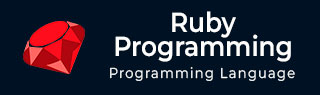
- Ruby 基础
- Ruby - 首页
- Ruby - 概述
- Ruby - 环境搭建
- Ruby - 语法
- Ruby - 类和对象
- Ruby - 变量
- Ruby - 运算符
- Ruby - 注释
- Ruby - IF...ELSE
- Ruby - 循环
- Ruby - 方法
- Ruby - 代码块
- Ruby - 模块
- Ruby - 字符串
- Ruby - 数组
- Ruby - 哈希表
- Ruby - 日期和时间
- Ruby - 范围
- Ruby - 迭代器
- Ruby - 文件 I/O
- Ruby - 异常
Ruby - 代码块
您已经了解了 Ruby 如何定义方法,您可以在其中放置多条语句,然后调用该方法。类似地,Ruby 有一个代码块的概念。
代码块由代码块组成。
您为代码块分配一个名称。
代码块中的代码始终用大括号({})括起来。
代码块总是从与代码块名称相同的函数中调用。这意味着如果您有一个名为test的代码块,那么您使用函数test来调用此代码块。
您可以使用yield语句调用代码块。
语法
block_name { statement1 statement2 .......... }
在这里,您将学习如何使用简单的yield语句调用代码块。您还将学习如何使用带有参数的yield语句来调用代码块。您将使用这两种类型的yield语句检查示例代码。
yield 语句
让我们来看一个 yield 语句的示例:
#!/usr/bin/ruby def test puts "You are in the method" yield puts "You are again back to the method" yield end test {puts "You are in the block"}
这将产生以下结果:
You are in the method You are in the block You are again back to the method You are in the block
您也可以使用 yield 语句传递参数。这是一个例子:
#!/usr/bin/ruby def test yield 5 puts "You are in the method test" yield 100 end test {|i| puts "You are in the block #{i}"}
这将产生以下结果:
You are in the block 5 You are in the method test You are in the block 100
在这里,yield语句后面跟着参数。您甚至可以传递多个参数。在代码块中,您在两个竖线 (||) 之间放置一个变量来接受参数。因此,在前面的代码中,yield 5 语句将值 5 作为参数传递给 test 代码块。
现在,看一下下面的语句:
test {|i| puts "You are in the block #{i}"}
在这里,值 5 接收在变量i中。现在,观察下面的puts语句:
puts "You are in the block #{i}"
这个puts语句的输出是:
You are in the block 5
如果您想传递多个参数,那么yield语句将变为:
yield a, b
而代码块是:
test {|a, b| statement}
参数将用逗号分隔。
代码块和方法
您已经了解了代码块和方法如何相互关联。您通常使用 yield 语句从与代码块同名的的方法中调用代码块。因此,您编写:
#!/usr/bin/ruby def test yield end test{ puts "Hello world"}
此示例是实现代码块的最简单方法。您可以使用yield语句调用 test 代码块。
但是,如果方法的最后一个参数前面带有 &,那么您可以将代码块传递给此方法,并且此代码块将被赋值给最后一个参数。如果 * 和 & 都存在于参数列表中,& 应该放在后面。
#!/usr/bin/ruby def test(&block) block.call end test { puts "Hello World!"}
这将产生以下结果:
Hello World!
BEGIN 和 END 代码块
每个 Ruby 源文件都可以声明要在加载文件时运行的代码块(BEGIN 代码块)以及在程序执行完毕后运行的代码块(END 代码块)。
#!/usr/bin/ruby BEGIN { # BEGIN block code puts "BEGIN code block" } END { # END block code puts "END code block" } # MAIN block code puts "MAIN code block"
程序可能包含多个 BEGIN 和 END 代码块。BEGIN 代码块按遇到的顺序执行。END 代码块按相反顺序执行。执行后,上述程序产生以下结果:
BEGIN code block MAIN code block END code block