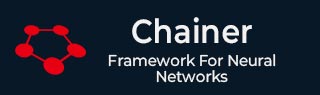
Chainer - 核心组件
Chainer是一个用途广泛的深度学习框架,旨在简化神经网络的开发和训练。Chainer的核心组件为构建复杂模型和执行高效计算提供了坚实的基础。
在Chainer中,核心组件Chain类用于管理网络层和参数,例如定义和应用模型操作的Links和Functions,以及处理数据和梯度的Variable类。
此外,Chainer集成了强大的优化器(Optimizers)用于更新模型参数,实用程序用于管理数据集(Dataset)和DataLoader,以及支持灵活模型架构的动态计算图(Computational Graph)。所有这些组件共同实现了模型创建、训练和优化的简化流程,使Chainer成为深度学习任务的全面工具。
以下是Chainer框架的不同核心组件:
变量(Variables)
在Chainer中,Variable类是表示数据及其在神经网络训练过程中相关梯度的基本构建块。Variable不仅封装了数据(例如输入、输出或中间计算),还封装了自动微分所需的信息,这对于反向传播至关重要。
Variable的关键特性
以下是Chainer框架中变量的关键特性:
- 数据存储:Variable以多维数组的形式保存数据,该数组通常是NumPy或CuPy数组,具体取决于计算是在CPU还是GPU上执行。存储在Variable中的数据可以是输入数据、输出预测或在网络前向传递过程中计算的任何中间值。
- 梯度存储:在反向传播过程中,Chainer计算损失函数相对于每个Variable的梯度。这些梯度存储在Variable本身中。Variable的grad属性包含梯度数据,该数据用于在训练期间更新模型参数。
- 自动微分:当对Variable对象应用操作时,Chainer会自动构建计算图。该图跟踪操作序列以及变量之间的依赖关系,从而能够在反向传递过程中高效地计算梯度。可以通过调用Variable的backward方法来触发整个网络的梯度计算。
- 设备灵活性:Variable支持使用NumPy的CPU和使用CuPy的GPU数组,从而可以轻松地在设备之间移动计算。Variable上的操作会自动适应数据所在的设备。
示例
以下示例演示了如何使用Chainer的Variable类执行基本操作并通过反向传播计算梯度:
import chainer import numpy as np # Create a Variable with data x = chainer.Variable(np.array([1.0, 2.0, 3.0], dtype=np.float32)) # Perform operations on Variable y = x ** 2 + 2 * x + 1 # Print the result print("Result:", y.data) # Output: [4. 9. 16.] # Assume y is a loss and perform backward propagation y.grad = np.ones_like(y.data) # Set gradient of y to 1 for backward pass y.backward() # Compute gradients # Print the gradient of x print("Gradient of x:", x.grad) # Output: [4. 6. 8.]
以下是Chainer变量类的输出:
Result: [ 4. 9. 16.] Gradient of x: [4. 6. 8.]
函数(Functions)
在Chainer中,函数(Functions)是应用于神经网络内数据的操作。这些函数是执行数学运算、激活函数、损失计算以及对数据进行其他转换的基本构建块,数据在网络中流动时会执行这些转换。
Chainer在chainer.functions模块中提供广泛的预定义函数,使用户能够轻松构建和自定义神经网络。
Chainer中的关键函数
激活函数:神经网络中的这些函数为模型引入了非线性,使其能够学习数据中的复杂模式。它们应用于每一层的输出,以确定网络的最终输出。以下是Chainer中的激活函数:
ReLU(修正线性单元):如果输入为正数,则ReLU直接输出输入,否则输出零。它广泛用于神经网络,因为它有助于减轻梯度消失问题,并且计算效率高,使其成为训练深度模型的有效方法。ReLU的公式为:
$$ReLU(x) = max(0, x)$$
chainer.functions模块中ReLU的函数为F.relu(x)。
sigmoid:此函数将输入映射到0到1之间的值,使其成为二元分类任务的理想选择。它提供了一个平滑的梯度,这有助于基于梯度的优化,但在深层网络中可能会遭受梯度消失问题。sigmoid的公式为:
$$Sigmoid(x)=\frac{1}{1+e^{-x}}$$
chainer.functions模块中Sigmoid的函数为F.sigmoid(x)
Tanh(双曲正切):Chainer中此函数用作神经网络中的激活函数。它将输入转换为-1到1之间的值,从而产生以零为中心的输出。此特性在训练中可能是有益的,因为它有助于解决与非中心数据相关的问题,从而潜在地提高模型的收敛速度。Tanh的公式为:
$$Tanh(x) = \frac{e^x - e^{-x}}{e^x + e^{-x}}$$
我们在chainer.functions模块中有一个函数F.tanh(x)用于计算Chainer中的Tanh。
Leaky ReLU:这也被称为神经网络中的泄漏修正线性单元函数,是标准ReLU激活函数的一个变体。与ReLU不同的是,ReLU对负输入输出零,而Leaky ReLU允许对负输入进行小的非零梯度。
此调整有助于防止“死亡ReLU”问题,其中神经元变得不活跃并停止学习,通过确保所有神经元继续参与模型的学习过程来实现。Leaky ReLU的公式为:
$$Leaky Relu(x) = max(\alpha x, x)$$
其中,$\alpha$是一个小常数。chainer.functions模块具有函数F.leaky_relu(x)用于在Chainer中计算Leaky ReLU。
Softmax:这是一个激活函数,通常用于神经网络的输出层,特别是对于多类分类任务。它将原始预测分数(logits)向量转换为概率分布,其中每个概率与其对应的输入值的指数成比例。
输出向量中的概率总和为1,这使得Softmax成为表示分类问题中每个类的可能性理想的选择。Softmax的公式为:
$$Softmax(x_{i})=\frac{e^{x_{i}}}{\sum_{j} e^{x_{j}}}$$
chainer.functions模块具有函数F.softmax(x)用于在Chainer中计算Softmax。
示例
这是一个示例,它演示了如何在简单的网络中使用Chainer中的各种激活函数:
import chainer import chainer.links as L import chainer.functions as F import numpy as np # Define a simple neural network using Chainer's Chain class class SimpleNN(chainer.Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): # Define layers: two linear layers self.l1 = L.Linear(4, 3) # Input layer with 4 features, hidden layer with 3 units self.l2 = L.Linear(3, 2) # Hidden layer with 3 units, output layer with 2 units def __call__(self, x): # Forward pass using different activation functions # Apply ReLU activation after the first layer h = F.relu(self.l1(x)) # Apply Sigmoid activation after the second layer y = F.sigmoid(self.l2(h)) return y # Create a sample input data with 4 features x = np.array([[0.5, -1.2, 3.3, 0.7]], dtype=np.float32) # Convert input to Chainer's Variable x_var = chainer.Variable(x) # Instantiate the neural network model = SimpleNN() # Perform a forward pass output = model(x_var) # Print the output print("Network output after applying ReLU and Sigmoid activations:", output.data)
以下是简单的网络中使用的激活函数的输出:
Network output after applying ReLU and Sigmoid activations: [[0.20396319 0.7766712 ]]
Chain和ChainList
