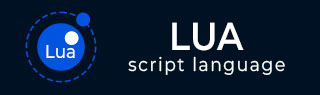
- Lua 教程
- Lua - 首页
- Lua 基础
- Lua - 概述
- Lua - 环境
- Lua - 基本语法
- Lua - 注释
- Lua - 打印 Hello World
- Lua - 变量
- Lua - 数据类型
- Lua - 运算符
- Lua - 循环
- Lua - 决策
- Lua - 函数
- Lua - 日期和时间
- Lua 数组
- Lua - 数组
- Lua - 多维数组
- Lua - 数组长度
- Lua - 遍历数组
- Lua 迭代器
- Lua - 迭代器
- Lua 列表
- Lua - 在列表中搜索
- Lua 模块
- Lua - 模块
- Lua - 命名空间
- Lua 元表
- Lua - 元表
- Lua 协程
- Lua - 协程
- Lua 文件处理
- Lua - 文件 I/O
- Lua 库
- Lua - 标准库
- Lua - 数学库
- Lua - 操作系统功能
- Lua 有用资源
- Lua - 快速指南
- Lua - 有用资源
- Lua - 讨论
Lua - if...else 语句
一个if语句后面可以跟着一个可选的else语句,当布尔表达式为假时,该语句将执行。
语法
Lua 编程语言中if...else语句的语法如下:
if(boolean_expression) then --[ statement(s) will execute if the boolean expression is true --] else --[ statement(s) will execute if the boolean expression is false --] end
如果布尔表达式计算结果为true,则将执行if 代码块,否则将执行else 代码块。
Lua 编程语言将布尔true和非nil值的任何组合视为true,如果它是布尔false或nil,则将其视为false值。需要注意的是,在 Lua 中,零将被视为 true。
流程图
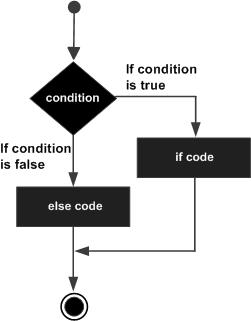
示例
在这个例子中,我们展示了 if else 语句的使用。我们创建了一个变量 a 并将其初始化为 100。然后在 if 语句中,我们用 20 检查 x。由于 if 语句为假,所以执行 else 代码块中的语句。
--[ local variable definition --] a = 100; --[ check the boolean condition --] if( a < 20 ) then --[ if condition is true then print the following --] print("a is less than 20" ) else --[ if condition is false then print the following --] print("a is not less than 20" ) end print("value of a is :", a)
输出
当你构建并运行以上代码时,它会产生以下结果。
a is not less than 20 value of a is : 100
if...else if...else 语句
一个if语句后面可以跟着一个可选的else if...else语句,这对于使用单个 if...else if 语句测试各种条件非常有用。
在使用 if、else if、else 语句时,需要注意以下几点:
一个if可以有零个或一个 else,并且它必须放在任何 else if 之后。
一个if可以有零到多个 else if,并且它们必须放在 else 之前。
一旦一个else if成功,就不会再测试任何剩下的 else if 或 else。
语法
Lua 编程语言中if...else if...else语句的语法如下:
if(boolean_expression 1) then --[ Executes when the boolean expression 1 is true --] elseif( boolean_expression 2) --[ Executes when the boolean expression 2 is true --] elseif( boolean_expression 3) --[ Executes when the boolean expression 3 is true --] else --[ executes when the none of the above condition is true --] end
示例
在这个例子中,我们展示了 if...else if...else 语句的使用。我们创建了一个变量 a 并将其初始化为 10。然后在 if 语句中,我们用 10 检查 a。由于 if 语句为假,控制权跳转到 else if 语句,检查另一个与 a 的值,依此类推。
--[ local variable definition --] a = 100 --[ check the boolean condition --] if( a == 10 ) then --[ if condition is true then print the following --] print("Value of a is 10" ) elseif( a == 20 ) then --[ if else if condition is true --] print("Value of a is 20" ) elseif( a == 30 ) then --[ if else if condition is true --] print("Value of a is 30" ) else --[ if none of the conditions is true --] print("None of the values is matching" ) end print("Exact value of a is: ", a )
输出
当你构建并运行以上代码时,它会产生以下结果。
None of the values is matching Exact value of a is: 100
嵌套 if-else 语句
当在给定条件为真的情况下需要检查其他条件时,嵌套 if else 语句用于做出更好的决策。在嵌套 if else 语句中,你可以有一个 if-else 语句块在另一个 if(或 else)块中。
语法
Lua 编程语言中嵌套 if-else语句的语法如下:
if(condition1) then -- code block if(condition2) then -- code block end end
示例
以下示例使用嵌套 if..else 语句找出三个数中最大的数。
x, y, z = 10, 20, 30 if (x >= y) then if(x >= z) then print(x, " is the largest.") else print(z, " is the largest.") end else if(y >= z) then print(y, " is the largest.") else print(z, " is the largest.") end end
输出
当你构建并运行以上代码时,它会产生以下结果。
30 is the largest.