如何在 Android 中以编程方式读取设备上的短信?
简介
在 Android 应用程序中,我们经常看到应用程序读取用户手机上的短信并在应用程序内显示。例如,当我们创建短信应用程序时,我们会将用户手机上的短信显示在我们的应用程序中。在本文中,我们将探讨如何在 Android 中以编程方式读取设备上的短信。
实现
我们将创建一个简单的应用程序,其中我们将创建一个 TextView 用于显示应用程序标题。然后,我们将创建另外三个 TextView,分别用于显示发件人姓名、短信正文以及接收短信的日期。在我们的应用程序中,我们将显示收件箱中最近收到的短信。现在让我们转到 Android Studio 创建一个新项目。
步骤 1:在 Android Studio 中创建一个新项目
导航到 Android Studio,如下面的屏幕所示。在下面的屏幕中,点击“新建项目”以创建一个新的 Android Studio 项目。
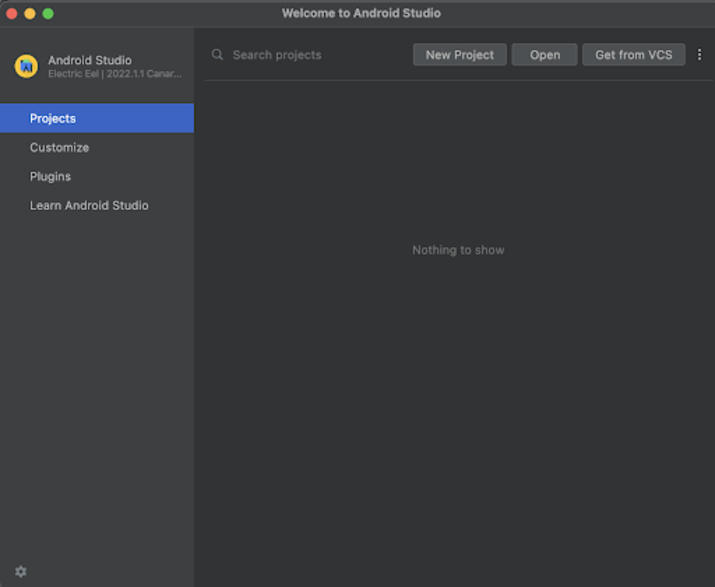
点击“新建项目”后,您将看到下面的屏幕。
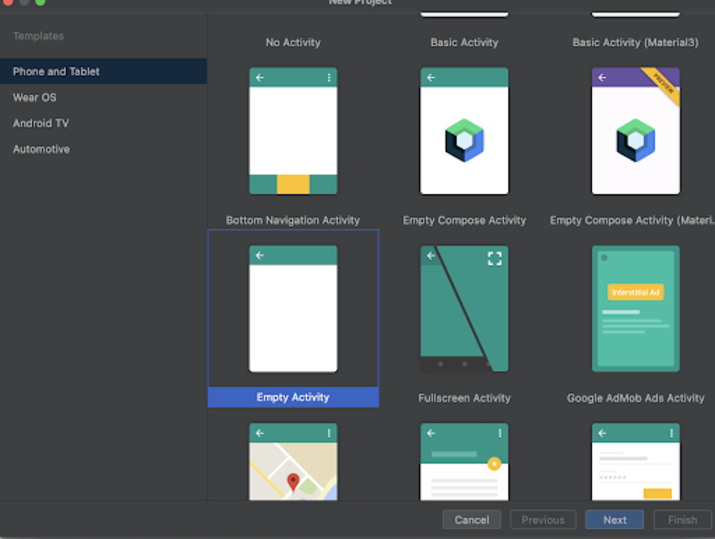
在这个屏幕中,我们只需选择“空活动”并点击“下一步”。点击“下一步”后,您将看到下面的屏幕。
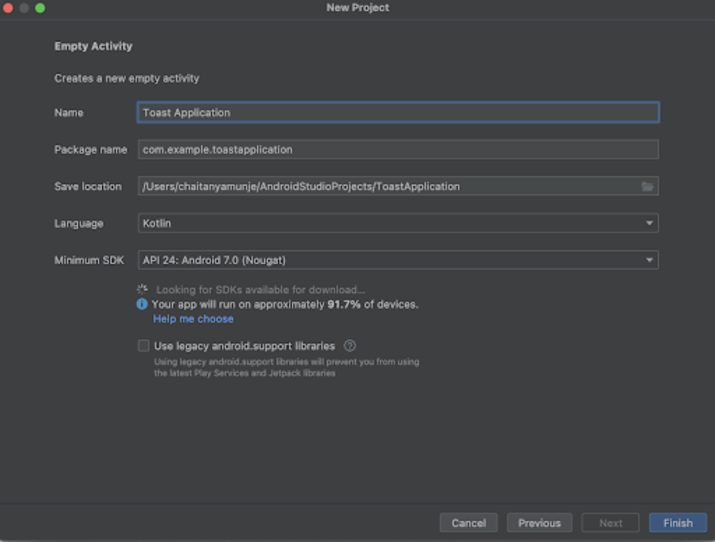
在这个屏幕中,我们只需指定项目名称。然后包名将自动生成。
注意:确保选择语言为 Java。
指定所有详细信息后,点击“完成”以创建一个新的 Android Studio 项目。
项目创建完成后,我们将看到两个打开的文件,即 activity_main.xml 和 MainActivity.java 文件。
步骤 2:使用 activity_main.xml
导航到 activity_main.xml。如果此文件不可见,要打开此文件,在左侧窗格中导航到 app > res > layout > activity_main.xml 以打开此文件。打开此文件后,将以下代码添加到其中。代码中添加了注释以详细了解。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> <!-- text view to displaying heading of application --> <TextView android:id="@+id/idTVHeading" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="100dp" android:text="Read SMS in Android" android:textAlignment="center" android:textColor="@color/black" android:textSize="20sp" android:textStyle="bold" /> <!-- on below line creating a text view for displaying the sms header --> <TextView android:id="@+id/idTVNumber" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idTVHeading" android:layout_centerHorizontal="true" android:layout_margin="10dp" android:padding="4dp" android:text="NUmber" android:textAlignment="center" android:textColor="@color/black" android:textSize="18sp" /> <!-- on below line creating a text view for displaying the sms body --> <TextView android:id="@+id/idTVSMSBody" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idTVNumber" android:layout_centerHorizontal="true" android:layout_margin="10dp" android:padding="4dp" android:text="SMS Body" android:textAlignment="center" android:textColor="@color/black" android:textSize="18sp" /> <!-- on below line creating a text view for displaying the date on which sms have recieved. --> <TextView android:id="@+id/idTVSMSDate" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idTVSMSBody" android:layout_centerHorizontal="true" android:layout_margin="10dp" android:padding="4dp" android:text="SMS Date" android:textAlignment="center" android:textColor="@color/black" android:textSize="18sp" /> </RelativeLayout>
