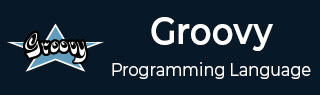
- Groovy 教程
- Groovy - 首页
- Groovy - 概述
- Groovy - 环境
- Groovy - 基本语法
- Groovy - 数据类型
- Groovy - 变量
- Groovy - 运算符
- Groovy - 循环
- Groovy - 决策
- Groovy - 方法
- Groovy - 文件 I/O
- Groovy - 可选值
- Groovy - 数字
- Groovy - 字符串
- Groovy - 范围
- Groovy - 列表
- Groovy - 映射
- Groovy - 日期和时间
- Groovy - 正则表达式
- Groovy - 异常处理
- Groovy - 面向对象
- Groovy - 泛型
- Groovy - 特性
- Groovy - 闭包
- Groovy - 注解
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSL
- Groovy - 数据库
- Groovy - 生成器
- Groovy - 命令行
- Groovy - 单元测试
- Groovy - 模板引擎
- Groovy - 元对象编程
- Groovy 有用资源
- Groovy - 快速指南
- Groovy - 有用资源
- Groovy - 讨论
Groovy - 变量
Groovy 中的变量可以通过两种方式定义:使用原生语法指定数据类型,或者使用 def 关键字。在变量定义中,必须显式提供类型名称或使用 "def" 替代。这是 Groovy 解析器所要求的。
如下所示是 Groovy 中的基本变量类型(在上一章中已有解释):
byte - 用于表示字节值。例如:2。
short - 用于表示短整型数。例如:10。
int - 用于表示整数。例如:1234。
long - 用于表示长整型数。例如:10000090。
float - 用于表示 32 位浮点数。例如:12.34。
double - 用于表示 64 位浮点数,是更长的十进制数表示,有时可能需要。例如:12.3456565。
char - 定义单个字符字面量。例如:'a'。
Boolean - 表示布尔值,可以是 true 或 false。
String - 是文本字面量,以字符链的形式表示。例如:“Hello World”。
Groovy 还允许使用其他类型的变量,例如数组、结构和类,我们将在后续章节中介绍。
变量声明
变量声明告诉编译器在哪里以及为变量创建多少存储空间。
以下是一个变量声明的示例:
class Example { static void main(String[] args) { // x is defined as a variable String x = "Hello"; // The value of the variable is printed to the console println(x); } }
运行上述程序后,我们将得到以下结果:
Hello
变量命名
变量名可以由字母、数字和下划线组成。它必须以字母或下划线开头。大小写字母是不同的,因为 Groovy 和 Java 一样,是一种区分大小写的编程语言。
class Example { static void main(String[] args) { // Defining a variable in lowercase int x = 5; // Defining a variable in uppercase int X = 6; // Defining a variable with the underscore in it's name def _Name = "Joe"; println(x); println(X); println(_Name); } }
运行上述程序后,我们将得到以下结果:
5 6 Joe
我们可以看到x和X是两个不同的变量(因为区分大小写),在第三种情况下,我们可以看到 _Name 以下划线开头。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
打印变量
您可以使用 println 函数打印变量的当前值。以下示例演示了如何实现这一点。
class Example { static void main(String[] args) { //Initializing 2 variables int x = 5; int X = 6; //Printing the value of the variables to the console println("The value of x is " + x + "The value of X is " + X); } }
运行上述程序后,我们将得到以下结果:
The value of x is 5 The value of X is 6