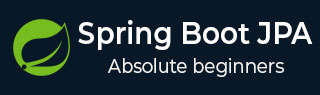
- Spring Boot JPA 教程
- Spring Boot JPA——主页
- Spring Boot JPA——概览
- Spring Boot JPA——环境设置
- Spring Boot JPA——架构
- Spring Boot JPA 与 Hibernate
- Spring Boot JPA——应用程序设置
- Spring Boot JPA——存储库单元测试
- Spring Boot JPA——方法
- Spring Boot JPA——自定义方法
- Spring Boot JPA——命名查询
- Spring Boot JPA——自定义查询
- Spring Boot JPA——本机查询
- Spring Boot JPA 便捷资源
- Spring Boot JPA——快速指南
- Spring Boot JPA——便捷资源
- Spring Boot JPA——讨论
Spring Boot JPA——存储库方法
我们现在来分析一下我们已创建的存储库界面中可用方法。
存储库——EmployeeRepository.java
以下是实现上面实体(Employee)上 CRUD 操作的存储库的默认代码。
package com.tutorialspoint.repository; import org.springframework.data.repository.CrudRepository; import org.springframework.stereotype.Repository; import com.tutorialspoint.entity.Employee; @Repository public interface EmployeeRepository extends CrudRepository<Employee, Integer> { }
这个存储库现在包含以下默认方法。
序号 | 方法和说明 |
---|---|
1 | count():long 返回可用的实体数。 |
2 | delete(Employee entity):void 删除一个实体。 |
3 | deleteAll():void 删除所有实体。 |
4 | deleteAll(Iterable< extends Employee > entities):void 删除作为参数传递的实体。 |
5 | deleteAll(Iterable< extends Integer > ids):void 删除使用作为参数传递的 ID 标识的实体。 |
6 | existsById(Integer id):boolean 使用 ID 检查实体是否存在。 |
7 | findAll():Iterable< Employee > 返回所有实体。 |
8 | findAllByIds(Iterable< Integer > ids):Iterable< Employee > 返回所有使用作为参数传递的 ID 识别的实体。 |
9 | findById(Integer id):Optional< Employee > 返回使用 ID 识别的实体。 |
10 | save(Employee entity):Employee 保存一个实体,并返回更新后的实体。 |
11 | saveAll(Iterable< Employee> entities): Iterable< Employee> 保存所有传递的实体,并返回更新后的实体。 |
广告