说明:在上面的代码中,我们创建一个根布局作为相对布局。在此布局内,我们创建一个 TextView 用于显示应用程序标题。然后,我们创建另外三个 TextView,第一个 TextView 显示发件人姓名,第二个 TextView 显示发件人的短信正文,第三个 TextView 显示接收短信的日期。
步骤 3:在 AndroidManifest.xml 文件中添加权限
导航到 app > AndroidManifest.xml 文件,并在 manifest 标签中添加以下权限以读取短信。
<uses-permission android:name="android.permission.READ_SMS" />
步骤 4:使用 MainActivity.java 文件
导航到 MainActivity.java。如果此文件不可见,要打开此文件,在左侧窗格中导航到 app > res > layout > MainActivity.java 以打开此文件。打开此文件后,将以下代码添加到其中。代码中添加了注释以详细了解。
package com.example.java_test_application; import android.app.PendingIntent; import android.content.ContentResolver; import android.content.Intent; import android.content.IntentSender; import android.database.Cursor; import android.net.Uri; import android.os.Bundle; import android.provider.Telephony; import android.text.TextUtils; import android.util.Log; import android.util.Patterns; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import androidx.annotation.NonNull; import androidx.annotation.Nullable; import androidx.appcompat.app.AppCompatActivity; import com.google.android.gms.auth.api.Auth; import com.google.android.gms.auth.api.credentials.Credential; import com.google.android.gms.auth.api.credentials.CredentialPickerConfig; import com.google.android.gms.auth.api.credentials.CredentialRequest; import com.google.android.gms.auth.api.credentials.CredentialRequestResult; import com.google.android.gms.auth.api.credentials.Credentials; import com.google.android.gms.auth.api.credentials.CredentialsClient; import com.google.android.gms.auth.api.credentials.CredentialsOptions; import com.google.android.gms.auth.api.credentials.HintRequest; import com.google.android.gms.auth.api.credentials.IdentityProviders; import com.google.android.gms.auth.api.signin.GoogleSignIn; import com.google.android.gms.auth.api.signin.GoogleSignInAccount; import com.google.android.gms.auth.api.signin.GoogleSignInClient; import com.google.android.gms.auth.api.signin.GoogleSignInOptions; import com.google.android.gms.common.ConnectionResult; import com.google.android.gms.common.api.CommonStatusCodes; import com.google.android.gms.common.api.GoogleApiClient; import com.google.android.gms.common.api.ResolvableApiException; import com.google.android.gms.common.api.ResultCallback; import com.google.android.gms.common.api.Status; import com.google.android.gms.tasks.OnCompleteListener; import com.google.android.gms.tasks.Task; import org.w3c.dom.Text; import java.util.Date; public