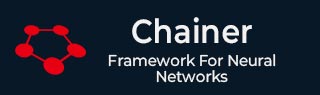
Chainer - 高级功能
Chainer 提供了一些高级功能,增强了其在深度学习中的灵活度、效率和可扩展性。这些功能包括**使用 CuPy 的 GPU 加速**,它利用 NVIDIA GPU 进行更快的计算;**混合精度训练**,它使用 16 位和 32 位浮点数来优化性能和内存使用;以及**分布式训练**,它能够跨多个 GPU 或机器进行扩展,以处理更大的模型和数据集。
此外,Chainer 还提供了强大的**调试和分析工具**,允许实时检查和优化神经网络的性能。这些功能共同促成了 Chainer 能够有效地处理复杂的大规模机器学习任务的能力。
使用 CuPy 的 GPU 加速
**使用 CuPy 的 GPU 加速**是深度学习和数值计算的一个重要方面,它利用 GPU 的计算能力来加速运算。**CuPy** 是一个 GPU 加速库,它提供了一个类似 NumPy 的 API,用于使用 CUDA 在 NVIDIA GPU 上执行运算。它在像 Chainer 这样的深度学习框架中特别有用,可以有效地处理大规模数据和计算。
CuPy 的主要特性
- **类似 NumPy 的 API:**CuPy 提供了一个类似于 NumPy 的接口,通过它可以轻松地从基于 CPU 的计算过渡到基于 GPU 的加速计算,只需进行最少的代码更改。
- **CUDA 后端:**CuPy 利用 CUDA(NVIDIA 的并行计算平台)在 GPU 上执行运算。与基于 CPU 的计算相比,这使得数值运算的性能得到了显著提升。
- **数组操作:**它支持广泛的数组操作,包括元素级操作、归约和线性代数运算,所有这些都由 GPU 加速。
- **与深度学习框架集成:**CuPy 与深度学习框架(如 Chainer)无缝集成,允许使用 GPU 加速高效地训练和评估模型。
示例
在 Chainer 中,我们可以使用 CuPy 数组代替 NumPy 数组,Chainer 会自动利用 GPU 加速进行计算。以下示例演示了如何将 Chainer 与 CuPy 集成:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain, optimizers, Variable import cupy as cp class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.l1 = L.Linear(None, 10) self.l2 = L.Linear(10, 10) self.l3 = L.Linear(10, 1) def forward(self, x): h1 = F.relu(self.l1(x)) h2 = F.relu(self.l2(h1)) y = F.sigmoid(self.l3(h2)) return y # Initialize model and optimizer model = SimpleNN() optimizer = optimizers.Adam() optimizer.setup(model) # Example data (using CuPy arrays) X_train = cp.random.rand(100, 5).astype(cp.float32) y_train = cp.random.randint(0, 2, size=(100, 1)).astype(cp.float32) # Convert to Chainer Variables x_batch = Variable(X_train) y_batch = Variable(y_train) # Forward pass y_pred = model.forward(x_batch) # Compute loss loss = F.sigmoid_cross_entropy(y_pred, y_batch) # Backward pass and update model.cleargrads() loss.backward() optimizer.update()
混合精度训练
**混合精度训练**是一种用于加速深度学习训练并减少内存消耗的技术,它使用不同的数值精度(通常是 float16 和 float32)用于模型和训练过程的不同部分。**16 位浮点数 (FP16)** 用于大多数计算,以节省内存并提高计算速度;**32 位浮点数 (FP32)** 用于精度至关重要的关键操作,例如维护模型的权重和梯度。
混合精度训练的关键组件
- **损失缩放:**为了避免在使用 FP16 进行训练时出现下溢问题,在反向传播之前会对损失进行放大(乘以)。这种缩放有助于将梯度的幅度保持在 FP16 可以处理的范围内。
- **损失动态缩放:**动态损失缩放根据梯度的幅度调整缩放因子,以防止梯度溢出或下溢。
- **FP16 算术运算:**尽可能使用 FP16 执行计算(例如矩阵乘法),然后将结果转换为 FP32 进行累积和更新。
示例
以下示例演示了如何在 chainer 中使用混合精度训练:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain, optimizers, Variable import numpy as np import cupy as cp # Define the model class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.l1 = L.Linear(None, 10) # Input to hidden layer self.l2 = L.Linear(10, 10) # Hidden layer to hidden layer self.l3 = L.Linear(10, 1) # Hidden layer to output layer def __call__(self, x): h1 = F.relu(self.l1(x)) h2 = F.relu(self.l2(h1)) y = F.sigmoid(self.l3(h2)) return y # Mixed Precision Training Function def mixed_precision_training(model, optimizer, X_train, y_train, n_epochs=10, batch_size=10): # Convert inputs to float16 X_train = cp.asarray(X_train, dtype=cp.float16) y_train = cp.asarray(y_train, dtype=cp.float16) scaler = 1.0 # Initial scaling factor for gradients for epoch in range(n_epochs): for i in range(0, len(X_train), batch_size): x_batch = Variable(X_train[i:i+batch_size]) y_batch = Variable(y_train[i:i+batch_size]) # Forward pass y_pred = model(x_batch) # Compute loss (convert y_batch to float32 for loss calculation) loss = F.sigmoid_cross_entropy(y_pred, y_batch.astype(cp.float32)) # Backward pass and weight update model.cleargrads() loss.backward() # Adjust gradients using the scaler for param in model.params(): param.grad *= scaler optimizer.update() # Optionally, adjust scaler based on gradient norms # Here you can implement dynamic loss scaling if needed print(f'Epoch {epoch+1}, Loss: {loss.array}') # Instantiate model and optimizer model = SimpleNN() optimizer = optimizers.Adam() optimizer.setup(model) # Example data (features and labels) X_train = np.random.rand(100, 5).astype(np.float32) # 100 samples, 5 features y_train = np.random.randint(0, 2, size=(100, 1)).astype(np.float32) # 100 binary labels # Perform mixed precision training mixed_precision_training(model, optimizer, X_train, y_train) # Test data X_test = np.random.rand(10, 5).astype(np.float32) # 10 samples, 5 features X_test = cp.asarray(X_test, dtype=cp.float16) # Convert test data to float16 y_test = model(Variable(X_test)) print("Predictions:", y_test.data) # Save the model chainer.serializers.save_npz('simple_nn.model', model) # Load the model chainer.serializers.load_npz('simple_nn.model', model)
分布式训练
Chainer 中的**分布式训练**允许我们将模型训练扩展到多个 GPU 甚至多台机器上。Chainer 提供了工具来促进分布式训练,使我们能够利用并行计算资源来加速训练过程。
分布式训练的关键组件
以下是 Chainer 分布式训练中的关键组件:
- **数据并行:**分布式训练中最常见的方法,其中数据集被分割到多个 GPU 或机器上,每个实例根据其数据子集计算梯度。然后对梯度进行平均,并应用于模型参数。
- **模型并行:**涉及将单个模型分割到多个 GPU 或机器上。每个设备处理模型参数和计算的一部分。这种方法不如数据并行常见,通常用于非常大的模型。
示例
以下示例演示了如何在 Chainer 中使用分布式训练:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain, optimizers, training from chainer.training import extensions from chainer.dataset import DatasetMixin import numpy as np # Define the model class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.l1 = L.Linear(None, 10) self.l2 = L.Linear(10, 10) self.l3 = L.Linear(10, 1) def __call__(self, x): h1 = F.relu(self.l1(x)) h2 = F.relu(self.l2(h1)) y = F.sigmoid(self.l3(h2)) return y # Create a custom dataset class RandomDataset(DatasetMixin): def __init__(self, size=100): self.data = np.random.rand(size, 5).astype(np.float32) self.target = np.random.randint(0, 2, size=(size, 1)).astype(np.float32) def __len__(self): return len(self.data) def get_example(self, i): return self.data[i], self.target[i] # Prepare the dataset and iterators dataset = RandomDataset() train_iter = chainer.iterators.SerialIterator(dataset, batch_size=10) # Set up the model and optimizer model = SimpleNN() optimizer = optimizers.Adam() optimizer.setup(model) # Set up the updater and trainer updater = training.StandardUpdater(train_iter, optimizer, device=0) # Use GPU 0 trainer = training.Trainer(updater, (10, 'epoch'), out='result') # Add extensions trainer.extend(extensions.Evaluator(train_iter, model, device=0)) trainer.extend(extensions.LogReport()) trainer.extend(extensions.PrintReport(['epoch', 'main/loss', 'validation/main/loss'])) trainer.extend(extensions.ProgressBar()) # Run the training trainer.run()
调试和分析工具
Chainer 提供了一系列调试和分析工具,帮助开发人员监控和优化神经网络训练。这些工具有助于识别瓶颈、诊断问题并确保模型训练和评估的正确性。以下是可用关键工具的细分:
- **运行时定义调试** Chainer 的运行时定义架构允许使用标准的 Python 调试工具,例如 print 语句,在正向传递过程中打印中间值以检查变量状态,以及 Python 调试器 (pdb),用于逐步遍历代码以交互式地调试和检查变量。
- **梯度检查** Chainer 提供了使用**chainer.gradient_check**进行梯度检查的内置支持。此工具可确保计算出的梯度与数值估计的梯度相匹配。
- **Chainer 分析器:**Chainer 分析器有助于测量正向和反向传递的执行时间。它识别哪些操作正在减慢训练速度。
- **CuPy 分析器:**对于使用 CuPy 的 GPU 加速模型,Chainer 允许您分析 GPU 操作并优化其执行。
- **内存使用分析:**使用**chainer.reporter**模块跟踪训练期间的内存消耗,以确保高效的内存管理,尤其是在大型模型中。
- **处理数值不稳定性:**诸如**chainer.utils.isfinite()**之类的工具检测张量中的 NaN 或 Inf 值,梯度裁剪可以防止梯度爆炸。
这些功能使在 Chainer 中调试和优化神经网络变得容易,同时确保模型训练期间的性能和稳定性。
示例
以下示例演示了如何使用 Chainer 的调试和分析工具监控简单神经网络的训练:
import chainer import chainer.functions as F import chainer.links as L from chainer import Variable, Chain, optimizers, training, report import numpy as np from chainer import reporter, profiler # Define a simple neural network model class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.l1 = L.Linear(None, 10) # Input layer to hidden layer self.l2 = L.Linear(10, 1) # Hidden layer to output layer def forward(self, x): h1 = F.relu(self.l1(x)) # ReLU activation y = self.l2(h1) return y # Create a simple dataset X_train = np.random.rand(100, 5).astype(np.float32) # 100 samples, 5 features y_train = np.random.rand(100, 1).astype(np.float32) # 100 target values # Instantiate the model and optimizer model = SimpleNN() optimizer = optimizers.Adam() optimizer.setup(model) # Enable the profiler with profiler.profile() as prof: # Start profiling for epoch in range(10): # Training for 10 epochs for i in range(0, len(X_train), 10): # Batch size of 10 x_batch = Variable(X_train[i:i+10]) y_batch = Variable(y_train[i:i+10]) # Forward pass y_pred = model.forward(x_batch) # Debugging using print statements print(f'Epoch {epoch+1}, Batch {i//10+1}: Predicted {y_pred.data}, Actual {y_batch.data}') # Compute loss loss = F.mean_squared_error(y_pred, y_batch) # Clear gradients, backward pass, and update model.cleargrads() loss.backward() optimizer.update() # Report memory usage (for large models) reporter.report({'loss': loss}) # Output profiling result prof.print() # Print profiling information # Check for NaN or Inf in weights for param in model.params(): assert chainer.utils.isfinite(param.array), "NaN or Inf found in parameters!" print("Training complete!")