如何使用 tkinter 将按钮移到其父容器外部?
虽然 Tkinter 在创建动态应用程序方面表现出色,但在尝试操作小部件的位置时会出现一些挑战。在本教程中,我们将解释一种高级的 Tkinter 技术,并展示如何将按钮移动到其父容器的外部。
了解 Tkinter 层次结构
Tkinter 遵循一种层次结构,其中小部件嵌套在其父小部件中。小部件的位置和可见性受其父容器边界的限制。当尝试将小部件移动到其父容器区域外部时,此限制可能是一个障碍。但是,通过利用顶级窗口,我们可以创建一个解决方法来克服此限制。
创建简单的 Tkinter 应用程序
让我们从创建一个基本 Tkinter 应用程序开始,该应用程序包含一个用作我们起点的按钮。然后,我们将探讨如何将此按钮移动到其父容器的边界之外。
示例
import tkinter as tk # Defining the move_button_outside function def move_button_outside(): # Create a new Toplevel window toplevel = tk.Toplevel(root) toplevel.title("Outside Button") # Create a button in the Toplevel window outside_button = tk.Button(toplevel, text="Outside Button") outside_button.pack() # Move the Toplevel window to a specific position toplevel.geometry("+400+300") # Create the main Tkinter window root = tk.Tk() root.title("Main Window") root.geometry("720x250") # Create a button in the main window inside_button = tk.Button(root, text="Inside Button") inside_button.pack() # Create a button to move the outside button move_button = tk.Button(root, text="Move Outside Button", command=move_button_outside) move_button.pack() # Run the Tkinter event loop root.mainloop()
在上面的示例中,我们执行了以下操作:
我们导入 Tkinter 模块并将其简称为“tk”。
定义了 move_button_outside 函数来创建一个新的顶级窗口并在其中放置一个按钮。
主 Tkinter 窗口 (root) 包含一个“内部按钮”和一个“移到外部按钮”,后者触发 move_button_outside 函数。
输出
运行此代码后,您将获得以下输出窗口:
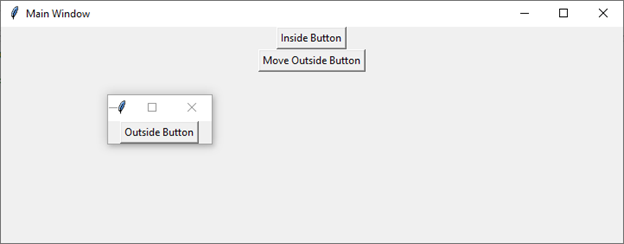
使用顶级窗口
将小部件移动到其父容器外部的关键是使用顶级窗口。这些窗口充当独立的容器,允许其中的小部件存在于主窗口的限制之外。在我们的示例中,单击“移到外部按钮”将创建一个包含“外部按钮”的顶级窗口。
def move_button_outside(): # Create a new Toplevel window toplevel = tk.Toplevel(root) toplevel.title("Outside Button") # Create a button in the Toplevel window outside_button = tk.Button(toplevel, text="Outside Button") outside_button.pack() # Move the Toplevel window to a specific position toplevel.geometry("+400+100") # Adjust the coordinates as needed
在 move_button_outside 函数中,创建一个新的顶级窗口,并在其中放置一个按钮。geometry 方法用于设置顶级窗口的位置,有效地将其放置在主窗口外部。geometry 方法中指定的坐标可以调整以达到所需的位置。
结论
Tkinter 中的顶级窗口为将小部件移动到其父容器边界之外提供了一种强大的解决方法。这种高级技术能够创建动态且视觉上引人注目的用户界面。通过将小部件策略性地放置在独立的容器(如顶级窗口)中,开发人员可以增强整体用户体验。