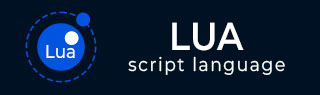
- Lua 教程
- Lua - 首页
- Lua 基础
- Lua - 概述
- Lua - 环境
- Lua - 基本语法
- Lua - 注释
- Lua - 打印 Hello World
- Lua - 变量
- Lua - 数据类型
- Lua - 运算符
- Lua - 循环
- Lua - 决策
- Lua - 函数
- Lua - 日期和时间
- Lua 数组
- Lua - 数组
- Lua - 多维数组
- Lua - 数组长度
- Lua - 迭代数组
- Lua 迭代器
- Lua - 迭代器
- Lua 列表
- Lua - 在列表中搜索
- Lua 模块
- Lua - 模块
- Lua - 命名空间
- Lua 元表
- Lua - 元表
- Lua 协程
- Lua - 协程
- Lua 文件处理
- Lua - 文件 I/O
- Lua 库
- Lua - 标准库
- Lua - 数学库
- Lua - 操作系统功能
- Lua 有用资源
- Lua - 快速指南
- Lua - 有用资源
- Lua - 讨论
Lua - 文件 I/O
I/O 库用于在 Lua 中读取和操作文件。Lua 中有两种文件操作,即隐式文件描述符和显式文件描述符。
对于以下示例,我们将使用如下所示的示例文件 test.lua。
test.lua
-- sample test.lua -- sample2 test.lua
简单的文件打开操作使用以下语句。
file = io.open (filename [, mode])
各种文件模式列在以下表格中。
序号 | 模式及描述 |
---|---|
1 | "r" 只读模式,也是默认模式,打开现有的文件。 |
2 | "w" 启用写入模式,覆盖现有文件或创建新文件。 |
3 | "a" 追加模式,打开现有文件或创建新文件以进行追加。 |
4 | "r+" 现有文件的读写模式。 |
5 | "w+" 如果文件存在,则删除所有现有数据,或创建具有读写权限的新文件。 |
6 | "a+" 启用读模式的追加模式,打开现有文件或创建新文件。 |
隐式文件描述符
隐式文件描述符使用标准输入/输出模式或使用单个输入和单个输出文件。使用隐式文件描述符的示例如下所示。
main.lua
-- Opens a file in read file = io.open("test.lua", "r") -- sets the default input file as test.lua io.input(file) -- prints the first line of the file print(io.read()) -- closes the open file io.close(file) -- Opens a file in append mode file = io.open("test.lua", "a") -- sets the default output file as test.lua io.output(file) -- appends a word test to the last line of the file io.write("-- End of the test.lua file") -- closes the open file io.close(file)
输出
运行程序时,您将获得 test.lua 文件第一行的输出。对于我们的程序,我们得到了以下输出。
-- Sample test.lua
这是 test.lua 文件中语句的第一行。此外," -- End of the test.lua file" 将追加到 test.lua 代码的最后一行。
在上面的示例中,您可以看到隐式描述符如何使用 io."x" 方法与文件系统交互。上面的示例使用 io.read() 而不带可选参数。可选参数可以是以下任何一个。
序号 | 模式及描述 |
---|---|
1 | "*n" 从当前文件位置读取,如果文件位置存在数字则返回该数字,否则返回 nil。 |
2 | "*a" 从当前文件位置返回文件的全部内容。 |
3 | "*l" 从当前文件位置读取行,并将文件位置移动到下一行。 |
4 | 数字 读取函数中指定的字节数。 |
其他常见的 I/O 方法包括:
io.tmpfile() - 返回一个临时文件,用于读取和写入,程序退出后将被删除。
io.type(file) - 根据输入文件返回文件、已关闭文件或 nil。
io.flush() - 清除默认输出缓冲区。
io.lines(可选文件名) - 提供一个通用的 for 循环迭代器,循环遍历文件并在最后关闭文件,如果提供了文件名或使用了默认文件并且在循环结束时未关闭。
显式文件描述符
我们经常使用显式文件描述符,它允许我们同时操作多个文件。这些函数与隐式文件描述符非常相似。在这里,我们使用 file:function_name 代替 io.function_name。以下示例显示了与相同隐式文件描述符示例相同的文件版本。
main.lua
-- Opens a file in read mode file = io.open("test.lua", "r") -- prints the first line of the file print(file:read()) -- closes the opened file file:close() -- Opens a file in append mode file = io.open("test.lua", "a") -- appends a word test to the last line of the file file:write("--test") -- closes the open file file:close()
运行程序时,您将获得与隐式描述符示例类似的输出。
-- Sample test.lua
所有文件打开模式和外部描述符读取的参数与隐式文件描述符相同。
其他常见的文件方法包括:
file:seek(可选的 whence,可选的 offset) - Whence 参数是 "set"、"cur" 或 "end"。使用从文件开头更新的文件位置设置新的文件指针。此函数中的偏移量为零基。如果第一个参数为 "set",则偏移量从文件开头测量;如果为 "cur",则从文件中的当前位置测量;如果为 "end",则从文件末尾测量。默认参数值为 "cur" 和 0,因此可以通过不带参数地调用此函数来获取当前文件位置。
file:flush() - 清除默认输出缓冲区。
io.lines(可选文件名) - 提供一个通用的 for 循环迭代器,循环遍历文件并在最后关闭文件,如果提供了文件名或使用了默认文件并且在循环结束时未关闭。
使用 seek 方法的示例如下所示。它将光标从文件末尾之前的 25 个位置偏移。read 函数打印从 seek 位置开始的文件的其余部分。
main.lua
-- Opens a file in read file = io.open("test.lua", "r") file:seek("end",-5) print(file:read("*a")) -- closes the opened file file:close()
输出
您将获得一些类似于以下内容的输出。
--test
您可以尝试所有不同的模式和参数,以了解 Lua 文件操作的全部功能。