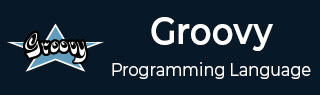
- Groovy 教程
- Groovy - 首页
- Groovy - 概述
- Groovy - 环境配置
- Groovy - 基本语法
- Groovy - 数据类型
- Groovy - 变量
- Groovy - 运算符
- Groovy - 循环
- Groovy - 条件判断
- Groovy - 方法
- Groovy - 文件 I/O
- Groovy - 可选值
- Groovy - 数字
- Groovy - 字符串
- Groovy - 范围
- Groovy - 列表
- Groovy - 映射
- Groovy - 日期和时间
- Groovy - 正则表达式
- Groovy - 异常处理
- Groovy - 面向对象编程
- Groovy - 泛型
- Groovy - 特性
- Groovy - 闭包
- Groovy - 注解
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSL
- Groovy - 数据库
- Groovy - 构造器
- Groovy - 命令行
- Groovy - 单元测试
- Groovy - 模板引擎
- Groovy - 元对象编程
- Groovy 有用资源
- Groovy - 快速指南
- Groovy - 有用资源
- Groovy - 讨论
Groovy - 异常处理
任何编程语言都需要异常处理来处理运行时错误,以便维护应用程序的正常流程。
异常通常会中断应用程序的正常流程,这就是为什么我们需要在应用程序中使用异常处理的原因。
异常大致分为以下几类:
检查异常 - 扩展 Throwable 类但不包括 RuntimeException 和 Error 的类被称为检查异常,例如 IOException、SQLException 等。检查异常在编译时进行检查。
一个典型的例子是 FileNotFoundException。假设您的应用程序中有以下代码从 E 盘读取文件。
class Example { static void main(String[] args) { File file = new File("E://file.txt"); FileReader fr = new FileReader(file); } }
如果 E 盘中不存在该文件 (file.txt),则会引发以下异常。
捕获到:java.io.FileNotFoundException: E:\file.txt (系统找不到指定的文件)。
java.io.FileNotFoundException: E:\file.txt (系统找不到指定的文件)。
未检查异常 - 扩展 RuntimeException 的类被称为未检查异常,例如 ArithmeticException、NullPointerException、ArrayIndexOutOfBoundsException 等。未检查异常不在编译时检查,而是在运行时检查。
一个典型的例子是 ArrayIndexOutOfBoundsException,它发生在您尝试访问数组索引超出数组长度时。以下是一个此类错误的典型示例。
class Example { static void main(String[] args) { def arr = new int[3]; arr[5] = 5; } }
执行上述代码时,将引发以下异常。
捕获到:java.lang.ArrayIndexOutOfBoundsException: 5
java.lang.ArrayIndexOutOfBoundsException: 5
错误 - 错误是不可恢复的,例如 OutOfMemoryError、VirtualMachineError、AssertionError 等。
这些是程序无法从中恢复的错误,并将导致程序崩溃。
下图显示了 Groovy 中异常的继承结构。它完全基于 Java 中定义的继承结构。
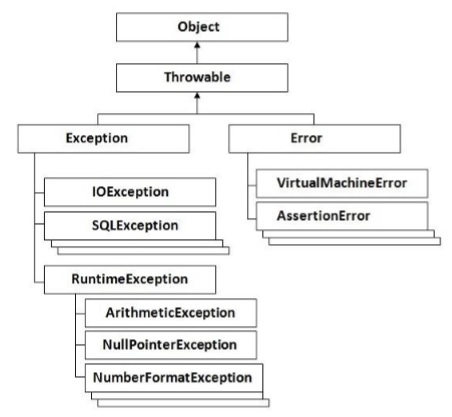
捕获异常
方法使用try和catch关键字的组合来捕获异常。try/catch 块放置在可能生成异常的代码周围。
try { //Protected code } catch(ExceptionName e1) { //Catch block }
所有可能引发异常的代码都放在受保护的代码块中。
在 catch 块中,您可以编写自定义代码来处理异常,以便应用程序可以从异常中恢复。
让我们来看一个与上面类似的例子,访问数组的索引值大于数组大小。但是这次让我们将代码包装在 try/catch 块中。
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; } catch(Exception ex) { println("Catching the exception"); } println("Let's move on after the exception"); } }
运行上述程序时,我们将得到以下结果:
Catching the exception Let's move on after the exception
从上面的代码中,我们将有问题的代码包装在 try 块中。在 catch 块中,我们只是捕获异常并输出一条消息,指出发生了异常。
多个 catch 块
可以使用多个 catch 块来处理多种类型的异常。对于每个 catch 块,根据引发的异常类型,您可以编写相应的代码来处理它。
让我们修改上面的代码来专门捕获 ArrayIndexOutOfBoundsException。以下是用代码片段。
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; }catch(ArrayIndexOutOfBoundsException ex) { println("Catching the Array out of Bounds exception"); }catch(Exception ex) { println("Catching the exception"); } println("Let's move on after the exception"); } }
运行上述程序时,我们将得到以下结果:
Catching the Aray out of Bounds exception Let's move on after the exception
从上面的代码可以看出,ArrayIndexOutOfBoundsException catch 块首先被捕获,因为它满足异常的条件。
finally 块
finally块跟在 try 块或 catch 块之后。finally 块的代码始终执行,无论是否发生异常。
使用 finally 块允许您运行任何您想要执行的清理类型的语句,无论受保护的代码中发生什么情况。此块的语法如下所示。
try { //Protected code } catch(ExceptionType1 e1) { //Catch block } catch(ExceptionType2 e2) { //Catch block } catch(ExceptionType3 e3) { //Catch block } finally { //The finally block always executes. }
让我们修改上面的代码并添加 finally 代码块。以下是用代码片段。
