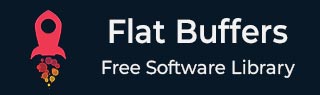
- FlatBuffers 教程
- FlatBuffers - 首页
- FlatBuffers - 简介
- FlatBuffers - 模式
- FlatBuffers - 结构
- FlatBuffers - 表格
- FlatBuffers - 字符串
- FlatBuffers - 数字
- FlatBuffers - 布尔值
- FlatBuffers - 枚举
- FlatBuffers - 向量
- FlatBuffers - 结构体
- FlatBuffers - 联合体
- FlatBuffers - 嵌套表格
- FlatBuffers - 默认值
- FlatBuffers - JSON 转二进制
- FlatBuffers - 二进制转 JSON
- FlatBuffers - 可变缓冲区
- FlatBuffers - 向后兼容性
- FlatBuffers - 语言独立性
- FlatBuffers 有用资源
- FlatBuffers - 快速指南
- FlatBuffers - 有用资源
- FlatBuffers - 讨论
FlatBuffers - 语言独立性
概述
到目前为止,我们一直在使用 Java 来序列化和反序列化电影院数据。但是,Google FlatBuffers 提供的关键特性之一是“语言独立性”。在本章中,我们将了解如何使用 Java 进行序列化,并使用 Python 进行反序列化。
继续我们从Flat Buffers - 字符串章节中的剧院示例,以下是我们在本示例中使用的模式:
theater.fbs
namespace com.tutorialspoint.theater; table Theater { name:string; address:string; } root_type Theater;
使用 Java 进行序列化
要使用 FlatBuffers,我们现在必须使用flatc二进制文件从这个“.fbs”文件创建所需的类。让我们看看如何做到这一点:
flatc --java theater.fbs
这将在当前目录中的com > tutorialspoint > theater文件夹中创建一个Theater.java类。我们在应用程序中使用此类,类似于Flat Buffers - 模式章节中所做的那样。
使用从 fbs 文件创建的 Java 类
首先,让我们创建一个写入器来写入剧院信息:
TheaterWriter.java
package com.tutorialspoint.theater; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import com.google.flatbuffers.FlatBufferBuilder; public class TheaterWriter { public static void main(String[] args) throws FileNotFoundException, IOException { // create a flat buffer builder // it will be used to create Theater FlatBuffer FlatBufferBuilder builder = new FlatBufferBuilder(1024); int name = builder.createString("Silver Screener"); int address = builder.createString("212, Maple Street, LA, California"); // create theater FlatBuffers using startTheater() method Theater.startTheater(builder); // add the name and address to the Theater FlatBuffer Theater.addName(builder, name); Theater.addAddress(builder, address); // mark end of data being entered in Greet FlatBuffer int theater = Theater.endTheater(builder); // finish the builder builder.finish(theater); // get the bytes to be stored byte[] data = builder.sizedByteArray(); String filename = "theater_flatbuffers_output"; System.out.println("Saving theater to file: " + filename); // write the builder content to the file named theater_flatbuffers_output try(FileOutputStream output = new FileOutputStream(filename)){ output.write(data); } System.out.println("Saved theater with following data to disk: \n" + theater); } }
编译项目
现在我们已经设置了读取器和写入器,让我们编译项目。
mvn clean install
序列化 Java 对象
现在,编译后,让我们先执行写入器:
java -cp .\target\flatbuffers-tutorial-1.0.jar com.tutorialspoint.theater.TheaterWriter Saving theater to file: theater_flatbuffers_output Saved theater with following data to disk: 72
使用 Python 反序列化序列化对象
从 proto 文件生成 Python 类
让我们为 Theater 类生成 Python 代码:
flatc --python theater.fbs
执行此命令后,您会注意到在当前目录中的com > tutorialspoint > theater文件夹中有一个自动生成的类Theater.py。此类将帮助我们反序列化剧院对象。
使用生成的 Python 类
现在,让我们编写数据的读取器,它将使用 Java 读取包含序列化对象的文件:
theaterReader.py
import Theater filename = "E:/theater_flatbuffers_output"; print("Reading from file: " + filename) theater = Theater.Theater() f = open(filename, "rb") buf = f.read() buf = bytearray(buf) theater = theater.GetRootAs(buf); f.close() print("Name: " + theater.Name().decode("utf-8")) print("Address: " + theater.Address().decode("utf-8"))
然后,让我们执行读取器。
py theaterReader.py Reading from file: E:/theater_flatbuffers_output Name: Silver Screener Address: 212, Maple Street, LA, California
因此,正如我们所看到的,Java 客户端写入的所有值都已正确反序列化并由我们的 Python 客户端读取,这有效地意味着 FlatBuffers 是语言独立的。
广告