spring-batch-infrastructure</artifactId> <version>${spring.batch.version}</version> </dependency> <!-- Spring Batch unit test --> <dependency> <groupId>org.springframework.batch</groupId> <artifactId>spring-batch-test</artifactId> <version>${spring.batch.version}</version> </dependency> <!-- Junit --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> </dependencies> <build> <finalName>spring-batch</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-eclipse-plugin</artifactId> <version>2.9</version> <configuration> <downloadSources>true</downloadSources> <downloadJavadocs>false</downloadJavadocs> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.3.2</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> </plugins> </build> </project>
jobConfig.xml
以下是我们示例 Spring Batch 应用程序的配置文件。在 Maven 项目的 **src > main > resources** 文件夹中创建此文件。
<beans xmlns = "http://www.springframework.org/schema/beans" xmlns:batch = "http://www.springframework.org/schema/batch" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/batch http://www.springframework.org/schema/batch/spring-batch-2.2.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd "> <import resource="context.xml" /> <!-- Defining a bean --> <bean id = "tasklet" class = "MyTasklet" /> <!-- Defining a job--> <batch:job id = "helloWorldJob"> <!-- Defining a Step --> <batch:step id = "step1"> <tasklet ref = "tasklet"/> </batch:step> </batch:job> </beans>
Context.xml
以下是我们 Spring Batch 应用程序的 **context.xml**。在 Maven 项目的 **src > main > resources** 文件夹中创建此文件。
<beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd"> <bean id = "jobRepository" class="org.springframework.batch.core.repository.support.MapJobRepositoryFactoryBean"> <property name = "transactionManager" ref = "transactionManager" /> </bean> <bean id = "transactionManager" class = "org.springframework.batch.support.transaction.ResourcelessTransactionManager" /> <bean id = "jobLauncher" class = "org.springframework.batch.core.launch.support.SimpleJobLauncher"> <property name = "jobRepository" ref = "jobRepository" /> </bean> </beans>
Tasklet.java
以下是显示简单消息的 Tasklet 类。在 Maven 项目的 **src > main > java** 文件夹中创建此类。
import org.springframework.batch.core.StepContribution; import org.springframework.batch.core.scope.context.ChunkContext; import org.springframework.batch.core.step.tasklet.Tasklet; import org.springframework.batch.repeat.RepeatStatus; public class MyTasklet implements Tasklet { @Override public RepeatStatus execute(StepContribution arg0, ChunkContext arg1) throws Exception { System.out.println("Hello This is a sample example of spring batch"); return RepeatStatus.FINISHED; } }
App.java
以下是启动批处理过程的代码。在 Maven 项目的 **src > main > java** 文件夹中创建此类。
import org.springframework.batch.core.Job; import org.springframework.batch.core.JobExecution; import org.springframework.batch.core.JobParameters; import org.springframework.batch.core.launch.JobLauncher; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class App { public static void main(String[] args)throws Exception { String[] springConfig = {"jobConfig.xml"}; // Creating the application context object ApplicationContext context = new ClassPathXmlApplicationContext(springConfig); // Creating the job launcher JobLauncher jobLauncher = (JobLauncher) context.getBean("jobLauncher"); // Creating the job Job job = (Job) context.getBean("helloWorldJob"); // Executing the JOB JobExecution execution = jobLauncher.run(job, new JobParameters()); System.out.println("Exit Status : " + execution.getStatus()); } }
输出
在 Eclipse 中右键单击项目,选择 **以...方式运行 -> Maven 构建**。将目标设置为 **clean package** 并运行项目。您将看到以下输出。
[INFO] Scanning for projects... [INFO] [INFO] [1m----------------< [0;36mcom.tutorialspoint:SpringBatchSample[0;1m >----------------[m [INFO] [1mBuilding SpringBatchExample 1.0-SNAPSHOT[m [INFO] from pom.xml [INFO] [1m--------------------------------[ jar ]---------------------------------[m [INFO] [INFO] [1m--- [0;32mclean:3.2.0:clean[m [1m(default-clean)[m @ [36mSpringBatchSample[0;1m ---[m [INFO] Deleting C:\Users\Tutorialspoint\eclipse-workspace\SpringBatchSample\target [INFO] [INFO] [1m--- [0;32mresources:3.3.1:resources[m [1m(default-resources)[m @ [36mSpringBatchSample[0;1m ---[m [WARNING] Using platform encoding (UTF-8 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] Copying 3 resources from src\main\resources to target\classes [INFO] [INFO] [1m--- [0;32mcompiler:2.3.2:compile[m [1m(default-compile)[m @ [36mSpringBatchSample[0;1m ---[m [WARNING] File encoding has not been set, using platform encoding UTF-8, i.e. build is platform dependent! [INFO] Compiling 5 source files to C:\Users\Tutorialspoint\eclipse-workspace\SpringBatchSample\target\classes [INFO] [INFO] [1m--- [0;32mresources:3.3.1:testResources[m [1m(default-testResources)[m @ [36mSpringBatchSample[0;1m ---[m [WARNING] Using platform encoding (UTF-8 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] Copying 0 resource from src\test\resources to target\test-classes [INFO] [INFO] [1m--- [0;32mcompiler:2.3.2:testCompile[m [1m(default-testCompile)[m @ [36mSpringBatchSample[0;1m ---[m [INFO] Nothing to compile - all classes are up to date [INFO] [INFO] [1m--- [0;32msurefire:3.1.2:test[m [1m(default-test)[m @ [36mSpringBatchSample[0;1m ---[m [INFO] [INFO] [1m--- [0;32mjar:3.3.0:jar[m [1m(default-jar)[m @ [36mSpringBatchSample[0;1m ---[m [INFO] Building jar: C:\Users\Tutorialspoint\eclipse-workspace\SpringBatchSample\target\spring-batch.jar [INFO] [1m------------------------------------------------------------------------[m [INFO] [1;32mBUILD SUCCESS[m [INFO] [1m------------------------------------------------------------------------[m [INFO] Total time: 4.426 s [INFO] Finished at: 2024-07-30T11:10:59+05:30 [INFO] [1m------------------------------------------------------------------------
要检查上述 SpringBatch 程序的输出,请右键单击 App.java 类并选择 **以...方式运行 -> Java 应用程序**。它将产生以下输出:
Jul 30, 2024 11:21:25 AM org.springframework.batch.core.launch.support.SimpleJobLauncher afterPropertiesSet INFO: No TaskExecutor has been set, defaulting to synchronous executor. Jul 30, 2024 11:21:25 AM org.springframework.batch.core.launch.support.SimpleJobLauncher$1 run INFO: Job: [FlowJob: [name=helloWorldJob]] launched with the following parameters: [{}] Jul 30, 2024 11:21:25 AM org.springframework.batch.core.job.SimpleStepHandler handleStep INFO: Executing step: [step1] Hello This is a sample example of spring batch Jul 30, 2024 11:21:25 AM org.springframework.batch.core.step.AbstractStep execute INFO: Step: [step1] executed in 25ms Jul 30, 2024 11:21:25 AM org.springframework.batch.core.launch.support.SimpleJobLauncher$1 run INFO: Job: [FlowJob: [name=helloWorldJob]] completed with the following parameters: [{}] and the following status: [COMPLETED] in 47ms Exit Status : COMPLETED