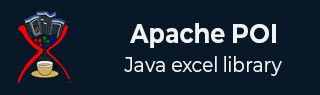
- Apache POI 教程
- Apache POI – 主页
- Apache POI – 概览
- Apache POI – Java Excel API
- Apache POI – 环境
- Apache POI – 核心类
- Apache POI – 工作簿
- Apache POI – 电子表格
- Apache POI – 单元格
- Apache POI – 字体
- Apache POI – 公式
- Apache POI – 链接
- Apache POI – 打印范围
- Apache POI – 数据库
- Apache POI 资源
- Apache POI – 问答
- Apache POI – 快速指南
- Apache POI – 有用资源
- Apache POI – 讨论
Apache POI – 数据库
本章解释了 POI 库如何与数据库进行交互。借助 JDBC,你可以从数据库中检索数据,并使用 POI 库将该数据插入电子表格。我们以 MySQL 数据库作为 SQL 操作的范例。
将数据从数据库写入 Excel
让我们假设要从 MySQL 数据库**test**中检索名为**emp_tbl**的以下员工数据表。
员工 ID | 员工姓名 | 学位 | 薪水 | 部门 |
---|---|---|---|---|
1201 | Gopal | 技术经理 | 45000 | IT |
1202 | Manisha | 校对员 | 45000 | 测试 |
1203 | Masthanvali | 技术作家 | 45000 | IT |
1204 | Kiran | 人事管理员 | 40000 | 人力资源 |
1205 | Kranthi | 运营管理员 | 30000 | 管理 |
使用以下代码从数据库中检索数据并将其插入电子表格。
import java.io.File; import java.io.FileOutputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; import org.apache.poi.xssf.usermodel.XSSFCell; import org.apache.poi.xssf.usermodel.XSSFRow; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; public class ExcelDatabase { public static void main(String[] args) throws Exception { Class.forName("com.mysql.jdbc.Driver"); Connection connect = DriverManager.getConnection( "jdbc:mysql://127.0.0.1:3306/test" , "root" , "root" ); Statement statement = connect.createStatement(); ResultSet resultSet = statement.executeQuery("select * from emp_tbl"); XSSFWorkbook workbook = new XSSFWorkbook(); XSSFSheet spreadsheet = workbook.createSheet("employe db"); XSSFRow row = spreadsheet.createRow(1); XSSFCell cell; cell = row.createCell(1); cell.setCellValue("EMP ID"); cell = row.createCell(2); cell.setCellValue("EMP NAME"); cell = row.createCell(3); cell.setCellValue("DEG"); cell = row.createCell(4); cell.setCellValue("SALARY"); cell = row.createCell(5); cell.setCellValue("DEPT"); int i = 2; while(resultSet.next()) { row = spreadsheet.createRow(i); cell = row.createCell(1); cell.setCellValue(resultSet.getInt("eid")); cell = row.createCell(2); cell.setCellValue(resultSet.getString("ename")); cell = row.createCell(3); cell.setCellValue(resultSet.getString("deg")); cell = row.createCell(4); cell.setCellValue(resultSet.getString("salary")); cell = row.createCell(5); cell.setCellValue(resultSet.getString("dept")); i++; } FileOutputStream out = new FileOutputStream(new File("exceldatabase.xlsx")); workbook.write(out); out.close(); System.out.println("exceldatabase.xlsx written successfully"); } }
我们将上述代码另存为**ExcelDatabase.java**。从命令提示符处编译并执行它,如下所示。
$javac ExcelDatabase.java $java ExcelDatabase
它将在你的当前目录中生成一个名为**exceldatabase.xlsx**的 Excel 文件,并在命令提示符处显示以下输出。
exceldatabase.xlsx written successfully
**exceldatabase.xlsx**文件如下所示。
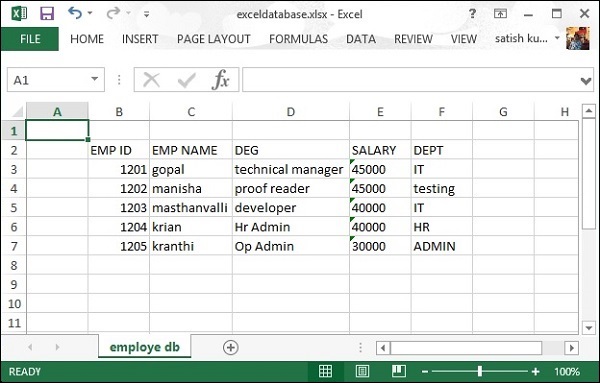
广告