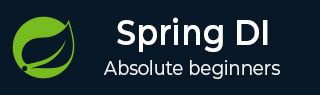
- Spring DI 教程
- Spring DI - 首页
- Spring DI - 概述
- Spring DI - 环境设置
- Spring DI - IOC容器
- Spring 依赖注入
- Spring DI - 创建项目
- 基于构造函数注入的示例
- Spring DI - 基于构造函数的依赖注入
- Spring DI - 内部Bean构造函数
- Spring DI - 集合构造函数
- Spring DI - 集合引用构造函数
- Spring DI - Map 构造函数
- Spring DI - Map 引用构造函数
- 基于Setter方法注入的示例
- Spring DI - 基于Setter方法的依赖注入
- Spring DI - 内部Bean Setter方法
- Spring DI - 集合 Setter方法
- Spring DI - 集合引用 Setter方法
- Spring DI - Map Setter方法
- Spring DI - Map 引用 Setter方法
- 自动装配示例
- Spring DI - 自动装配
- Spring DI - 按名称自动装配
- Spring DI - 按类型自动装配
- Spring DI - 构造函数自动装配
- 工厂方法
- Spring DI - 静态工厂方法
- Spring DI - 非静态工厂方法
- Spring DI 有用资源
- Spring DI - 快速指南
- Spring DI - 有用资源
- Spring DI - 讨论
Spring DI - 基于Setter方法的依赖注入
基于Setter方法的DI是通过容器在调用无参数构造函数或无参数静态工厂方法实例化Bean之后,调用Bean上的Setter方法来实现的。
示例
以下示例显示了一个名为TextEditor的类,它只能使用纯基于Setter方法的注入进行依赖注入。
让我们更新在Spring DI - 创建项目章节中创建的项目。我们添加以下文件:
TextEditor.java - 包含SpellChecker作为依赖项的类。
SpellChecker.java - 依赖类。
MainApp.java - 运行和测试主应用程序。
以下是TextEditor.java文件的内容:
package com.tutorialspoint; public class TextEditor { private SpellChecker spellChecker; // a setter method to inject the dependency. public void setSpellChecker(SpellChecker spellChecker) { System.out.println("Inside setSpellChecker." ); this.spellChecker = spellChecker; } // a getter method to return spellChecker public SpellChecker getSpellChecker() { return spellChecker; } public void spellCheck() { spellChecker.checkSpelling(); } }
在这里,您需要检查Setter方法的命名约定。要设置变量spellChecker,我们使用setSpellChecker()方法,这与Java POJO类非常相似。让我们创建另一个依赖类文件SpellChecker.java的内容:
package com.tutorialspoint; public class SpellChecker { public SpellChecker(){ System.out.println("Inside SpellChecker constructor." ); } public void checkSpelling() { System.out.println("Inside checkSpelling." ); } }
以下是MainApp.java文件的内容:
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("applicationcontext.xml"); TextEditor te = (TextEditor) context.getBean("textEditor"); te.spellCheck(); } }
以下是包含基于Setter方法注入配置的配置文件applicationcontext.xml:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <!-- Definition for textEditor bean --> <bean id = "textEditor" class = "com.tutorialspoint.TextEditor"> <property name = "spellChecker" ref = "spellChecker"/> </bean> <!-- Definition for spellChecker bean --> <bean id = "spellChecker" class = "com.tutorialspoint.SpellChecker"></bean> </beans>
您应该注意在基于构造函数注入和基于Setter方法注入中定义的applicationcontext.xml文件中的区别。唯一的区别在于<bean>元素内部,我们在基于构造函数注入中使用了<constructor-arg>标签,在基于Setter方法注入中使用了<property>标签。
第二个需要注意的重要点是,如果您正在传递对对象的引用,则需要使用<property>标签的ref属性;如果您直接传递值,则应使用value属性。
输出
创建源文件和Bean配置文件后,让我们运行应用程序。如果您的应用程序一切正常,这将打印以下消息:
Inside SpellChecker constructor. Inside setSpellChecker. Inside checkSpelling.
广告