在Chainer中,Chain和ChainList是基本类,它们有助于组织和管理神经网络中的层和参数。Chain和ChainList都是从chainer.Link派生的,chainer.Link是负责定义模型参数的基类。但是,它们具有不同的用途,并且用于不同的场景。让我们详细了解Chain和ChainList:
Chain
Chain类旨在将神经网络或网络中的模块表示为链接(层)的集合。使用Chain时,我们可以通过将每一层显式地指定为实例变量来定义网络结构。这种方法对于具有固定架构的网络非常有用。
当我们拥有一个定义明确的固定网络架构,并且想要直接访问和组织模型的每一层或组件时,可以使用Chain。
以下是Chain类的关键特性:
- 命名组件:添加到Chain的层或链接可以通过名称访问,这使得引用网络的特定部分变得简单直接。
- 静态架构:Chain的结构通常在初始化时定义,在训练或推理期间不会动态更改。
示例
以下示例演示了在Chainer框架中使用Chain类:
import chainer import chainer.links as L import chainer.functions as F # Define a simple neural network using Chain class SimpleChain(chainer.Chain): def __init__(self): super(SimpleChain, self).__init__() with self.init_scope(): self.l1 = L.Linear(4, 3) # Linear layer with 4 inputs and 3 outputs self.l2 = L.Linear(3, 2) # Linear layer with 3 inputs and 2 outputs def forward(self, x): h = F.relu(self.l1(x)) # Apply ReLU after the first layer y = self.l2(h) # No activation after the second layer return y # Instantiate the model model = SimpleChain() print(model)
以下是上述示例的输出:
SimpleChain( (l1): Linear(in_size=4, out_size=3, nobias=False), (l2): Linear(in_size=3, out_size=2, nobias=False), )
ChainList
ChainList类类似于Chain,但我们不是将每一层定义为实例变量,而是将其存储在类似列表的结构中。当层或组件的数量可能发生变化或架构是动态的时,ChainList非常有用。
当我们的模型具有可变数量的层,或者网络结构可以动态更改时,可以使用ChainList。它也适用于诸如循环网络之类的架构,在这些架构中,相同类型的层被多次使用。
以下是ChainList的关键特性:
- 无序组件:添加到ChainList的层或链接通过其索引而不是名称来访问。
- 灵活的架构:它更适合于网络结构可能会发生变化或在循环或列表中处理层的情况。
示例
以下示例演示了如何在Chainer框架中使用ChainList类:
import chainer import chainer.links as L import chainer.functions as F # Define a neural network using ChainList class SimpleChainList(chainer.ChainList): def __init__(self): super(SimpleChainList, self).__init__( L.Linear(4, 3), # Linear layer with 4 inputs and 3 outputs L.Linear(3, 2) # Linear layer with 3 inputs and 2 outputs ) def forward(self, x): h = F.relu(self[0](x)) # Apply ReLU after the first layer y = self[1](h) # No activation after the second layer return y # Instantiate the model model = SimpleChainList() print(model)
以下是使用Chainer框架中ChainList类的输出:
SimpleChainList( (0): Linear(in_size=4, out_size=3, nobias=False), (1): Linear(in_size=3, out_size=2, nobias=False), )
优化器(Optimizers)
在Chainer中,优化器(Optimizers)在训练神经网络中起着至关重要的作用,它通过调整模型的参数(例如权重和偏差)来最小化损失函数。
在训练期间,在通过反向传播计算出损失函数相对于参数的梯度后,优化器会使用这些梯度来更新参数,从而逐渐减少损失。
Chainer提供了各种内置优化器,每个优化器都采用不同的参数更新策略,以适应不同类型的模型和任务。以下是Chainer中的关键优化器:
SGD(随机梯度下降)
最基本的优化器是SGD,它沿其负梯度的方向更新每个参数,并按学习率缩放。它很简单,但收敛速度可能很慢。
这些通常可以用于更简单或更小的模型,或者作为与更复杂优化器进行比较的基线。
Chainer中计算SGD的函数为chainer.optimizers.SGD示例
这是一个使用Chainer中的随机梯度下降(SGD)训练基本神经网络的简单示例。我们将使用一个小数据集,定义一个神经网络模型,然后应用SGD优化器在训练期间更新模型的参数:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain import numpy as np from chainer import Variable from chainer import optimizers class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.fc1 = L.Linear(None, 100) # Fully connected layer with 100 units self.fc2 = L.Linear(100, 10) # Output layer with 10 units (e.g., for 10 classes) def forward(self, x): h = F.relu(self.fc1(x)) # Apply ReLU activation function return self.fc2(h) # Output layer # Dummy data: 5 samples, each with 50 features x_data = np.random.rand(5, 50).astype(np.float32) # Dummy labels: 5 samples, each with 10 classes (one-hot encoded) y_data = np.random.randint(0, 10, 5).astype(np.int32) # Convert to Chainer variables x = Variable(x_data) y = Variable(y_data) # Initialize the model model = SimpleNN() # Set up SGD optimizer with a learning rate of 0.01 optimizer = optimizers.SGD(lr=0.01) optimizer.setup(model) def loss_func(predictions, targets): return F.softmax_cross_entropy(predictions, targets) # Training loop for epoch in range(10): # Number of epochs # Zero the gradients model.cleargrads() # Forward pass predictions = model(x) # Calculate loss loss = loss_func(predictions, y) # Backward pass loss.backward() # Update parameters optimizer.update() # Print loss print(f'Epoch {epoch + 1}, Loss: {loss.data}')
以下是SGD优化器的输出:
Epoch 1, Loss: 2.3100974559783936 Epoch 2, Loss: 2.233552932739258 Epoch 3, Loss: 2.1598660945892334 Epoch 4, Loss: 2.0888497829437256 Epoch 5, Loss: 2.020642042160034 Epoch 6, Loss: 1.9552147388458252 Epoch 7, Loss: 1.8926388025283813 Epoch 8, Loss: 1.8325523138046265 Epoch 9, Loss: 1.7749309539794922 Epoch 10, Loss: 1.7194255590438843
