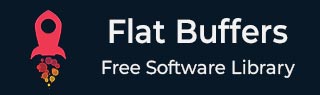
- Flat Buffers 教程
- Flat Buffers - 首页
- Flat Buffers - 简介
- Flat Buffers - 模式
- Flat Buffers - 结构
- Flat Buffers - 表格
- Flat Buffers - 字符串
- Flat Buffers - 数字
- Flat Buffers - 布尔值
- Flat Buffers - 枚举
- Flat Buffers - 向量
- Flat Buffers - 结构体
- Flat Buffers - 联合体
- Flat Buffers - 嵌套表格
- Flat Buffers - 默认值
- Flat Buffers - JSON 转二进制
- Flat Buffers - 二进制转 JSON
- Flat Buffers - 可变缓冲区
- Flat Buffers - 向后兼容性
- Flat Buffers - 语言独立性
- Flat Buffers 有用资源
- Flat Buffers - 快速指南
- Flat Buffers - 有用资源
- Flat Buffers - 讨论
Flat Buffers - 结构体
概述
struct 数据类型是 Flat Buffers 的复合数据类型之一。它用于创建不可变的数据结构。结构体占用更少的内存,并且查找速度很快。结构体通常是标量类型的组合。
继续我们从 Flat Buffers - 字符串 章节中的theater 示例,以下是我们需要使用的语法,以指示 FlatBuffers 我们将创建一个struct:
theater.fbs
namespace com.tutorialspoint.theater; struct Position { x: int; y: int; z: int; } table Theater { location: Position; } root_type Theater;
现在我们的表格包含定义为 Position 类型位置的结构体属性。Position 是一个结构体,用于定义三个整数的数据结构。
从 fbs 文件创建 Java 类
要使用 FlatBuffers,我们现在必须使用flatc二进制文件从这个“.fbs”文件创建所需的类。让我们看看如何操作:
flatc --java theater.fbs
这将在当前目录中的com > tutorialspoint > theater文件夹中创建Theater和Position类。我们在应用程序中使用此类,类似于在Flat Buffers - 模式章节中所做的那样。
使用从 fbs 文件创建的 Java 类
创建和写入结构体
为了创建一个结构体,我们需要首先准备标量类型数组的偏移量,然后我们可以将向量添加到扁平缓冲区。
// create offset for location struct int location = Position.createPosition(builder, 100, 110, 120); // add details to the Theater FlatBuffer Theater.addLocation(builder, location);
以下示例代码显示了创建整数结构体的过程。
TheaterWriter.java
package com.tutorialspoint.theater; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import com.google.flatbuffers.FlatBufferBuilder; public class TheaterWriter { public static void main(String[] args) throws FileNotFoundException, IOException { // create a flat buffer builder // it will be used to create Theater FlatBuffer FlatBufferBuilder builder = new FlatBufferBuilder(1024); // create offset for location struct int location = Position.createPosition(builder, 100, 110, 120); // create theater FlatBuffers using startTheater() method Theater.startTheater(builder); // add details to the Theater FlatBuffer Theater.addLocation(builder, location); // mark end of data being entered in Greet FlatBuffer int theater = Theater.endTheater(builder); // finish the builder builder.finish(theater); // get the bytes to be stored byte[] data = builder.sizedByteArray(); String filename = "theater_flatbuffers_output"; System.out.println("Saving theater to file: " + filename); // write the builder content to the file named theater_flatbuffers_output try(FileOutputStream output = new FileOutputStream(filename)){ output.write(data); } System.out.println("Saved theater with following data to disk: \n" + theater); } }
读取结构体
为了读取结构体,我们有方法可以获取结构体的每个值。
Position position = theater.location(); System.out.println("x: " + position.x()); System.out.println("y: " + position.y()); System.out.println("z: " + position.z());
以下示例代码显示了读取整数结构体的过程。
TheaterReader.java
package com.tutorialspoint.theater; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.nio.ByteBuffer; public class TheaterReader { public static void main(String[] args) throws FileNotFoundException, IOException { String filename = "theater_flatbuffers_output"; System.out.println("Reading from file " + filename); try(FileInputStream input = new FileInputStream(filename)) { // get the serialized data byte[] data = input.readAllBytes(); ByteBuffer buf = ByteBuffer.wrap(data); // read the root object in serialized data Theater theater = Theater.getRootAsTheater(buf); // print theater values System.out.println("Location: "); Position position = theater.location(); System.out.println("x: " + position.x()); System.out.println("y: " + position.y()); System.out.println("z: " + position.z()); } } }
编译项目
现在我们已经设置了读取器和写入器,让我们编译项目。
mvn clean install
序列化 Java 对象
现在,编译后,让我们首先执行写入器:
> java -cp .\target\flatbuffers-tutorial-1.0.jar com.tutorialspoint.theater.TheaterWriter Saving theater information to file: theater_flatbuffers_output Saved theater information with following data to disk: 16
反序列化序列化对象
现在,让我们执行读取器从同一个文件读取:
java -cp .\target\flatbuffers-tutorial-1.0.jar com.tutorialspoint.theater.TheaterReader Reading from file theater_flatbuffers_output Location: x: 100 y: 110 z: 120
因此,正如我们所看到的,我们能够通过将二进制数据反序列化为Theater对象来读取序列化的结构体。在下一章Flat Buffers - 联合体中,我们将了解联合体,这是一种复合类型。
广告