class MainActivity extends AppCompatActivity { // creating variables for text view on below line for displaying sender name, body of the message and the date on which message is received. private TextView numberTV, bodyTV, dateTV; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // initializing variables for text view on below line. numberTV = findViewById(R.id.idTVNumber); bodyTV = findViewById(R.id.idTVSMSBody); dateTV = findViewById(R.id.idTVSMSDate); // creating a variable for content resolver on below line. ContentResolver cr = getApplicationContext().getContentResolver(); // creating a variable for cursor on below line and setting uri as sms uri. Cursor c = cr.query(Telephony.Sms.CONTENT_URI, null, null, null, null); // on below line checking if the cursor is not null. if (c != null) { // on below line moving to the first position. if (c.moveToFirst()) { // on below line extracting the sms data from the cursor. String smsDate = c.getString(c.getColumnIndexOrThrow(Telephony.Sms.DATE)); // on below line extracting the sender name/ number from cursor. . String number = c.getString(c.getColumnIndexOrThrow(Telephony.Sms.ADDRESS)); // on below line extracting the body of the sms from the cursor. String body = c.getString(c.getColumnIndexOrThrow(Telephony.Sms.BODY)); // on below line converting date in date format. Date dateFormat = new Date(Long.valueOf(smsDate)); // on below line moving cursor to next position. c.moveToNext(); // on below line setting data from the string to our text views. numberTV.setText("Sender is : " + number); bodyTV.setText("Body is :
" + body); dateTV.setText(dateFormat.toString()); } // on below line closing the cursor. c.close(); } else { // on below line displaying toast message if there is no sms present in the device. Toast.makeText(this, "No message to show!", Toast.LENGTH_SHORT).show(); } } }
说明:在上面的代码中,首先我们为 TextView 创建变量。现在我们将看到 onCreate 方法。这是每个 Android 应用程序的默认方法。当应用程序视图创建时,将调用此方法。在此方法内,我们设置内容视图,即名为 activity_main.xml 的布局文件,以从该文件设置 UI。在 onCreate 方法内,我们使用我们在 activity_main.xml 文件中给出的 id 初始化 button 变量。然后,我们为内容解析器创建一个变量。然后,我们创建并初始化游标变量,方法是将短信查询和其他参数作为 null 传递。然后,我们检查游标是否不为 null。在这种情况下,我们将游标移动到第一个位置。在此方法内,我们提取短信的不同部分,例如发件人姓名、短信正文以及接收短信的日期。获取所有数据后,我们将这些数据设置为我们创建的 TextView。然后,我们关闭游标。如果游标为 null,这意味着收件箱为空,在这种情况下,我们将显示一条吐司消息,提示“没有短信显示”。
添加上述代码后,我们只需点击顶部栏中的绿色图标即可在移动设备上运行我们的应用程序。
注意 - 确保您已连接到您的真实设备或模拟器。
输出
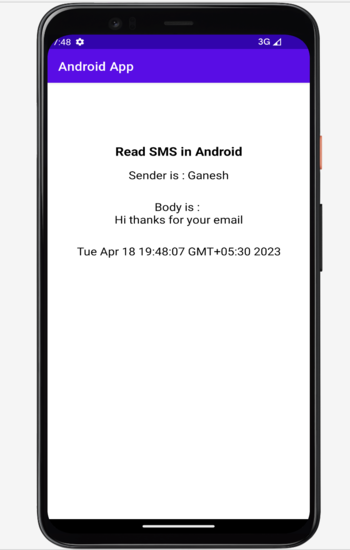
结论
在本文中,我们了解了如何在 Android 中以编程方式读取设备上的短信。