Momentum SGD
Momentum SGD是SGD的扩展,它包括动量,这有助于加速梯度向量向正确的方向移动,从而导致更快的收敛。它累积一个朝梯度方向的速率向量。
这适用于普通SGD难以收敛的模型。我们有一个名为chainer.optimizers.MomentumSGD的函数来执行Momentum SGD优化。
动量项:将先前梯度更新的一部分添加到当前更新中。这有助于加速梯度向量向正确的方向移动并抑制振荡。
公式:带有动量的参数θ的更新规则为:
$$v_{t} = \beta v_{t-1} + (1 - \beta) \nabla L(\theta)$$ $$\theta = \theta-\alpha v_{t}$$
其中:
- $v_{t}$是速度(或累积梯度)
- $\beta$是动量系数(通常约为0.9)
- $\alpha$是学习率
- $\nabla L(\theta)$是损失函数相对于参数的梯度。
示例
这是一个关于如何在Chainer中使用Momentum SGD优化器和简单神经网络的基本示例:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain from chainer import optimizers import numpy as np from chainer import Variable class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.fc1 = L.Linear(None, 100) # Fully connected layer with 100 units self.fc2 = L.Linear(100, 10) # Output layer with 10 units (e.g., for 10 classes) def forward(self, x): h = F.relu(self.fc1(x)) # Apply ReLU activation function return self.fc2(h) # Output layer # Dummy data: 5 samples, each with 50 features x_data = np.random.rand(5, 50).astype(np.float32) # Dummy labels: 5 samples, each with 10 classes (one-hot encoded) y_data = np.random.randint(0, 10, 5).astype(np.int32) # Convert to Chainer variables x = Variable(x_data) y = Variable(y_data) # Initialize the model model = SimpleNN() # Set up Momentum SGD optimizer with a learning rate of 0.01 and momentum of 0.9 optimizer = optimizers.MomentumSGD(lr=0.01, momentum=0.9) optimizer.setup(model) def loss_func(predictions, targets): return F.softmax_cross_entropy(predictions, targets) # Training loop for epoch in range(10): # Number of epochs # Zero the gradients model.cleargrads() # Forward pass predictions = model(x) # Calculate loss loss = loss_func(predictions, y) # Backward pass loss.backward() # Update parameters optimizer.update() # Print loss print(f'Epoch {epoch + 1}, Loss: {loss.data}')
以下是Momentum SGD优化器的输出:
Epoch 1, Loss: 2.4459869861602783 Epoch 2, Loss: 2.4109833240509033 Epoch 3, Loss: 2.346194267272949 Epoch 4, Loss: 2.25825572013855 Epoch 5, Loss: 2.153470754623413 Epoch 6, Loss: 2.0379838943481445 Epoch 7, Loss: 1.9174035787582397 Epoch 8, Loss: 1.7961997985839844 Epoch 9, Loss: 1.677260398864746 Epoch 10, Loss: 1.5634090900421143
Adam
Adam 优化器结合了 SGD 的另外两个扩展的优点,即AdaGrad(擅长处理稀疏梯度)和RMSProp(擅长处理非平稳环境)。Adam 保持梯度及其平方的移动平均值,并根据这些平均值更新参数。
由于其在各种任务和模型中的鲁棒性和效率,它通常用作默认优化器。在 Chainer 中,我们使用函数chainer.optimizers.Adam 执行 Adam 优化。
以下是 Adam 优化器的关键特性:
- 自适应学习率:Adam 为每个参数动态调整学习率,使其在各种任务中有效。
- 梯度的矩:它计算梯度的一阶矩(均值)和二阶矩(无偏方差)以改进优化。
- 偏差校正:Adam 使用偏差校正来解决初始化过程中引入的偏差,尤其是在训练初期。
- 公式:Adam 优化的公式如下:
$$m_t = \beta_1 m_{t-1} + (1 - \beta_1) \nabla L(\theta)$$ $$v_t = \beta_2 v_{t-1} + (1 - \beta_2) (\nabla L(\theta))^2$$ $$\hat{m}_t = \frac{m_t}{1 - \beta_1^t}$$ $$\hat{v}_t = \frac{v_t}{1 - \beta_2^t}$$ $$\theta = \theta - \frac{\alpha\hat{m}_t}{\sqrt{\hat{v}_t} + \epsilon}$$
其中,$\alpha$ 是学习率,$\beta_1$ 和 $\beta_2$ 分别是梯度及其平方的移动平均值的衰减率,通常分别为 0.9 和 0.999,${m_t}$ 和 ${v_t}$ 分别是一阶矩和二阶矩估计,$\epsilon$ 是为了数值稳定性而添加的小常数。
示例
以下示例展示了如何在 Chainer 中使用 Adam 优化器和神经网络:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain from chainer import optimizers import numpy as np from chainer import Variable class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.fc1 = L.Linear(None, 100) # Fully connected layer with 100 units self.fc2 = L.Linear(100, 10) # Output layer with 10 units (e.g., for 10 classes) def forward(self, x): h = F.relu(self.fc1(x)) # Apply ReLU activation function return self.fc2(h) # Output layer # Dummy data: 5 samples, each with 50 features x_data = np.random.rand(5, 50).astype(np.float32) # Dummy labels: 5 samples, each with 10 classes (one-hot encoded) y_data = np.random.randint(0, 10, 5).astype(np.int32) # Convert to Chainer variables x = Variable(x_data) y = Variable(y_data) # Initialize the model model = SimpleNN() # Set up Adam optimizer with default parameters optimizer = optimizers.Adam() optimizer.setup(model) def loss_func(predictions, targets): return F.softmax_cross_entropy(predictions, targets) # Training loop for epoch in range(10): # Number of epochs # Zero the gradients model.cleargrads() # Forward pass predictions = model(x) # Calculate loss loss = loss_func(predictions, y) # Backward pass loss.backward() # Update parameters optimizer.update() # Print loss print(f'Epoch {epoch + 1}, Loss: {loss.data}')
