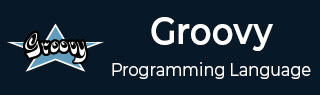
- Groovy 教程
- Groovy - 首页
- Groovy - 概述
- Groovy - 环境配置
- Groovy - 基本语法
- Groovy - 数据类型
- Groovy - 变量
- Groovy - 运算符
- Groovy - 循环
- Groovy - 条件判断
- Groovy - 方法
- Groovy - 文件 I/O
- Groovy - 可选值
- Groovy - 数字
- Groovy - 字符串
- Groovy - 范围
- Groovy - 列表
- Groovy - 映射
- Groovy - 日期和时间
- Groovy - 正则表达式
- Groovy - 异常处理
- Groovy - 面向对象编程
- Groovy - 泛型
- Groovy - 特质
- Groovy - 闭包
- Groovy - 注解
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSL
- Groovy - 数据库
- Groovy - 构造器
- Groovy - 命令行
- Groovy - 单元测试
- Groovy - 模板引擎
- Groovy - 元对象编程
- Groovy 有用资源
- Groovy - 快速指南
- Groovy - 有用资源
- Groovy - 讨论
Groovy - 基本语法
为了理解 Groovy 的基本语法,让我们首先来看一个简单的 Hello World 程序。
创建你的第一个 Hello World 程序
创建你的第一个 Hello World 程序就像输入以下代码行一样简单:
class Example { static void main(String[] args) { // Using a simple println statement to print output to the console println('Hello World'); } }
运行上述程序后,我们将得到以下结果:
Hello World
Groovy 中的导入语句
导入语句可以用来导入其他库的功能,这些功能可以在你的代码中使用。这是通过使用 **import** 关键字来完成的。
以下示例演示了如何使用 MarkupBuilder 类的简单导入,这可能是用于创建 HTML 或 XML 标记的最常用的类之一。
import groovy.xml.MarkupBuilder def xml = new MarkupBuilder()
默认情况下,Groovy 在你的代码中包含以下库,因此你不需要显式地导入它们。
import java.lang.* import java.util.* import java.io.* import java.net.* import groovy.lang.* import groovy.util.* import java.math.BigInteger import java.math.BigDecimal
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
Groovy 中的标记
标记可以是关键字、标识符、常量、字符串字面量或符号。
println(“Hello World”);
在上方的代码行中,有两个标记,第一个是关键字 println,下一个是“Hello World”的字符串字面量。
Groovy 中的注释
注释用于记录你的代码。Groovy 中的注释可以是单行注释或多行注释。
单行注释通过在行中的任何位置使用 // 来标识。如下所示:
class Example { static void main(String[] args) { // Using a simple println statement to print output to the console println('Hello World'); } }
多行注释以 /* 开头,以 */ 结尾。
class Example { static void main(String[] args) { /* This program is the first program This program shows how to display hello world */ println('Hello World'); } }
分号
与 Java 编程语言不同,在每个语句的末尾使用分号不是强制性的,它是可选的。
class Example { static void main(String[] args) { def x = 5 println('Hello World'); } }
如果你执行上述程序,主方法中的两个语句都不会产生任何错误。
标识符
标识符用于定义变量、函数或其他用户定义的变量。标识符以字母、美元符号或下划线开头。它们不能以数字开头。以下是一些有效标识符的示例:
def employeename def student1 def student_name
其中 **def** 是 Groovy 中用于定义标识符的关键字。
以下是一个代码示例,演示如何在我们的 Hello World 程序中使用标识符。
class Example { static void main(String[] args) { // One can see the use of a semi-colon after each statement def x = 5; println('Hello World'); } }
在上面的示例中,变量 **x** 用作标识符。
关键字
顾名思义,关键字是在 Groovy 编程语言中保留的特殊单词。下表列出了 Groovy 中定义的关键字。
as | assert | break | case |
catch | class | const | continue |
def | default | do | else |
enum | extends | false | finally |
for | goto | if | implements |
import | in | instanceof | interface |
new | pull | package | return |
super | switch | this | throw |
throws | trait | true | try |
while |
空格
空格是 Java 和 Groovy 等编程语言中用来描述空格、制表符、换行符和注释的术语。空格将语句的一个部分与另一个部分分开,并使编译器能够识别语句中的一个元素在哪里。
例如,在下面的代码示例中,关键字 **def** 和变量 x 之间有一个空格。这是为了让编译器知道需要使用 **def** 关键字,并且 x 应该是需要定义的变量名。
def x = 5;
字面量
字面量是表示 Groovy 中固定值的符号。Groovy 语言具有整数、浮点数、字符和字符串的符号。以下是 Groovy 编程语言中字面量的一些示例:
12 1.45 ‘a’ “aa”