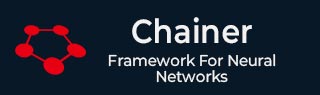
Chainer - 创建神经网络
使用 Chainer **创建神经网络**是一个灵活且直观的流程,它采用 **运行时定义** 的方法。这允许开发者在数据流经网络时动态构建和修改计算图。Chainer 支持各种神经网络架构,从简单的前馈网络到更复杂的结构,如循环神经网络或卷积神经网络。
通过启用动态图构建,Chainer 使得更容易实验不同的网络设计,调试问题并实现针对特定任务定制的高级模型。这种灵活性在研究和开发中特别有价值,因为快速原型设计和迭代至关重要。
在 Chainer 中创建神经网络的步骤
让我们详细了解如何使用 Chainer 构建、训练和测试一个简单的前馈神经网络。这些步骤突出了 Chainer 运行时定义方法的灵活性和简单性,通过简化对不同网络架构和训练方法的实验。
安装 Chainer
在开始使用 Chainer 创建神经网络之前,我们应该确保 **Chainer** 已安装在我们的工作环境中。我们可以使用以下代码通过 pip 安装它:
pip install Chainer
导入所需的库
在我们的工作环境中安装 Chainer 后,我们需要从 Chainer 中导入所有必要的组件,例如 Chain、Variable、优化器以及激活函数和损失计算的函数。
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain, optimizers, Variable import numpy as np
定义神经网络
在此步骤中,我们将定义一个具有两个隐藏层的神经网络。每个层都将使用 ReLU 激活函数,输出层将使用 sigmoid 函数,因为这是一个二元分类任务。
以下是定义神经网络的代码:
class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.l1 = L.Linear(None, 10) # Input to hidden layer 1 self.l2 = L.Linear(10, 10) # Hidden layer 1 to hidden layer 2 self.l3 = L.Linear(10, 1) # Hidden layer 2 to output layer def forward(self, x): h1 = F.relu(self.l1(x)) h2 = F.relu(self.l2(h1)) y = F.sigmoid(self.l3(h2)) # Sigmoid activation for binary classification return y
创建模型和优化器
接下来,我们必须初始化模型并选择一个优化器。在这里,我们使用 **Adam 优化器**。以下是代码:
# Instantiate the model and optimizer model = SimpleNN() optimizer = optimizers.Adam() optimizer.setup(model)
准备数据
为了更好地理解,我们将创建一些虚拟数据。通常情况下,我们会在此时加载我们的数据集。
# Generate example data X_train = np.random.rand(100, 5).astype(np.float32) # 100 samples, 5 features y_train = np.random.randint(0, 2, size=(100, 1)).astype(np.int32) # 100 binary labels (integers)
训练网络
在此步骤中,我们在 Chainer 中执行手动训练循环,展示了如何通过以小批量迭代数据集来训练神经网络,执行前向传递以进行预测,计算损失,然后使用反向传播更新模型的权重。循环运行指定数量的 epoch,并打印每个 epoch 的损失以跟踪模型的训练进度。
我们将使用一个简单的循环来训练网络。对于每个 epoch,我们将执行以下步骤:
- 前向传递
- 计算损失
- 反向传播(梯度计算)
- 更新权重
以下是执行 Chainer 中简单手动训练循环的代码:
n_epochs = 10 batch_size = 10 for epoch in range(n_epochs): for i in range(0, len(X_train), batch_size): x_batch = Variable(X_train[i:i+batch_size]) y_batch = Variable(y_train[i:i+batch_size]) # Forward pass y_pred = model.forward(x_batch) # Debugging: Print shapes and types print(f"x_batch shape: {x_batch.shape}, type: {x_batch.dtype}") print(f"y_batch shape: {y_batch.shape}, type: {y_batch.dtype}") print(f"y_pred shape: {y_pred.shape}, type: {y_pred.dtype}") # Ensure y_pred and y_batch have the same shape if y_pred.shape != y_batch.shape: y_pred = F.reshape(y_pred, y_batch.shape) # Compute loss loss = F.sigmoid_cross_entropy(y_pred, y_batch) # Backward pass and weight update model.cleargrads() loss.backward() optimizer.update() print(f'Epoch {epoch+1}, Loss: {loss.array}')
测试模型
训练后,我们需要在新的数据上测试模型。以下是我们在 Chainer 中进行测试的方法。以下是代码:
# Test the model X_test = np.random.rand(10, 5).astype(np.float32) # 10 samples, 5 features y_test = model.forward(Variable(X_test)) print("Predictions:", y_test.data)
保存和加载模型
我们可以将训练好的模型保存到文件中,并在以后加载以进行推理,如下所示:
