如何使用Python在OpenCV中调整图像大小?
OpenCV 提供了函数 cv2.resize() 来调整图像大小。在OpenCV中,调整大小被称为缩放。我们可以通过指定图像大小或缩放因子来调整图像大小。当我们指定缩放因子时,纵横比会得到保留。
cv2.resize() 函数使用了不同的插值方法:
cv2.INTER_AREA - 用于缩小图像。
cv2.INTER_CUBIC - 速度较慢,用于放大。
cv2.INTER_LINEAR - 用于放大。它是所有调整大小目的的默认值。
步骤
您可以使用以下步骤来调整图像大小:
导入所需的库。在以下所有Python示例中,所需的Python库是OpenCV和Matplotlib。请确保您已经安装了它们。
import cv2 import matplotlib.pyplot as plt
使用cv2.imread()函数读取图像。使用图像类型(.jpg或.png)指定完整的图像路径。
img = cv2.imread('birds.jpg')
传递new_size或缩放因子fx和fy以及插值来调整图像大小。fx和fy分别是宽度和高度的缩放因子。
resize_img = cv2.resize(img, new_size) resize_img = cv2.resize(img,(0, 0),fx=0.5, fy=0.7, interpolation = cv2.INTER_AREA)
显示调整大小后的图像。
plt.imshow(resize_img)
让我们借助一些Python示例来了解不同的图像大小调整选项。
在以下示例中,我们将使用此图像作为输入文件:
示例1
在下面的Python程序中,我们将输入图像调整为new_size = 450, 340)。这里宽度=450,高度=340。
import cv2 import matplotlib.pyplot as plt img = cv2.imread('birds.jpg') h, w, c = img.shape print(f"Height and width of original image: {h}, {w}" ) # resize the image new_size = (450, 340) # new_size=(width, height) print(f"New height and width: {new_size[1]}, {new_size[0]}" ) resize_img = cv2.resize(img, new_size) # Convert the images from BGR to RGB img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) resize_img = cv2.cvtColor(resize_img, cv2.COLOR_BGR2RGB) plt.subplot(121),plt.imshow(img), plt.title("Original Image") plt.subplot(122), plt.imshow(resize_img), plt.title("Resized Image") plt.show()
输出
运行上述程序后,将产生以下输出:
Height and width of original image: 465, 700 New height and width of original image: 340, 450
并且它将显示以下输出窗口,显示原始图像和调整大小后的图像。
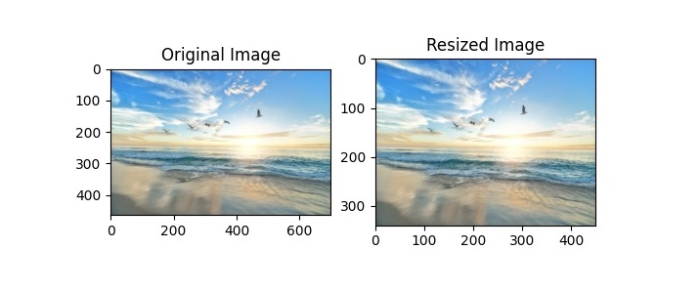
示例2
在下面的Python程序中,我们将使用不同的缩放因子和插值来调整输入图像的大小。
# import the required libraries import cv2 import matplotlib.pyplot as plt # read the input image img = cv2.imread('birds.jpg') # resize the image using different interpolations resize_cubic = cv2.resize(img,(0, 0),fx=2, fy=2, interpolation = cv2.INTER_CUBIC) resize_area = cv2.resize(img,(0, 0),fx=0.5, fy=0.7, interpolation = cv2.INTER_AREA) resize_linear = cv2.resize(img,(0, 0),fx=2, fy=2, interpolation = cv2.INTER_LINEAR) # display the original and resized images plt.subplot(221),plt.imshow(img), plt.title("Original Image") plt.subplot(222), plt.imshow(resize_cubic), plt.title("Interpolation Cubic") plt.subplot(223), plt.imshow(resize_area), plt.title("Interpolation Area") plt.subplot(224), plt.imshow(resize_linear), plt.title("Interpolation Linear") plt.show()
输出
运行上述程序后,将产生以下输出:
广告