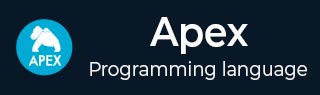
- Apex编程教程
- Apex - 首页
- Apex - 概述
- Apex - 环境
- Apex - 示例
- Apex - 数据类型
- Apex - 变量
- Apex - 字符串
- Apex - 数组
- Apex - 常量
- Apex - 决策
- Apex - 循环
- Apex - 集合
- Apex - 类
- Apex - 方法
- Apex - 对象
- Apex - 接口
- Apex - DML
- Apex - 数据库方法
- Apex - SOSL
- Apex - SOQL
- Apex - 安全性
- Apex - 调用
- Apex - 触发器
- Apex - 触发器设计模式
- Apex - 限制
- Apex - 批量处理
- Apex - 调试
- Apex - 测试
- Apex - 部署
- Apex 有用资源
- Apex - 快速指南
- Apex - 资源
- Apex - 讨论
Apex - 集合
集合是一种可以存储多个记录的变量类型。例如,列表可以存储多个帐户对象的记录。现在让我们详细了解所有集合类型。
列表
列表可以包含任意数量的原始数据、集合、sObject、用户定义和内置 Apex 类型的记录。这是最重要的集合类型之一,并且它还有一些专门为列表使用而定制的系统方法。列表索引始终从 0 开始。这与 Java 中的数组相同。列表应使用关键字“List”声明。
示例
下面是包含原始数据类型(字符串)列表的列表,即城市列表。
List<string> ListOfCities = new List<string>(); System.debug('Value Of ListOfCities'+ListOfCities);
声明列表的初始值是可选的。但是,我们将在此处声明初始值。以下示例显示了相同的内容。
List<string> ListOfStates = new List<string> {'NY', 'LA', 'LV'}; System.debug('Value ListOfStates'+ListOfStates);
帐户列表(sObject)
List<account> AccountToDelete = new List<account> (); //This will be null System.debug('Value AccountToDelete'+AccountToDelete);
我们也可以声明嵌套列表。它最多可以嵌套五层。这称为多维列表。
这是整数集合的列表。
List<List<Set<Integer>>> myNestedList = new List<List<Set<Integer>>>(); System.debug('value myNestedList'+myNestedList);
列表可以包含任意数量的记录,但为了防止性能问题和占用资源,堆大小有限制。
列表方法
列表有一些可用方法,我们在编程时可以使用它们来实现某些功能,例如计算列表的大小、添加元素等。
以下是一些最常用的方法:
- size()
- add()
- get()
- clear()
- set()
以下示例演示了所有这些方法的使用
// Initialize the List List<string> ListOfStatesMethod = new List<string>(); // This statement would give null as output in Debug logs System.debug('Value of List'+ ListOfStatesMethod); // Add element to the list using add method ListOfStatesMethod.add('New York'); ListOfStatesMethod.add('Ohio'); // This statement would give New York and Ohio as output in Debug logs System.debug('Value of List with new States'+ ListOfStatesMethod); // Get the element at the index 0 String StateAtFirstPosition = ListOfStatesMethod.get(0); // This statement would give New York as output in Debug log System.debug('Value of List at First Position'+ StateAtFirstPosition); // set the element at 1 position ListOfStatesMethod.set(0, 'LA'); // This statement would give output in Debug log System.debug('Value of List with element set at First Position' + ListOfStatesMethod[0]); // Remove all the elements in List ListOfStatesMethod.clear(); // This statement would give output in Debug log System.debug('Value of List'+ ListOfStatesMethod);
您也可以使用数组表示法来声明列表,如下所示,但这在 Apex 编程中不是一般的做法:
String [] ListOfStates = new List<string>();
集合
集合是一种包含多个无序唯一记录的集合类型。集合不能包含重复记录。与列表一样,集合也可以嵌套。
示例
我们将定义公司销售的产品集合。
Set<string> ProductSet = new Set<string>{'Phenol', 'Benzene', 'H2SO4'}; System.debug('Value of ProductSet'+ProductSet);
集合方法
集合支持我们在编程时可以利用的方法,如下所示(我们扩展了上面的示例):
// Adds an element to the set // Define set if not defined previously Set<string> ProductSet = new Set<string>{'Phenol', 'Benzene', 'H2SO4'}; ProductSet.add('HCL'); System.debug('Set with New Value '+ProductSet); // Removes an element from set ProductSet.remove('HCL'); System.debug('Set with removed value '+ProductSet); // Check whether set contains the particular element or not and returns true or false ProductSet.contains('HCL'); System.debug('Value of Set with all values '+ProductSet);
映射
它是一个键值对,每个值都包含唯一的键。键和值都可以是任何数据类型。
示例
以下示例表示产品名称与产品代码的映射。
// Initialize the Map Map<string, string> ProductCodeToProductName = new Map<string, string> {'1000'=>'HCL', '1001'=>'H2SO4'}; // This statement would give as output as key value pair in Debug log System.debug('value of ProductCodeToProductName'+ProductCodeToProductName);
映射方法
以下是一些演示可以使用映射的方法的示例:
// Define a new map Map<string, string> ProductCodeToProductName = new Map<string, string>(); // Insert a new key-value pair in the map where '1002' is key and 'Acetone' is value ProductCodeToProductName.put('1002', 'Acetone'); // Insert a new key-value pair in the map where '1003' is key and 'Ketone' is value ProductCodeToProductName.put('1003', 'Ketone'); // Assert that the map contains a specified key and respective value System.assert(ProductCodeToProductName.containsKey('1002')); System.debug('If output is true then Map contains the key and output is:' + ProductCodeToProductName.containsKey('1002')); // Retrieves a value, given a particular key String value = ProductCodeToProductName.get('1002'); System.debug('Value at the Specified key using get function: '+value); // Return a set that contains all of the keys in the map Set SetOfKeys = ProductCodeToProductName.keySet(); System.debug('Value of Set with Keys '+SetOfKeys);
映射值可能是无序的,因此我们不应该依赖于存储值的顺序,并尝试始终使用键访问映射。映射值可以为 null。声明为字符串的映射键区分大小写;例如,ABC 和 abc 将被视为不同的键并被视为唯一。
广告