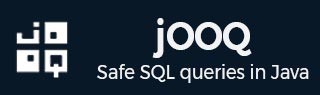
jOOQ - 事务语句
什么是事务?
事务是一系列一个或多个操作,作为一个单一的工 作单元执行。如果任何任务失败,则事务失败。事务必须满足 ACID 属性,它们是:
原子性:事务是原子的,这意味着要么所有操作都成功,要么都不成功。
一致性:它确保数据库在成功提交的事务后能够正确地从一个状态转换到另一个状态。
隔离性:事务彼此隔离。
持久性:一旦事务提交,即使系统崩溃,更改也会持久保存。
事务操作
常见的事务操作包括:
BEGIN:启动一个新事务。
COMMIT:使更改永久生效。
ROLLBACK:撤销更改
jOOQ 中的事务语句
在 jOOQ 中,DSL 类的以下方法用于创建事务语句:
序号 | 方法和描述 |
---|---|
1. | transaction(Configuration) 此方法将多个数据库操作组合在一个事务块中。 |
2. | setTransactionIsolation(int isolationLevel) 它用于设置当前事务的隔离级别。 |
3. | commit() 此方法用于提交当前事务。 |
4. | rollback() 它将撤消事务期间所做的任何更改。 |
jOOQ 中事务语句的示例
在此示例中,我们将把ALTER TABLE操作和INSERT操作组合在一起。我们将使用以下表进行此操作:
排名 | 姓名 | 职位 |
---|---|---|
1 | Aman | 技术作家 |
2 | Shriansh | 软件工程师 |
4 | Vivek | 开发者 |
首先在“src -> main -> java -> com.example.demo”内部创建一个包。“com.example.demo”文件夹的名称将取决于你的项目名称。在这个包内创建一个 Java 类。我们已将包命名为“service”,并将 Java 类命名为“EmployeeService.java”。你可以随意命名。
在 jOOQ 中,我们使用alterTable()方法修改表结构,使用renameColumn()方法重命名列。要将数据插入表中,我们使用insertInto()方法。将以下代码片段复制并粘贴到“EmployeeService.java”文件中。
package com.example.demo.service; import org.jooq.*; import org.jooq.Record; import org.jooq.impl.DSL; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import javax.sql.DataSource; @Service public class EmployeeService { private final DataSource dataSource; @Autowired public EmployeeService(DataSource dataSource) { this.dataSource = dataSource; } public void run() { // Create a DSLContext using the DataSource DSLContext create = DSL.using(dataSource, SQLDialect.MYSQL); // Wrap the operations inside a transaction create.transaction(configuration -> { // Create a DSLContext within the transaction context DSLContext ctx = DSL.using(configuration); // Manually define the table and columns Table<?> employee = DSL.table("employee"); Field<Integer> id = DSL.field("id", Integer.class); Field<String> name = DSL.field("name", String.class); Field<String> jobTitle = DSL.field("job_title", String.class); // Rename the column 'id' to 'rank' ctx.alterTable("employee") .renameColumn("rank").to("id") .execute(); // Insert a new employee record into the table ctx.insertInto(employee, id, name, jobTitle) .values(3, "Ansh", "Software Engineer") .execute(); // Fetch the values from the employee table Result<Record3<Integer, String, String>> result = ctx.select(id, name, jobTitle) .from(employee) .fetch(); // Print the results for (Record record : result) { Integer employeeId = record.get(id); String employeeName = record.get(name); String employeeJobTitle = record.get(jobTitle); System.out.println("ID: " + employeeId + ", Name: " + employeeName + ", Job Title: " + employeeJobTitle); } }); } }
现在,导航到“com.example.demo”文件夹内的“DemoApplication.java”文件。然后,编写如下代码:
package com.example.demo; import com.example.demo.service.EmployeeService; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication implements CommandLineRunner { private final EmployeeService demoApplication; public DemoApplication(EmployeeService demoApplication) { this.demoApplication = demoApplication; } public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } @Override public void run(String... args) throws Exception { demoApplication.run(); } }
运行此代码时,它将把“Rank”列更改为“id”,并将另一行插入到指定的表中:
ID: 1, Name: Aman, Job Title: Technical Writer ID: 2, Name: Shriansh, Job Title: Software Engineer ID: 3, Name: Ansh, Job Title: Software Engineer ID: 4, Name: Vivek, Job Title: Developer