# Save the model chainer.serializers.save_npz('simple_nn.model', model) # Load the model chainer.serializers.load_npz('simple_nn.model', model)
现在让我们将上面提到的所有步骤组合成一个,并查看在 Chainer 中创建的神经网络的结果:
import chainer import chainer.functions as F import chainer.links as L from chainer import Chain, optimizers, Variable import numpy as np class SimpleNN(Chain): def __init__(self): super(SimpleNN, self).__init__() with self.init_scope(): self.l1 = L.Linear(None, 10) # Input to hidden layer 1 self.l2 = L.Linear(10, 10) # Hidden layer 1 to hidden layer 2 self.l3 = L.Linear(10, 1) # Hidden layer 2 to output layer def forward(self, x): h1 = F.relu(self.l1(x)) h2 = F.relu(self.l2(h1)) y = F.sigmoid(self.l3(h2)) # Sigmoid activation for binary classification return y # Instantiate the model and optimizer model = SimpleNN() optimizer = optimizers.Adam() optimizer.setup(model) # Generate example data X_train = np.random.rand(100, 5).astype(np.float32) # 100 samples, 5 features y_train = np.random.randint(0, 2, size=(100, 1)).astype(np.int32) # 100 binary labels (integers) n_epochs = 10 batch_size = 10 for epoch in range(n_epochs): for i in range(0, len(X_train), batch_size): x_batch = Variable(X_train[i:i+batch_size]) y_batch = Variable(y_train[i:i+batch_size]) # Forward pass y_pred = model.forward(x_batch) # Debugging: Print shapes and types print(f"x_batch shape: {x_batch.shape}, type: {x_batch.dtype}") print(f"y_batch shape: {y_batch.shape}, type: {y_batch.dtype}") print(f"y_pred shape: {y_pred.shape}, type: {y_pred.dtype}") # Ensure y_pred and y_batch have the same shape if y_pred.shape != y_batch.shape: y_pred = F.reshape(y_pred, y_batch.shape) # Compute loss loss = F.sigmoid_cross_entropy(y_pred, y_batch) # Backward pass and weight update model.cleargrads() loss.backward() optimizer.update() print(f'Epoch {epoch+1}, Loss: {loss.array}') # Test the model X_test = np.random.rand(10, 5).astype(np.float32) # 10 samples, 5 features y_test = model.forward(Variable(X_test)) print("Predictions:", y_test.data) # Save the model chainer.serializers.save_npz('simple_nn.model', model) # Load the model chainer.serializers.load_npz('simple_nn.model', model)
以下是使用 Chainer 框架创建的简单神经网络的输出:
x_batch shape: (10, 5), type: float32 y_batch shape: (10, 1), type: int32 y_pred shape: (10, 1), type: float32 x_batch shape: (10, 5), type: float32 y_batch shape: (10, 1), type: int32 y_pred shape: (10, 1), type: float32 x_batch shape: (10, 5), type: float32 y_batch shape: (10, 1), type: int32 y_pred shape: (10, 1), type: float32 x_batch shape: (10, 5), type: float32 y_batch shape: (10, 1), type: int32 ------------------------------------ ------------------------------------ ------------------------------------ y_pred shape: (10, 1), type: float32 Epoch 10, Loss: 0.6381329298019409 Predictions: [[0.380848 ] [0.40808532] [0.35226226] [0.42560062] [0.3757095 ] [0.35753834] [0.38465175] [0.35967904] [0.37653774] [0.4149222 ]]
在本章中,我们演示了使用 Chainer 创建和训练神经网络的基本工作流程。我们可以实验不同的架构、优化器和超参数,以了解它们如何影响性能。