以下是将Adam 优化器应用于神经网络的输出:
Epoch 1, Loss: 2.4677982330322266 Epoch 2, Loss: 2.365001678466797 Epoch 3, Loss: 2.2655398845672607 Epoch 4, Loss: 2.1715924739837646 Epoch 5, Loss: 2.082294464111328 Epoch 6, Loss: 1.9973262548446655 Epoch 7, Loss: 1.9164447784423828 Epoch 8, Loss: 1.8396313190460205 Epoch 9, Loss: 1.7676666975021362 Epoch 10, Loss: 1.7006778717041016
AdaGrad
AdaGrad 也称为自适应梯度算法,这是一种优化算法,它根据训练期间累积的梯度历史记录为每个参数调整学习率。它对于稀疏数据和特征频率或重要性不同的场景特别有效。
这适用于具有稀疏数据的问题,以及处理某些参数需要比其他参数更多调整的模型。函数chainer.optimizers.AdaGrad 用于在 Chainer 中执行 AdaGrad 优化。
以下是 AdaGrad 优化器的关键特性:
- 自适应学习率:AdaGrad 根据梯度平方累积和分别为每个参数调整学习率。这导致对不频繁的参数进行较大的更新,对频繁的参数进行较小的更新。
- 无需调整学习率:AdaGrad 自动缩放学习率,通常无需手动调整。
公式:AdaGrad 的公式如下:
$$g_t = \nabla L(\theta)$$ $$G_t = G_{t-1} +{g_t}^2$$ $$\theta = \theta - \frac{\alpha}{\sqrt{G_t} + \epsilon} g_t$$其中:
- $g_t$ 是 t 时刻的梯度。
- $G_t$ 是直到 t 时刻梯度平方的累积和。
- $\alpha$ 是全局学习率。
- $\epsilon$ 是一个小的常数,用于防止除以零。
示例
这是一个如何在 Chainer 中使用 AdaGrad 优化器和简单神经网络的示例:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain from chainer import optimizers import numpy as np from chainer import Variable class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.fc1 = L.Linear(None, 100) # Fully connected layer with 100 units self.fc2 = L.Linear(100, 10) # Output layer with 10 units (e.g., for 10 classes) def forward(self, x): h = F.relu(self.fc1(x)) # Apply ReLU activation function return self.fc2(h) # Output layer # Dummy data: 5 samples, each with 50 features x_data = np.random.rand(5, 50).astype(np.float32) # Dummy labels: 5 samples, each with 10 classes (one-hot encoded) y_data = np.random.randint(0, 10, 5).astype(np.int32) # Convert to Chainer variables x = Variable(x_data) y = Variable(y_data) # Initialize the model model = SimpleNN() # Set up AdaGrad optimizer with a learning rate of 0.01 optimizer = optimizers.AdaGrad(lr=0.01) optimizer.setup(model) def loss_func(predictions, targets): return F.softmax_cross_entropy(predictions, targets) # Training loop for epoch in range(10): # Number of epochs # Zero the gradients model.cleargrads() # Forward pass predictions = model(x) # Calculate loss loss = loss_func(predictions, y) # Backward pass loss.backward() # Update parameters optimizer.update() # Print loss print(f'Epoch {epoch + 1}, Loss: {loss.data}')
以下是将AdaGrad 优化器应用于神经网络的输出:
Epoch 1, Loss: 2.2596702575683594 Epoch 2, Loss: 1.7732301950454712 Epoch 3, Loss: 1.4647505283355713 Epoch 4, Loss: 1.2398217916488647 Epoch 5, Loss: 1.0716438293457031 Epoch 6, Loss: 0.9412426352500916 Epoch 7, Loss: 0.8350374102592468 Epoch 8, Loss: 0.7446572780609131 Epoch 9, Loss: 0.6654194593429565 Epoch 10, Loss: 0.59764164686203
RMSProp
RMSProp 优化器通过引入衰减因子来改进AdaGrad,通过防止学习率下降过多,从而防止学习率过小。它在循环神经网络或需要快速适应不同梯度尺度的模型中特别有效。
在 Chainer 中,我们使用函数chainer.optimizers.RMSprop 执行 RMSProp 优化。
以下是 RMSProp 优化器的关键特性:
- 衰减因子:RMSProp 通过防止学习率变得太小并允许更稳定的收敛,向梯度平方累积和引入了衰减因子。
- 自适应学习率:与 AdaGrad 一样,RMSProp 优化器根据梯度历史记录分别为每个参数调整学习率,但它通过限制过去梯度平方的累积来避免学习率减小的问题。
公式:RMSProp 优化器的公式如下:
$$g_t = \nabla L(\theta)$$ $$E[g^2]_t = \gamma E[g^2]_{t-1} + (1 - \gamma){g_t}^2$$ $$\theta = \theta - \frac{\alpha}{\sqrt{E[g^2]_t} + \epsilon} g_t$$
其中:
- $g_t$ 是 t 时刻的梯度。
- $E[g^2]_t$ 是梯度平方的移动平均值。
- $\gamma$ 是衰减因子,通常约为 0.9。
- $\alpha$ 是全局学习率。
- $\epsilon$ 是一个小的常数,用于防止除以零。
示例
以下示例展示了如何在 Chainer 中使用RMSProp 优化器和简单神经网络:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain import numpy as np from chainer import Variable from chainer import optimizers class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.fc1 = L.Linear(None, 100) # Fully connected layer with 100 units self.fc2 = L.Linear(100, 10) # Output layer with 10 units (e.g., for 10 classes) def forward(self, x): h = F.relu(self.fc1(x)) # Apply ReLU activation function return self.fc2(h) # Output layer # Dummy data: 5 samples, each with 50 features x_data = np.random.rand(5, 50).astype(np.float32) # Dummy labels: 5 samples, each with 10 classes (one-hot encoded) y_data = np.random.randint(0, 10, 5).astype(np.int32) # Convert to Chainer variables x = Variable(x_data) y = Variable(y_data) # Initialize the model model = SimpleNN() # Set up RMSProp optimizer with a learning rate of 0.01 and decay factor of 0.9 optimizer = optimizers.RMSprop(lr=0.01, alpha=0.9) optimizer.setup(model) def loss_func(predictions, targets): return F.softmax_cross_entropy(predictions, targets) # Training loop for epoch in range(10): # Number of epochs # Zero the gradients model.cleargrads() # Forward pass predictions = model(x) # Calculate loss loss = loss_func(predictions, y) # Backward pass loss.backward() # Update parameters optimizer.update() # Print loss print(f'Epoch {epoch + 1}, Loss: {loss.data}')
