在Flutter中添加3D对象
什么是Flutter中的3D对象?
3D对象就像三维对象,我们在应用程序中显示它们以对图像进行动画处理。我们可以对以3D对象形式显示的图像进行三维旋转以查看它。
在Flutter中实现3D对象
我们将创建一个简单的应用程序,在其中我们将显示一个文本视图和一个简单的3D对象在我们的Android应用程序中。我们将遵循分步指南在Android应用程序中实现吐司消息。
步骤1 - 在Android Studio中创建一个新的Flutter项目。
导航到Android Studio,如下面的屏幕所示。在下面的屏幕中,单击“新建项目”以创建新的Flutter项目。
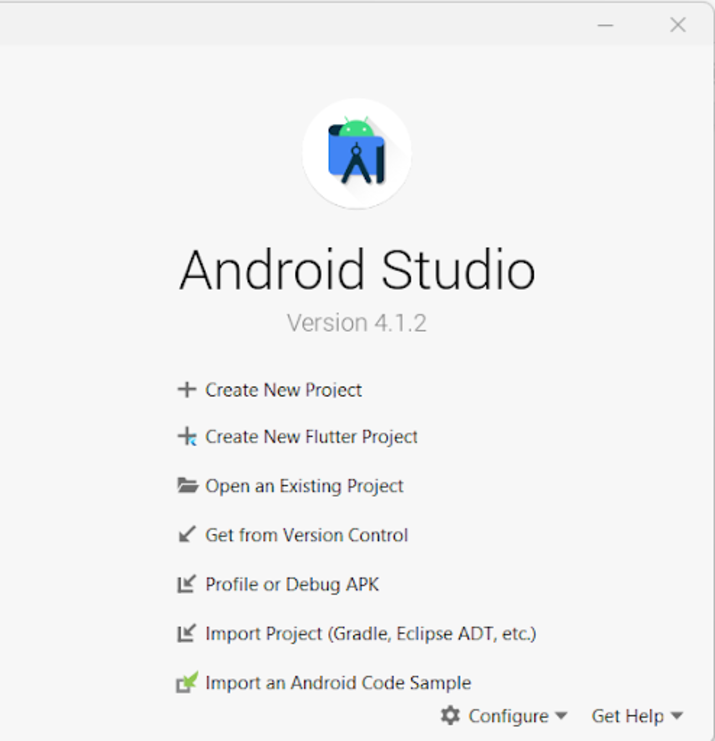
单击“新建项目”后,您将看到下面的屏幕。
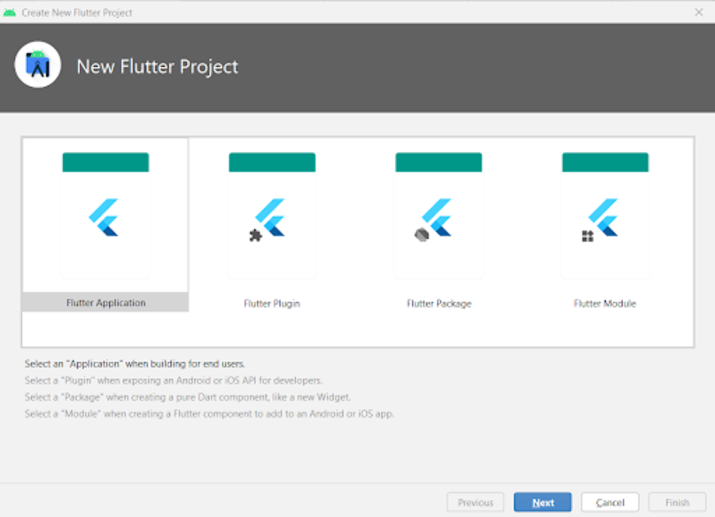
在此屏幕中,您必须选择Flutter Application,然后单击“下一步”,您将看到下面的屏幕。
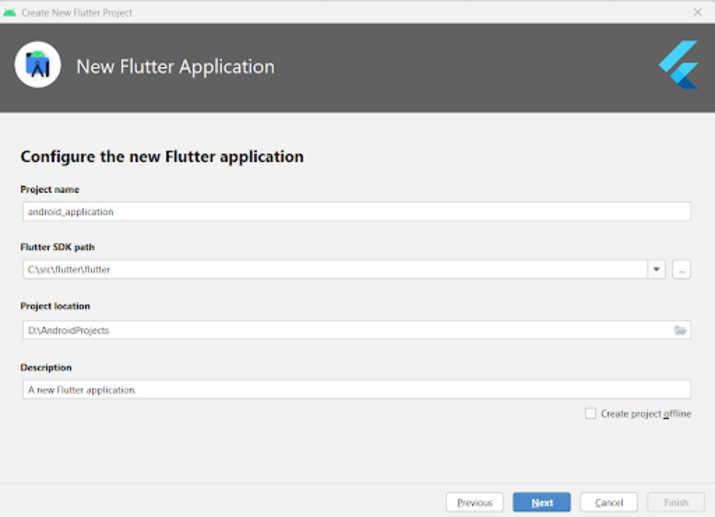
在此屏幕中,您必须指定项目名称,然后只需单击“下一步”即可
步骤2 - 在您的项目中添加3D图像。
从此链接下载3D图像并导航到您的Flutter项目。右键单击项目根目录>新建>目录,并将其命名为assets。复制下载的资产文件夹,并将该资产粘贴到您的assets文件夹中。
步骤3:使用pubspec.yaml文件。
在pubspec.yaml文件的dev_dependencies部分中添加flutter cube的依赖项。同时,在pubspec.yaml文件中添加资产。以下是它的代码。
dev_dependencies: flutter_test: sdk: flutter # The "flutter_lints" package below contains a set of recommended lints to # encourage good coding practices. The lint set provided by the package is # activated in the `analysis_options.yaml` file located at the root of your # package. See that file for information about deactivating specific lint # rules and activating additional ones. flutter_lints: ^1.0.0 flutter_cube: ^0.0.6 # For information on the generic Dart part of this file, see the # following page: https://dart.ac.cn/tools/pub/pubspec # The following section is specific to Flutter. flutter: # The following line ensures that the Material Icons font is # included with your application, so that you can use the icons in # the material Icons class. uses-material-design: true assets: - assets/earth/
添加上述代码后,只需单击“Pub get”即可安装项目中的所有依赖项。
步骤4 - 使用main.dart文件。
导航到您的项目>lib>main.dart文件,并在其中添加以下代码。代码中添加了注释以详细了解。
语法
import 'package:flutter/material.dart'; import 'package:flutter/rendering.dart'; import 'package:flutter_cube/flutter_cube.