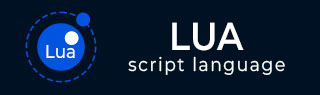
- Lua 教程
- Lua - 首页
- Lua 基础
- Lua - 概述
- Lua - 环境
- Lua - 基本语法
- Lua - 注释
- Lua - 打印 Hello World
- Lua - 变量
- Lua - 数据类型
- Lua - 运算符
- Lua - 循环
- Lua - 决策
- Lua - 函数
- Lua - 日期和时间
- Lua 数组
- Lua - 数组
- Lua - 多维数组
- Lua - 数组长度
- Lua - 迭代数组
- Lua 迭代器
- Lua - 迭代器
- Lua 列表
- Lua - 在列表中搜索
- Lua 模块
- Lua - 模块
- Lua - 命名空间
- Lua 元表
- Lua - 元表
- Lua 协程
- Lua - 协程
- Lua 文件处理
- Lua - 文件 I/O
- Lua 库
- Lua - 标准库
- Lua - 数学库
- Lua - 操作系统功能
- Lua 有用资源
- Lua - 快速指南
- Lua - 有用资源
- Lua - 讨论
Lua - 检查字符串是否为空或空值
在 Lua 中,我们可以通过多种方式检查字符串是否为空或空值/Nil。
使用 string.len(str) 方法− 使用 string.len() 方法,我们可以将字符串作为参数传递并获取所需的长度。如果长度为零,则字符串为空。
使用 == 运算符− 我们可以将字符串与 '' 进行比较以检查它是否为空。
使用 Nil 检查− 我们可以使用 == 运算符将字符串与 Nil 进行比较以检查它是否为空。
以下示例显示了上述检查。
示例 - string.len() 方法
下面显示了使用 string.len 方法的示例。
main.lua
-- text is nil -- we can check length of string if not text or string.len(text) == 0 then print(text,"is empty.") else print(text,"is not empty.") end -- text is empty text = '' if not text or string.len(text) == 0 then print(text,"is empty.") else print(text,"is not empty.") end -- text is mot empty text = 'ABC' if not text or string.len(text) == 0 then print(text,"is empty.") else print(text,"is not empty.") end
输出
运行上述程序时,我们将获得以下输出。
nil is empty. is empty. ABC is not empty.
示例 - 使用 == 运算符
下面显示了使用 == 运算符的示例。
main.lua
-- text is nil -- check if string is empty if text == '' then print(text,"is empty.") else print(text,"is not empty.") end text = '' -- empty string if text == '' then print(text,"is empty.") else print(text,"is not empty.") end text = 'ABC' if text == '' then print(text,"is empty.") else print(text,"is not empty.") end
输出
运行上述程序时,我们将获得以下输出。
nil is empty. is empty. ABC is not empty.
示例 - Nil 检查
下面显示了使用 string.len 方法的示例。
main.lua
-- text is nil -- check if string is nil or empty if text == nil or text == '' then print(text,"is empty.") else print(text,"is not empty.") end text = '' -- nil or empty string is considered as true if not text or text == '' then print(text,"is empty.") else print(text,"is not empty.") end text = 'ABC' if not text or text == '' then print(text,"is empty.") else print(text,"is not empty.") end
输出
运行上述程序时,我们将获得以下输出。
nil is empty. is empty. ABC is not empty.
广告