以下是使用 RMSProp 优化的上述示例的输出:
Epoch 1, Loss: 2.3203792572021484 Epoch 2, Loss: 1.1593462228775024 Epoch 3, Loss: 1.2626817226409912 Epoch 4, Loss: 0.6015896201133728 Epoch 5, Loss: 0.3906801640987396 Epoch 6, Loss: 0.28964582085609436 Epoch 7, Loss: 0.21569299697875977 Epoch 8, Loss: 0.15832018852233887 Epoch 9, Loss: 0.12146510928869247 Epoch 10, Loss: 0.09462013095617294
Chainer 中的数据集和迭代器
在 Chainer 中,高效地处理数据对于训练神经网络至关重要。为了促进这一点,Chainer 框架提供了两个基本组件,即数据集和迭代器。这些组件通过确保数据以结构化和高效的方式馈送到模型来帮助管理数据。
数据集
Chainer 中的数据集是可以馈送到神经网络进行训练、验证或测试的数据样本的集合。Chainer 提供了一个 Dataset 类,可以扩展它来创建自定义数据集,以及用于常见任务的几个内置数据集类。
Chainer 中的数据集类型
Chainer 提供了几种类型的数据集来处理各种数据格式和结构。这些数据集可以大致分为内置数据集、自定义数据集和数据集转换。
内置数据集
Chainer 附带了一些常用的数据集,通常用于基准测试和实验。这些数据集随时可用,可以使用内置函数轻松加载。
以下是获取 Chainer 中所有可用数据集列表的代码:
import chainer.datasets as datasets # Get all attributes in the datasets module all_datasets = [attr for attr in dir(datasets) if attr.startswith('get_')] # Print the available datasets print("Built-in datasets available in Chainer:") for dataset in all_datasets: print(f"- {dataset}")
以下是显示 Chainer 框架中所有内置数据集的输出:
Built-in datasets available in Chainer: - get_cifar10 - get_cifar100 - get_cross_validation_datasets - get_cross_validation_datasets_random - get_fashion_mnist - get_fashion_mnist_labels - get_kuzushiji_mnist - get_kuzushiji_mnist_labels - get_mnist - get_ptb_words - get_ptb_words_vocabulary - get_svhn
自定义数据集
当使用自定义数据时,我们可以通过子类化chainer.dataset.DatasetMixin来创建我们自己的数据集。这允许我们定义如何加载和返回数据。
这是一个使用chainer.dataset.DatasetMixin创建自定义数据集并打印其中第一行的示例:
import chainer import numpy as np class MyDataset(chainer.dataset.DatasetMixin): def __init__(self, data, labels): self.data = data self.labels = labels def __len__(self): return len(self.data) def get_example(self, i): return self.data[i], self.labels[i] # Creating a custom dataset data = np.random.rand(100, 3) labels = np.random.randint(0, 2, 100) dataset = MyDataset(data, labels) print(dataset[0])
以下是自定义数据集第一行的输出:
(array([0.82744124, 0.33828446, 0.06409377]), 0)
预处理数据集
Chainer 提供了将转换应用于数据集的工具,例如缩放、归一化或数据增强。可以使用 TransformDataset 动态应用这些转换。
这是一个在 Chainer 中使用预处理数据集的示例:
from chainer.datasets import TransformDataset def transform(data): x, t = data x = x / 255.0 # Normalize input data return x, t # Apply transformation to dataset transformed_dataset = TransformDataset(dataset, transform) print(transformed_dataset[0])
以下是使用 TransformDataset() 函数获得的预处理数据集的第一行:
(array([0.00324487, 0.00132661, 0.00025135]), 0)
连接数据集
ConcatDataset 用于将多个数据集连接成单个数据集。当我们的数据分布在不同的来源时,这非常有用。这是一个在 Chainer 框架中使用连接数据集的示例,它打印出连接数据集中每个样本的数据和标签。组合数据集包含数据集 1 和数据集 2 的所有样本:
import numpy as np from chainer.datasets import ConcatenatedDataset from chainer.dataset import DatasetMixin # Define a custom dataset class class MyDataset(DatasetMixin): def __init__(self, data, labels): self.data = data self.labels = labels def __len__(self): return len(self.data) def get_example(self, i): return self.data[i], self.labels[i] # Sample data arrays data1 = np.random.rand(5, 3) # 5 samples, 3 features each labels1 = np.random.randint(0, 2, 5) # Binary labels for data1 data2 = np.random.rand(5, 3) # Another 5 samples, 3 features each labels2 = np.random.randint(0, 2, 5) # Binary labels for data2 # Create MyDataset instances dataset1 = MyDataset(data1, labels1) dataset2 = MyDataset(data2, labels2) # Concatenate the datasets combined_dataset = ConcatenatedDataset(dataset1, dataset2) # Iterate over the combined dataset and print each example for i in range(len(combined_dataset)): data, label = combined_dataset[i] print(f"Sample {i+1}: Data = {data}, Label = {label}")
以下是 Chainer 中连接数据集的输出:
Sample 1: Data = [0.6153635 0.19185915 0.26029754], Label = 1 Sample 2: Data = [0.69201927 0.70393578 0.85382294], Label = 1 Sample 3: Data = [0.46647242 0.37787839 0.37249345], Label = 0 Sample 4: Data = [0.2975833 0.90399536 0.15978975], Label = 1 Sample 5: Data = [0.29939455 0.21290926 0.97327959], Label = 1 Sample 6: Data = [0.68297438 0.64874375 0.09129224], Label = 1 Sample 7: Data = [0.52026288 0.24197601 0.5239313 ], Label = 0 Sample 8: Data = [0.63250008 0.85023346 0.94985447], Label = 1 Sample 9: Data = [0.75183151 0.01774763 0.66343944], Label = 0 Sample 10: Data = [0.60212864 0.48215319 0.02736618], Label = 0
元组和字典数据集
Chainer 提供了名为TupleDataset 和DictDataset 的特殊数据集类,允许我们方便地管理多个数据源。当我们有多种类型的数据(例如特征和标签)或我们想要一起处理的多个特征集时,这些类非常有用。
- 元组数据集:用于将多个数据集或数据数组组合成单个数据集,其中每个元素都是来自原始数据集的对应元素的元组。
这是一个展示如何在神经网络中使用元组数据集的示例:
import numpy as np from chainer.datasets import TupleDataset # Create two datasets (or data arrays) data1 = np.random.rand(100, 3) # 100 samples, 3 features each data2 = np.random.rand(100, 5) # 100 samples, 5 features each # Create a TupleDataset combining both data arrays tuple_dataset = TupleDataset(data1, data2) # Accessing data from the TupleDataset for i in range(5): print(f"Sample {i+1}: Data1 = {tuple_dataset[i][0]}, Data2 = {tuple_dataset[i][1]}")
以下是元组数据集的输出:
Sample 1: Data1 = [0.32992823 0.57362303 0.95586597], Data2 = [0.41455 0.52850591 0.55602243 0.36316931 0.93588697] Sample 2: Data1 = [0.37731994 0.00452533 0.67853069], Data2 = [0.71637691 0.04191565 0.54027323 0.68738626 0.01887967] Sample 3: Data1 = [0.85808665 0.15863516 0.51649116], Data2 = [0.9596284 0.12417238 0.22897152 0.63822924 0.99434029] Sample 4: Data1 = [0.2477932 0.27937585 0.59660463], Data2 = [0.92666318 0.93611279 0.96622103 0.41834484 0.72602107] Sample 5: Data1 = [0.71989544 0.46155552 0.31835487], Data2 = [0.27475741 0.33759694 0.22539997 0.40985004 0.00469414]
这是一个展示如何在 Chainer 中使用字典数据集的示例:
import numpy as np from chainer.datasets import DictDataset # Create two datasets (or data arrays) data1 = np.random.rand(100, 3) # 100 samples, 3 features each labels = np.random.randint(0, 2, 100) # Binary labels for each sample # Create a DictDataset dict_dataset = DictDataset(data=data1, label=labels) # Accessing data from the DictDataset for i in range(5): print(f"Sample {i+1}: Data = {dict_dataset[i]['data']}, Label = {dict_dataset[i]['label']}")
以下是元组数据集的输出:
Sample 1: Data = [0.09362018 0.33198328 0.11421714], Label = 1 Sample 2: Data = [0.53655817 0.9115115 0.0192754 ], Label = 0 Sample 3: Data = [0.48746879 0.18567869 0.88030764], Label = 0 Sample 4: Data = [0.10720832 0.79523399 0.56056922], Label = 0 Sample 5: Data = [0.76360577 0.69915416 0.64604595], Label = 1
迭代器
在 Chainer 中,迭代器对于管理机器学习模型训练期间的数据至关重要。它们将大型数据集分解成较小的块,称为小批量,这些块可以增量处理。这种方法提高了内存效率,并通过允许模型更频繁地更新其参数来加快训练过程。
Chainer 中的迭代器类型
Chainer 提供了各种类型的迭代器来处理机器学习模型的训练和评估期间的数据集。这些迭代器旨在处理不同的场景和需求,例如处理大型数据集、并行数据加载或确保数据混洗以获得更好的泛化能力。
SerialIterator
这是 Chainer 中最常见的迭代器。它以串行(顺序)方式迭代数据集,提供小批量数据。当到达数据集的末尾时,迭代器可以停止或从开头重新开始,具体取决于重复选项。这在数据顺序得到保留的标准训练中非常理想。
这是一个展示如何在 Chainer 中使用SerialIterator 的示例:
import chainer import numpy as np from chainer import datasets, iterators # Create a simple dataset (e.g., dummy data) x_data = np.random.rand(100, 2).astype(np.float32) # 100 samples, 2 features each y_data = np.random.randint(0, 2, size=(100,)).astype(np.int32) # 100 binary labels # Combine the features and labels into a Chainer dataset dataset = datasets.TupleDataset(x_data, y_data) # Initialize the SerialIterator iterator = iterators.SerialIterator(dataset, batch_size=10, repeat=True, shuffle=True) # Example of iterating over the dataset for epoch in range(2): # Run for two epochs while True: batch = iterator.next() # Get the next batch # Unpacking the batch manually x_batch = np.array([example[0] for example in batch]) # Extract x data y_batch = np.array([example[1] for example in batch]) # Extract y data print("X batch:", x_batch) print("Y batch:", y_batch) if iterator.is_new_epoch: # Check if a new epoch has started print("End of epoch") break # Reset the iterator to the beginning of the dataset (optional) iterator.reset()
以下是 Chainer 中使用的 SerialIterator 的输出:
