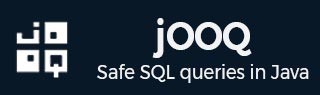
jOOQ - DML语句
jOOQ支持各种SQL语句。这些SQL语句由DSLContext对象创建。它将根据查询类型返回结果。在本教程中,我们将学习DML语句以及如何在jOOQ中创建和执行它们。
什么是DML?
DML代表数据操纵语言 (Data Manipulation Language)。如果您熟悉SQL,您可能已经了解它的命令。DML是一组SQL命令,允许您修改给定数据库中的数据。最常见的DML操作是
SELECT:此操作将从指定的表中检索数据。
INSERT:它将新数据插入到给定的表中。
UPDATE:用于修改表中现有数据。
DELETE:它从指定的表中删除数据。
创建DML语句的jOOQ方法
在jOOQ中,DSL类提供了一组用于创建DML语句的方法,如下表所示:
序号 | 方法和描述 |
---|---|
1. | insertInto(Table<Record> table) 此方法用于启动对给定表的INSERT语句。 |
2. | set(Field<T> field, T value) 此方法用于在插入和更新记录时设置字段的值。 |
3. | values(Object... values) 它用于定义要插入表中的值。 |
4. | update(Table<Record> table) 此方法用于启动对指定表的UPDATE语句。 |
5. | deleteFrom(Table<Record> table) 它用于启动对指定表的DELETE语句。 |
6. | select(Field<?>... fields) 此方法用于使用指定的字段创建SELECT查询。 |
7. | from(Table<?>... tables) 此方法用于指定要从中选择数据的表。 |
jOOQ中DML语句示例
在此示例中,我们将执行INSERT操作以添加新行。假设现有的表employee存储以下记录:
ID | 姓名 | 职位 |
---|---|---|
1 | Aman | 技术撰写人 |
4 | Vivek | 开发者 |
要使用jOOQ插入新记录,请在src -> main -> java -> com.example.demo内部创建一个包。com.example.demo文件夹的名称将取决于您的项目名称。在这个包内创建一个Java类。我们命名了包service和Java类EmployeeService.java。您可以随意命名。
将以下代码片段复制并粘贴到EmployeeService.java文件中。(此处省略代码示例)
package com.example.demo.service; import org.jooq.*; import org.jooq.Record; import org.jooq.impl.DSL; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import javax.sql.DataSource; @Service public class EmployeeService { private final DataSource dataSource; @Autowired public EmployeeService(DataSource dataSource) { this.dataSource = dataSource; } public void run() { // Create a DSLContext using the DataSource DSLContext create = DSL.using(dataSource, SQLDialect.MYSQL); // Manually define the table and columns Table<?> employee = DSL.table("employee"); Field<Integer> id = DSL.field("id", Integer.class); Field<String> name = DSL.field("name", String.class); Field<String> jobTitle = DSL.field("job_title", String.class); // Insert a new employee record into the table create.insertInto(employee, id, name, jobTitle) .values(2, "Shriansh", "Software Engineer") .execute(); // Fetch the values from the employee table Result<Record3<Integer, String, String>> result = create.select(id, name, jobTitle) .from(employee) .fetch(); // Print the results for (Record record : result) { Integer employeeId = record.get(id); String employeeName = record.get(name); String employeeJobTitle = record.get(jobTitle); System.out.println("ID: " + employeeId + ", Name: " + employeeName + ", Job Title: " + employeeJobTitle); } } }
现在,导航到com.example.demo文件夹内的DemoApplication.java文件。并编写以下代码:(此处省略代码示例)
package com.example.demo; import com.example.demo.service.EmployeeService; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication implements CommandLineRunner { private final EmployeeService demoApplication; public DemoApplication(EmployeeService demoApplication) { this.demoApplication = demoApplication; } public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } @Override public void run(String... args) throws Exception { demoApplication.run(); } }
运行此代码时,它将打印更新后的表:(此处省略代码示例)
ID: 1, Name: Aman, Job Title: Technical Writer ID: 2, Name: Shriansh, Job Title: Software Engineer ID: 4, Name: Vivek, Job Title: Developer