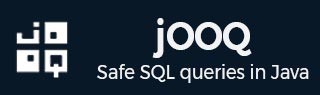
jOOQ - DSLContext API
jOOQ 中的 DSLContext 是什么?
DSLContext 是一个接口,可在执行查询时配置 jOOQ 的行为。它的引用或对象管理与数据库的实际交互。此上下文 DSL 是您的 jOOQ 代码访问与查询执行相关的类和功能的主要入口点。
DSL 用于创建 SQL 查询,而 DSLContext 用于执行这些查询并与数据库交互。
如何创建一个 DSLContext 对象?
您可以借助 DSL 类的组成部分 **using()** 方法创建 DSLContext 的对象或引用。在此方法中,您可以传递预先存在的配置或即席参数(如连接和方言)来创建 DSLContext 对象。
// Create it from a pre-existing configuration DSLContext create = DSL.using(configuration); // Create it from ad-hoc arguments DSLContext create = DSL.using(connection, dialect);
jOOQ DSLContext 示例
让我们看看如何使用 DSLContext API 执行读取表中值的查询。假设表名为 **employee**,并包含以下值 −
ID | 姓名 | 职务 |
---|---|---|
1 | 阿曼 | 技术编写员 |
4 | 维维克 | 开发人员 |
要使用 jOOQ 获取此记录,请在**src -> main -> java -> com.example.demo** 内创建一个包。**com.example.demo** 文件夹的名称取决于您的项目名称。在此包内创建一个 Java 类。我们已将包命名为 **service**,将 Java 类命名为 **EmployeeService.java**。您可以根据自己的喜好指定任何名称。
将以下代码段复制并粘贴到 **EmployeeService.java** 文件中。
package com.example.demo.service; import org.jooq.*; import org.jooq.Record; import org.jooq.impl.DSL; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import javax.sql.DataSource; @Service public class EmployeeService { private final DataSource dataSource; @Autowired public EmployeeService(DataSource dataSource) { this.dataSource = dataSource; } public void run() { // Create a DSLContext using the DataSource DSLContext create = DSL.using(dataSource, SQLDialect.MYSQL); // Manually define the table and columns Table<?> employee = DSL.table("employee"); Field<Integer> id = DSL.field("id", Integer.class); Field<String> name = DSL.field("name", String.class); Field<String> jobTitle = DSL.field("job_title", String.class); // Fetch the values from the employee table Result<Record3<Integer, String, String>> result = create.select(id, name, jobTitle) .from(employee) .fetch(); // Print the results for (Record record : result) { Integer employeeId = record.get(id); String employeeName = record.get(name); String employeeJobTitle = record.get(jobTitle); System.out.println("ID: " + employeeId + ", Name: " + employeeName + ", Job Title: " + employeeJobTitle); } } }
现在,导航到 **com.example.demo** 文件夹中的 **DemoApplication.java** 文件。编写如下给出的代码 −
package com.example.demo; import com.example.demo.service.EmployeeService; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication implements CommandLineRunner { private final EmployeeService demoApplication; public DemoApplication(EmployeeService demoApplication) { this.demoApplication = demoApplication; } public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } @Override public void run(String... args) throws Exception { demoApplication.run(); } }
当您运行此代码时,它将调用 **EmployeeService.java** 文件并打印以下结果 −
ID: 1, Name: Aman, Job Title: Technical Writer ID: 4, Name: Vivek, Job Title: Developer
广告