X batch: [[0.00603645 0.13716008] [0.97394305 0.9035589 ] [0.93046355 0.63140464] [0.44332692 0.5307854 ] [0.48565307 0.845648 ] [0.98147005 0.47466147] [0.3036461 0.62494874] [0.31664708 0.7176309 ] [0.14955625 0.65800977] [0.72328717 0.33383074]] Y batch: [1 0 0 1 0 0 1 1 1 0] ---------------------------- ---------------------------- ---------------------------- X batch: [[0.10038178 0.32700586] [0.4653218 0.11713986] [0.10589143 0.5662842 ] [0.9196327 0.08948212] [0.13177629 0.59920484] [0.46034923 0.8698121 ] [0.24727622 0.8066094 ] [0.01744546 0.88371164] [0.18966147 0.9189765 ] [0.06658458 0.02469426]] Y batch: [0 1 0 0 0 0 0 0 0 1] End of epoch
MultiprocessIterator
此迭代器旨在通过使用多个进程来加快数据加载速度。在处理大型数据集或数据预处理耗时的情况下,它特别有用。
以下是如何在 Chainer 框架中使用多进程迭代器的示例:
import chainer import numpy as np from chainer import datasets, iterators # Create a simple dataset (e.g., dummy data) x_data = np.random.rand(1000, 2).astype(np.float32) # 1000 samples, 2 features each y_data = np.random.randint(0, 2, size=(1000,)).astype(np.int32) # 1000 binary labels # Combine the features and labels into a Chainer dataset dataset = datasets.TupleDataset(x_data, y_data) # Initialize the MultiprocessIterator # n_processes: Number of worker processes to use iterator = iterators.MultiprocessIterator(dataset, batch_size=32, n_processes=4, repeat=True, shuffle=True) # Example of iterating over the dataset for epoch in range(2): # Run for two epochs while True: batch = iterator.next() # Get the next batch # Unpacking the batch manually x_batch = np.array([example[0] for example in batch]) # Extract x data y_batch = np.array([example[1] for example in batch]) # Extract y data print("X batch shape:", x_batch.shape) print("Y batch shape:", y_batch.shape) if iterator.is_new_epoch: # Check if a new epoch has started print("End of epoch") break # Reset the iterator to the beginning of the dataset (optional) iterator.reset()
以下是多进程迭代器的输出:
X batch shape: (32, 2) Y batch shape: (32,) X batch shape: (32, 2) Y batch shape: (32,) X batch shape: (32, 2) Y batch shape: (32,) --------------------- --------------------- X batch shape: (32, 2) Y batch shape: (32,) X batch shape: (32, 2) Y batch shape: (32,) End of epoch
MultithreadIterator
MultithreadIterator 是 Chainer 中的一个迭代器,旨在使用多个线程进行并行数据加载。在处理可以从并发数据处理中受益的数据集时,此迭代器特别有用,例如当数据加载或预处理是训练中的瓶颈时。
与使用多个进程的MultiprocessIterator不同,MultithreadIterator使用线程,使其更适合需要共享内存访问或轻量级并行的场景。
以下是Chainer框架中使用MultithreadIterator的示例:
import numpy as np from chainer.datasets import TupleDataset from chainer.iterators import MultithreadIterator # Create sample datasets data1 = np.random.rand(100, 3) # 100 samples, 3 features each data2 = np.random.rand(100, 5) # 100 samples, 5 features each # Create a TupleDataset dataset = TupleDataset(data1, data2) # Create a MultithreadIterator with 4 threads and a batch size of 10 iterator = MultithreadIterator(dataset, batch_size=10, n_threads=4, repeat=False, shuffle=True) # Iterate over the dataset for batch in iterator: # Unpack each tuple in the batch data_batch_1 = np.array([item[0] for item in batch]) # Extract the first element from each tuple data_batch_2 = np.array([item[1] for item in batch]) # Extract the second element from each tuple print("Data batch 1:", data_batch_1) print("Data batch 2:", data_batch_2)
以下是Multithread Iterator的输出:
Data batch 1: [[0.38723876 0.66585393 0.74603754] [0.136392 0.23425485 0.6053701 ] [0.99668734 0.13096871 0.13114792] [0.32277508 0.3718192 0.42083016] [0.93408236 0.59433832 0.23590596] [0.16351005 0.82340571 0.08372471] [0.78469682 0.81117013 0.41653794] [0.32369538 0.77524528 0.10378537] [0.21678887 0.8905319 0.88525376] [0.41348068 0.43437296 0.90430938]] --------------------- --------------------- Data batch 2: [[0.20541319 0.69626397 0.81508325 0.49767042 0.92252953] [0.12794664 0.33955336 0.81339754 0.54042266 0.44137714] [0.52487615 0.59930116 0.96334436 0.61622956 0.34192033] [0.93474439 0.37455884 0.94954379 0.73027705 0.24333167] [0.24805745 0.80921792 0.91316062 0.59701139 0.25295744] [0.27026875 0.67836862 0.16911597 0.50452568 0.86257208] [0.81722752 0.41361153 0.43188091 0.98313524 0.28605503] [0.50885091 0.80546812 0.89346966 0.63828489 0.8231125 ] [0.78996715 0.05338346 0.16573956 0.89421364 0.54267903] [0.05804313 0.5613496 0.09146587 0.79961318 0.02466306]]
