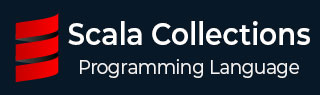
- Scala 集合教程
- Scala 集合 - 首页
- Scala 集合 - 概述
- Scala 集合 - 环境搭建
- Scala 集合 - 数组
- Scala 集合 - 数组
- Scala 集合 - 多维数组
- Scala 集合 - 使用 Range 创建数组
- Scala 集合 - ArrayBuffer
- Scala 集合 - 列表
- Scala 集合 - 列表
- Scala 集合 - ListBuffer
- Scala 集合 - ListSet
- Scala 集合 - 向量
- Scala 集合 - 集合
- Scala 集合 - Set
- Scala 集合 - BitSet
- Scala 集合 - HashSet
- Scala 集合 - TreeSet
- Scala 集合 - Map
- Scala 集合 - Map
- Scala 集合 - HashMap
- Scala 集合 - ListMap
- Scala 集合 - 其他
- Scala 集合 - 迭代器
- Scala 集合 - Option
- Scala 集合 - 队列
- Scala 集合 - 元组
- Scala 集合 - Seq
- Scala 集合 - 栈
- Scala 集合 - 流
- Scala 集合组合器方法
- Scala 集合 - drop
- Scala 集合 - dropWhile
- Scala 集合 - filter
- Scala 集合 - find
- Scala 集合 - flatMap
- Scala 集合 - flatten
- Scala 集合 - fold
- Scala 集合 - foldLeft
- Scala 集合 - foldRight
- Scala 集合 - map
- Scala 集合 - partition
- Scala 集合 - reduce
- Scala 集合 - scan
- Scala 集合 - zip
- Scala 集合有用资源
- Scala 集合 - 快速指南
- Scala 集合 - 有用资源
- Scala 集合 - 讨论
Scala 集合 - Set
Scala Set 是一个由相同类型且互不相同的元素组成的集合。换句话说,Set 是一个不包含重复元素的集合。Set 有两种类型:**不可变**和**可变**。不可变对象和可变对象的区别在于,不可变对象本身不能被修改。
默认情况下,Scala 使用不可变 Set。如果要使用可变 Set,则必须显式导入 **scala.collection.mutable.Set** 类。如果要在同一个集合中同时使用可变和不可变 Set,则可以继续将不可变 Set 称为 **Set**,而可变 Set 称为 **mutable.Set**。
以下是声明不可变 Set 的方法:
语法
// Empty set of integer type var s : Set[Int] = Set() // Set of integer type var s : Set[Int] = Set(1,3,5,7) or var s = Set(1,3,5,7)
定义空集合时,类型注解是必要的,因为系统需要为变量分配一个具体的类型。
Set 的基本操作
Set 的所有操作都可以用以下三种方法来表达:
序号 | 方法及描述 |
---|---|
1 |
head 此方法返回集合的第一个元素。 |
2 |
tail 此方法返回包含除第一个元素之外所有元素的集合。 |
3 |
isEmpty 如果集合为空,则此方法返回 true,否则返回 false。 |
尝试以下示例,其中显示了基本操作方法的使用:
示例
object Demo { def main(args: Array[String]) { val fruit = Set("apples", "oranges", "pears") val nums: Set[Int] = Set() println( "Head of fruit : " + fruit.head ) println( "Tail of fruit : " + fruit.tail ) println( "Check if fruit is empty : " + fruit.isEmpty ) println( "Check if nums is empty : " + nums.isEmpty ) } }
将上述程序保存为 **Demo.scala**。使用以下命令编译并执行此程序。
命令
\>scalac Demo.scala \>scala Demo
输出
Head of fruit : apples Tail of fruit : Set(oranges, pears) Check if fruit is empty : false Check if nums is empty : true
连接集合
可以使用 **++** 运算符或 **Set.++()** 方法来连接两个或多个集合,但在添加集合时会删除重复元素。
以下是连接两个集合的示例。
示例
object Demo { def main(args: Array[String]) { val fruit1 = Set("apples", "oranges", "pears") val fruit2 = Set("mangoes", "banana") // use two or more sets with ++ as operator var fruit = fruit1 ++ fruit2 println( "fruit1 ++ fruit2 : " + fruit ) // use two sets with ++ as method fruit = fruit1.++(fruit2) println( "fruit1.++(fruit2) : " + fruit ) } }
将上述程序保存为 **Demo.scala**。使用以下命令编译并执行此程序。
命令
\>scalac Demo.scala \>scala Demo
输出
fruit1 ++ fruit2 : Set(banana, apples, mangoes, pears, oranges) fruit1.++(fruit2) : Set(banana, apples, mangoes, pears, oranges)
查找集合中的最大值和最小值元素
可以使用 **Set.min** 方法查找集合中元素的最小值,使用 **Set.max** 方法查找集合中元素的最大值。以下示例显示了程序。
示例
object Demo { def main(args: Array[String]) { val num = Set(5,6,9,20,30,45) // find min and max of the elements println( "Min element in Set(5,6,9,20,30,45) : " + num.min ) println( "Max element in Set(5,6,9,20,30,45) : " + num.max ) } }
将上述程序保存为 **Demo.scala**。使用以下命令编译并执行此程序。
命令
\>scalac Demo.scala \>scala Demo
输出
Min element in Set(5,6,9,20,30,45) : 5 Max element in Set(5,6,9,20,30,45) : 45
查找集合中的公共值
可以使用 **Set.&** 方法或 **Set.intersect** 方法查找两个集合之间的公共值。尝试以下示例以显示用法。
示例
object Demo { def main(args: Array[String]) { val num1 = Set(5,6,9,20,30,45) val num2 = Set(50,60,9,20,35,55) // find common elements between two sets println( "num1.&(num2) : " + num1.&(num2) ) println( "num1.intersect(num2) : " + num1.intersect(num2) ) } }
将上述程序保存为 **Demo.scala**。使用以下命令编译并执行此程序。
命令
\>scalac Demo.scala \>scala Demo
输出
num1.&(num2) : Set(20, 9) num1.intersect(num2) : Set(20, 9)