Python中的数字低通巴特沃斯滤波器
低通滤波器是一种电子滤波器,它允许频率低于定义截止频率的信号通过,而频率高于截止频率的信号则会被衰减。高通巴特沃斯滤波器具有一些专门的特性,定义如下。
给定输入信号的采样率为 40 kHz。
通带的截止频率为 4 kHz。
阻带的截止频率为 8 kHz。
通带的纹波为 0.5 dB。
阻带的最小衰减为 40 dB,每个频率的衰减都基于滤波器设计。
实现低通巴特沃斯滤波器
Numpy 库提供了名为 butter() 的函数,该函数属于 scipy.signal 模块,用于通过将 btype 参数定义为 low 来创建低通巴特沃斯滤波器。以下是使用 Python 实现低通巴特沃斯滤波器的步骤。
步骤 1 - 在此步骤中,我们将定义用于传递较低频率的截止频率 f、采样频率 fs 和滤波器的阶数。
步骤 2 - 在 Python 中,我们有 scipy 库,它有一个名为 scipy.signal.butter() 的函数,用于使用定义的阶数和归一化频率设计巴特沃斯滤波器,将 btype 参数传递为 low 以实现低通巴特沃斯滤波器。
步骤 3 - 在此步骤中,我们将使用 scipy.signal.filtfilt() 函数创建给定输入信号频率的滤波器,以执行零相位滤波。
步骤 4 - 现在,我们将绘制低通巴特沃斯滤波器的输出频率和滤波后的输出频率。
示例
在以下示例中,我们通过组合所有提到的步骤来实现低通巴特沃斯滤波器。
import numpy as np import matplotlib.pyplot as plt from scipy.signal import butter, filtfilt t = np.linspace(0, 1, 1000, False) signal = np.sin(2*np.pi*10*t) + np.sin(2*np.pi*100*t) cutoff_freq = 50 nyquist_freq = 0.5 * 1000 order = 4 b, a = butter(order, cutoff_freq/nyquist_freq, btype='low') print("The output of the Low band pass Butterworth filter:",b,a) filtered_signal = filtfilt(b, a, signal) print("The output of the filtered Low band pass Butterworth filter:",filtered_signal[:60]) fig, (ax1, ax2) = plt.subplots(2, 1, sharex=True) ax1.plot(t, signal) ax1.set(title='Original signal') ax2.plot(t, filtered_signal) ax2.set(title='Filtered signal') plt.show()
输出
The output of the Low band pass Butterworth filter: [0.0004166 0.0016664 0.0024996 0.0016664 0.0004166] [ 1. -3.18063855 3.86119435 -2.11215536 0.43826514] The output of the filtered Low band pass Butterworth filter: [ 2.57011434e-02 8.61005841e-02 1.44535721e-01 2.01004914e-01 2.55827118e-01 3.09620894e-01 3.63131838e-01 4.16969733e-01 4.71355911e-01 5.25981148e-01 5.80034880e-01 6.32402662e-01 6.81965142e-01 7.27892755e-01 7.69831093e-01 8.07912298e-01 8.42592726e-01 8.74381837e-01 9.03567319e-01 9.30041579e-01 9.53294974e-01 9.72576767e-01 9.87160288e-01 9.96608860e-01 1.00093881e+00 1.00061547e+00 9.96382240e-01 9.88986904e-01 9.78909138e-01 9.66193004e-01 9.50448481e-01 9.31021733e-01 9.07269534e-01 8.78833540e-01 8.45810150e-01 8.08751539e-01 7.68497795e-01 7.25904493e-01 6.81569842e-01 6.35665536e-01 5.87935734e-01 5.37864244e-01 4.84945669e-01 4.28956498e-01 3.70122109e-01 3.09115413e-01 2.46887151e-01 1.84392199e-01 1.22315971e-01 6.09049996e-02 -3.39902671e-05 -6.09665336e-02 -1.22358749e-01 -1.84405645e-01 -2.46863503e-01 -3.09050695e-01 -3.70016926e-01 -4.28816577e-01 -4.84782072e-01 -5.37693155e-01]
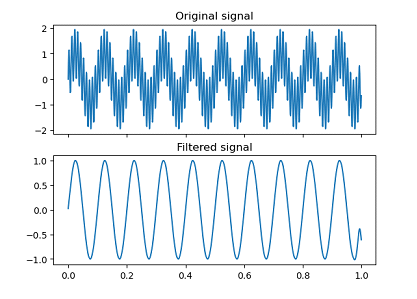
广告