ShuffleOrderSampler
ShuffleOrderSampler是Chainer中的一个组件,用于随机化数据集中索引的顺序。它确保每个训练时期的数据顺序不同,这有助于减少过拟合并提高模型的泛化能力。
import numpy as np from chainer.datasets import TupleDataset from chainer.iterators import SerialIterator, ShuffleOrderSampler # Create sample datasets data = np.random.rand(100, 3) # 100 samples, 3 features each labels = np.random.randint(0, 2, size=100) # 100 binary labels # Create a TupleDataset dataset = TupleDataset(data, labels) # Initialize ShuffleOrderSampler sampler = ShuffleOrderSampler() # Create a SerialIterator with the ShuffleOrderSampler iterator = SerialIterator(dataset, batch_size=10, repeat=False, order_sampler=sampler) # Iterate over the dataset for batch in iterator: # Since the batch contains tuples, we extract data and labels separately data_batch, label_batch = zip(*batch) print("Data batch:", np.array(data_batch)) print("Label batch:", np.array(label_batch))
以下是Chainer中应用ShuffleOrderSampler迭代器的输出:
Data batch: [[0.93062607 0.68334939 0.73764239] [0.87416648 0.50679946 0.17060853] [0.19647824 0.2195698 0.5010152 ] [0.28589369 0.08394862 0.28748563] [0.55498598 0.73032299 0.01946458] [0.68907645 0.8920713 0.7224627 ] [0.36771187 0.91855943 0.87878009] [0.14039665 0.88076789 0.76606626] [0.84889666 0.57975573 0.70021538] [0.45484641 0.17291856 0.42353947]] Label batch: [0 1 1 0 1 0 1 1 0 0] ------------------------------------- ------------------------------------- Data batch: [[0.0692231 0.24701816 0.24603659] [0.72014948 0.67211487 0.45648504] [0.8625562 0.45570299 0.58156546] [0.60350332 0.81757841 0.30411054] [0.93224841 0.3055118 0.07809648] [0.16425884 0.69060297 0.36452719] [0.79252781 0.35895253 0.26741555] [0.27568602 0.38510119 0.36718876] [0.58806512 0.35221788 0.08439596] [0.13015496 0.81817428 0.86631724]] Label batch: [0 0 1 0 1 0 1 0 0 1]
训练循环
训练循环是机器学习的核心机制,模型通过它从数据中学习。它包含一个重复的过程:将数据输入模型,计算误差(损失),调整模型参数以减少误差,然后重复此过程,直到模型在任务上的表现足够好。训练循环是训练神经网络和其他机器学习模型的基础。
训练循环中的关键组件
- 模型: 你想要训练的神经网络或机器学习模型。
- 损失函数: 用于衡量模型预测与实际数据匹配程度的函数,例如均方误差、交叉熵。
- 优化器: 用于根据计算的梯度更新模型参数的算法,例如SGD、Adam。
- 数据: 用于训练的数据集,通常被划分为小批量(minibatches)以提高处理效率。
为什么训练循环很重要?
训练循环在深度学习和机器学习中至关重要,原因如下:
- 效率: 它们允许模型通过处理小批量数据(即minibatches)来训练大型数据集。
- 迭代改进: 通过反复调整模型参数,训练循环使模型能够学习并随着时间的推移提高其准确性。
- 灵活性: 训练循环可以自定义,包括学习率调度、提前停止或监控指标等附加功能。
训练循环的关键步骤
训练循环的步骤如下:
- 前向传播: 首先将输入数据馈入模型,然后模型通过其各层处理数据以产生输出(预测)。
- 损失计算: 使用损失函数将输出与实际目标值进行比较。损失函数计算预测输出与实际目标之间的误差或差异。
- 反向传播: 计算损失相对于模型每个参数(权重)的梯度。这些梯度指示每个参数对误差的贡献程度。
- 参数更新: 使用优化算法(例如SGD、Adam等)更新模型参数。参数以最小化损失的方式进行调整。
- 重复: 此过程重复多次迭代(时期),模型多次查看数据。目标是让模型通过逐渐减少损失来学习并改进其预测。
示例
在Chainer中,训练循环用于迭代数据集,计算损失并更新模型参数。以下是一个使用Chainer演示基本训练循环的示例。此示例使用在MNIST数据集上训练的简单前馈神经网络。
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain, optimizers, training, serializers from chainer.datasets import TupleDataset from chainer.iterators import SerialIterator from chainer.training import extensions import numpy as np # Define the neural network model class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.l1 = L.Linear(3, 5) # Input layer to hidden layer self.l2 = L.Linear(5, 2) # Hidden layer to output layer def forward(self, x): h = F.relu(self.l1(x)) # Apply ReLU activation y = self.l2(h) # Output layer return y def __call__(self, x, t): y = self.forward(x) return F.softmax_cross_entropy(y, t) # Generate synthetic data data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]], dtype=np.float32) labels = np.array([0, 1, 0], dtype=np.int32) # Create a dataset and iterator dataset = TupleDataset(data, labels) iterator = SerialIterator(dataset, batch_size=1, shuffle=True) # Initialize the model, optimizer, and updater model = SimpleNN() optimizer = optimizers.Adam() optimizer.setup(model) # Set up the trainer updater = training.StandardUpdater(iterator, optimizer, device=-1) trainer = training.Trainer(updater, (10, 'epoch'), out='result') # Add extensions to monitor training trainer.extend(extensions.Evaluator(iterator, model, device=-1)) trainer.extend(extensions.LogReport()) trainer.extend(extensions.PrintReport(['epoch', 'main/loss', 'validation/main/loss'])) trainer.extend(extensions.ProgressBar()) # Start training trainer.run()
以下是训练循环的输出:
epoch main/loss validation/main/loss