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( // on below line specifying title. title: 'Flutter Demo', // on below line hiding our debug banner debugShowCheckedModeBanner: false, // on below line setting theme. theme: ThemeData( primarySwatch: Colors.blue, ), // on below line displaying home page home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { // on below line creating an object for earth late Object earth; // on below line initializing state @override void initState() { // on below line initializing earth object earth = Object(fileName: "assets/earth/earth_ball.obj"); super.initState(); } @override Widget build(BuildContext context) { // on below line creating our UI. return Scaffold( // on below line creating a center widget. body: Center( // on below line creating a column child: Column( // on below line specifying alignment mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ // on below line adding a sized box const SizedBox( height: 100, ), // on below line adding a text for displaying a text for heading. const Text( // on below line specifying text message "3D Objects in Flutter", // on below line specifying text style style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20), ), // on below line adding a sized box const SizedBox( height: 20, ), // on below line creating an expanded widget for displaying an earth Expanded( // on below line creating a cube child: Cube( // on below line creating a scene onSceneCreated: (Scene scene) { // on below line adding a scene as earth scene.world.add(earth); // on below line setting camera for zoom. scene.camera.zoom = 5; }, ), ), ], ), ), ); } }
解释
在上面的代码中,我们可以看到main方法,在其中我们调用我们创建的MyApp类,作为无状态小部件。在这个无状态小部件中,我们创建一个build方法,在其中我们返回我们的material app。在material app中,我们指定应用程序标题、应用程序主题、要显示的主页以及将调试检查模式横幅设置为false。
之后,我们创建一个HomePage state方法,它将扩展HomePage的状态。在这个HomePage方法中,我们为名为earth的对象创建一个变量。然后我们创建一个initState方法,在其中我们使用我们在assets文件夹中添加的文件路径初始化earth变量。
之后,我们创建一个build方法,在其中我们指定一个主体到中心,然后在这个主体中,我们创建一个列,在其中我们指定横轴和主轴对齐方式以将视图对齐到中心。之后,我们创建子项,并在其中我们将创建我们的widget。首先,我们创建一个大小框以从顶部添加边距。之后,我们创建一个Text widget以简单地显示应用程序名称的文本。之后,我们再次添加一个大小框以添加间距。然后,我们添加一个Expanded widget以显示我们的3D对象。
在这个expanded widget中,我们添加一个子项作为Cube。在这个cube中,我们添加onScene created,在其中我们将场景设置为earth并将相机缩放设置为5。
添加上述代码后,现在我们只需单击顶栏中的绿色图标即可在移动设备上运行我们的应用程序。
注意 - 确保您已连接到您的真实设备或模拟器。
输出

结论
在上面的教程中,我们学习了什么是Flutter中的3D对象以及如何将3D对象添加到我们的应用程序中并使用它。