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; } catch(ArrayIndexOutOfBoundsException ex) { println("Catching the Array out of Bounds exception"); }catch(Exception ex) { println("Catching the exception"); } finally { println("The final block"); } println("Let's move on after the exception"); } }
运行上述程序时,我们将得到以下结果:
Catching the Array out of Bounds exception The final block Let's move on after the exception
以下是 Groovy 中可用的异常方法:
public String getMessage()
返回关于发生的异常的详细消息。此消息在 Throwable 构造函数中初始化。
public Throwable getCause()
返回异常的原因,以 Throwable 对象表示。
public String toString()
返回类名与 getMessage() 结果的连接。
public void printStackTrace()
将 toString() 的结果以及堆栈跟踪打印到 System.err(错误输出流)。
public StackTraceElement [] getStackTrace()
返回一个包含堆栈跟踪中每个元素的数组。索引为 0 的元素表示调用堆栈的顶部,数组中的最后一个元素表示调用堆栈底部的函数。
public Throwable fillInStackTrace()
使用当前堆栈跟踪填充此 Throwable 对象的堆栈跟踪,添加到堆栈跟踪中的任何先前信息。
示例
以下是使用上面一些方法的代码示例:
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; }catch(ArrayIndexOutOfBoundsException ex) { println(ex.toString()); println(ex.getMessage()); println(ex.getStackTrace()); } catch(Exception ex) { println("Catching the exception"); }finally { println("The final block"); } println("Let's move on after the exception"); } }
运行上述程序时,我们将得到以下结果:
java.lang.ArrayIndexOutOfBoundsException: 5 5 [org.codehaus.groovy.runtime.dgmimpl.arrays.IntegerArrayPutAtMetaMethod$MyPojoMetaMet hodSite.call(IntegerArrayPutAtMetaMethod.java:75), org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCall(CallSiteArray.java:48) , org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:113) , org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:133) , Example.main(Sample:8), sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method), sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57), sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) , java.lang.reflect.Method.invoke(Method.java:606), org.codehaus.groovy.reflection.CachedMethod.invoke(CachedMethod.java:93), groovy.lang.MetaMethod.doMethodInvoke(MetaMethod.java:325), groovy.lang.MetaClassImpl.invokeStaticMethod(MetaClassImpl.java:1443), org.codehaus.groovy.runtime.InvokerHelper.invokeMethod(InvokerHelper.java:893), groovy.lang.GroovyShell.runScriptOrMainOrTestOrRunnable(GroovyShell.java:287), groovy.lang.GroovyShell.run(GroovyShell.java:524), groovy.lang.GroovyShell.run(GroovyShell.java:513), groovy.ui.GroovyMain.processOnce(GroovyMain.java:652), groovy.ui.GroovyMain.run(GroovyMain.java:384), groovy.ui.GroovyMain.process(GroovyMain.java:370), groovy.ui.GroovyMain.processArgs(GroovyMain.java:129), groovy.ui.GroovyMain.main(GroovyMain.java:109), sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method), sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57), sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) , java.lang.reflect.Method.invoke(Method.java:606), org.codehaus.groovy.tools.GroovyStarter.rootLoader(GroovyStarter.java:109), org.codehaus.groovy.tools.GroovyStarter.main(GroovyStarter.java:131), sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method), sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57), sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) , java.lang.reflect.Method.invoke(Method.java:606), com.intellij.rt.execution.application.AppMain.main(AppMain.java:144)] The final block Let's move on after the exception